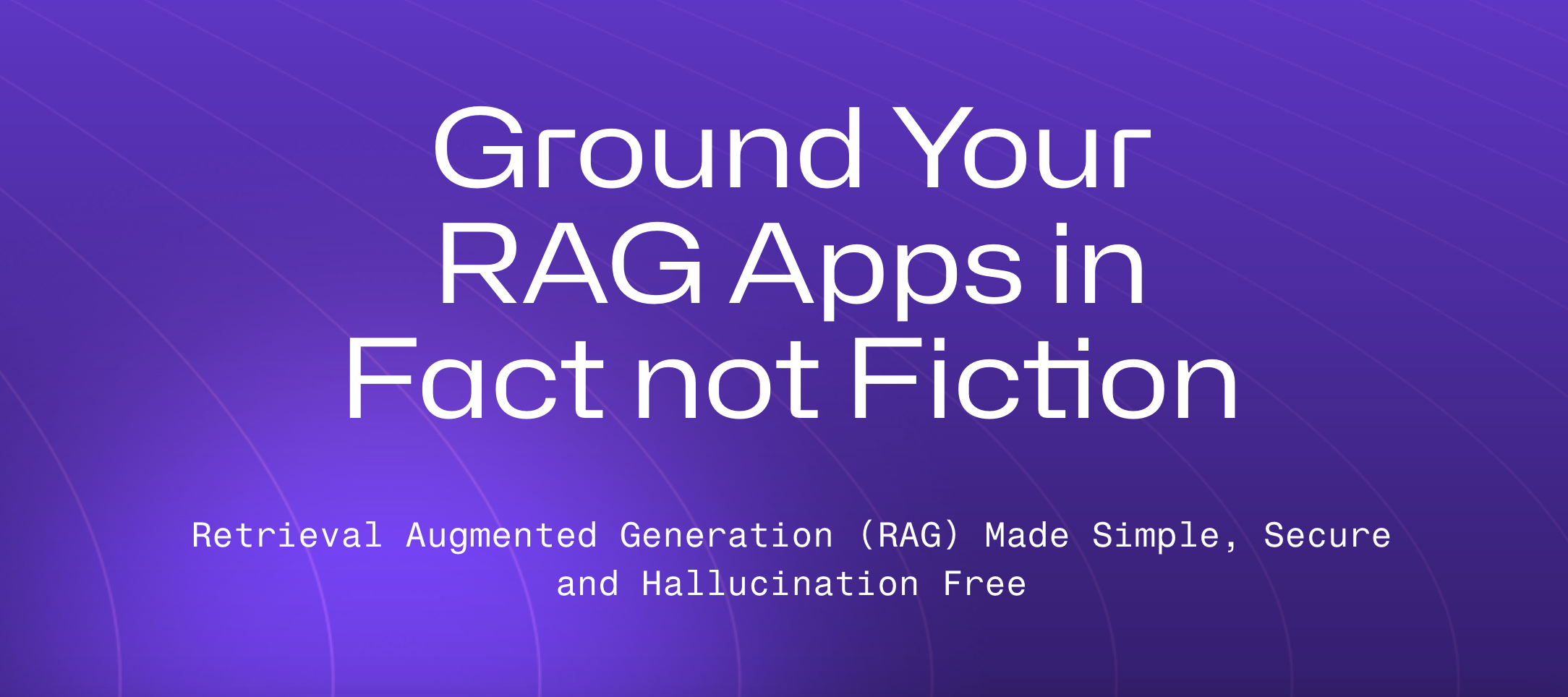
EyeLevel's GroundX APIs
RAG Made Simple, Secure and Hallucination Free

Table of Contents
Requirements
Python >=3.7
Installation
pip install groundx-python-sdk==1.3.29
Getting Started
from pprint import pprint
from groundx import Groundx, ApiException
groundx = Groundx(
api_key="YOUR_API_KEY",
)
try:
create_response = groundx.buckets.create(
name="your_bucket_name",
)
pprint(create_response.body)
pprint(create_response.body["bucket"])
pprint(create_response.headers)
pprint(create_response.status)
pprint(create_response.round_trip_time)
except ApiException as e:
print("Exception when calling BucketsApi.create: %s\n" % e)
pprint(e.body)
pprint(e.headers)
pprint(e.status)
pprint(e.reason)
pprint(e.round_trip_time)
Async
async
support is available by prepending a
to any method.
import asyncio
from pprint import pprint
from groundx import Groundx, ApiException
groundx = Groundx(
api_key="YOUR_API_KEY",
)
async def main():
try:
create_response = await groundx.buckets.acreate(
name="your_bucket_name",
)
pprint(create_response.body)
pprint(create_response.body["bucket"])
pprint(create_response.headers)
pprint(create_response.status)
pprint(create_response.round_trip_time)
except ApiException as e:
print("Exception when calling BucketsApi.create: %s\n" % e)
pprint(e.body)
pprint(e.headers)
pprint(e.status)
pprint(e.reason)
pprint(e.round_trip_time)
asyncio.run(main())
Reference
groundx.buckets.create
Create a new bucket.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
create_response = groundx.buckets.create(
name="your_bucket_name",
)
⚙️ Parameters
name: str
⚙️ Request Body
BucketCreateRequest
🔄 Return
BucketResponse
🌐 Endpoint
/v1/bucket
post
🔙 Back to Table of Contents
groundx.buckets.delete
Delete a bucket.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
delete_response = groundx.buckets.delete(
bucket_id=1,
)
⚙️ Parameters
bucket_id: int
The bucketId of the bucket being deleted.
🔄 Return
MessageResponse
🌐 Endpoint
/v1/bucket/{bucketId}
delete
🔙 Back to Table of Contents
groundx.buckets.get
Look up a specific bucket by its bucketId.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
get_response = groundx.buckets.get(
bucket_id=1,
)
⚙️ Parameters
bucket_id: int
The bucketId of the bucket to look up.
🔄 Return
BucketResponse
🌐 Endpoint
/v1/bucket/{bucketId}
get
🔙 Back to Table of Contents
groundx.buckets.list
List all buckets within your GroundX account
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
list_response = groundx.buckets.list(
n=1,
next_token="string_example",
)
⚙️ Parameters
n: int
The maximum number of returned buckets. Accepts 1-100 with a default of 20.
next_token: str
A token for pagination. If the number of buckets for a given query is larger than n, the response will include a "nextToken" value. That token can be included in this field to retrieve the next batch of n buckets.
🔄 Return
BucketListResponse
🌐 Endpoint
/v1/bucket
get
🔙 Back to Table of Contents
groundx.buckets.update
Rename a bucket.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
update_response = groundx.buckets.update(
new_name="your_bucket_name",
bucket_id=1,
)
⚙️ Parameters
new_name: str
The new name of the bucket being renamed.
bucket_id: int
The bucketId of the bucket being updated.
⚙️ Request Body
BucketUpdateRequest
🔄 Return
BucketUpdateResponse
🌐 Endpoint
/v1/bucket/{bucketId}
put
🔙 Back to Table of Contents
groundx.customer.get
Get the account information associated with the API key.
🛠️ Usage
get_response = groundx.customer.get()
🔄 Return
CustomerResponse
🌐 Endpoint
/v1/customer
get
🔙 Back to Table of Contents
groundx.documents.crawl_website
Upload the content of a publicly accessible website for ingestion into a GroundX bucket. This is done by following links within a specified URL, recursively, up to a specified depth or number of pages.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
crawl_website_response = groundx.documents.crawl_website(
websites=[
{
"bucket_id": 123,
"cap": 100,
"depth": 3,
"source_url": "https://my.website.com",
}
],
)
⚙️ Parameters
⚙️ Request Body
WebsiteCrawlRequest
🔄 Return
IngestResponse
🌐 Endpoint
/v1/ingest/documents/website
post
🔙 Back to Table of Contents
groundx.documents.delete
Delete multiple documents hosted on GroundX
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
delete_response = groundx.documents.delete(
document_ids=["documentIds_example"],
)
⚙️ Parameters
document_ids: List[str
]
A list of documentIds which correspond to documents ingested by GroundX
🔄 Return
IngestResponse
🌐 Endpoint
/v1/ingest/documents
delete
🔙 Back to Table of Contents
groundx.documents.delete1
Delete a single document hosted on GroundX
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
delete1_response = groundx.documents.delete1(
document_id="documentId_example",
)
⚙️ Parameters
document_id: str
A documentId which correspond to a document ingested by GroundX
🔄 Return
IngestResponse
🌐 Endpoint
/v1/ingest/document/{documentId}
delete
🔙 Back to Table of Contents
groundx.documents.get
Look up an existing document by documentId.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
get_response = groundx.documents.get(
document_id="documentId_example",
)
⚙️ Parameters
document_id: str
The documentId of the document for which GroundX information will be provided.
🔄 Return
DocumentResponse
🌐 Endpoint
/v1/ingest/document/{documentId}
get
🔙 Back to Table of Contents
groundx.documents.get_processing_status_by_id
Get the current status of an ingest, initiated with documents.ingest_remote, documents.ingest_local, or documents.crawl_website, by specifying the processId (the processId is included in the response of the documents.ingest functions).
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
get_processing_status_by_id_response = groundx.documents.get_processing_status_by_id(
process_id="processId_example",
)
⚙️ Parameters
process_id: str
the processId for the ingest process being checked
🔄 Return
ProcessStatusResponse
🌐 Endpoint
/v1/ingest/{processId}
get
🔙 Back to Table of Contents
groundx.documents.ingest_local
Upload documents hosted on a local file system for ingestion into a GroundX bucket.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
ingest_local_response = groundx.documents.ingest_local(
body=[
{
"blob": open("/path/to/file", "rb"),
"metadata": {
"bucket_id": 1234,
"file_name": "my_file.txt",
"file_type": "txt",
},
}
],
)
⚙️ Request Body
DocumentLocalIngestRequest
🔄 Return
IngestResponse
🌐 Endpoint
/v1/ingest/documents/local
post
🔙 Back to Table of Contents
groundx.documents.ingest_remote
Ingest documents hosted on public URLs to a GroundX bucket.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
ingest_remote_response = groundx.documents.ingest_remote(
documents=[
{
"bucket_id": 1234,
"file_name": "my_file.txt",
"file_type": "txt",
"source_url": "https://my.source.url.com/file.txt",
}
],
)
⚙️ Parameters
⚙️ Request Body
DocumentRemoteIngestRequest
🔄 Return
IngestResponse
🌐 Endpoint
/v1/ingest/documents/remote
post
🔙 Back to Table of Contents
groundx.documents.list
lookup all documents across all resources which are currently on GroundX
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
list_response = groundx.documents.list(
n=1,
filter="string_example",
sort="name",
sort_order="asc",
status="queued",
next_token="string_example",
)
⚙️ Parameters
n: int
The maximum number of returned documents. Accepts 1-100 with a default of 20.
filter: str
Only documents with names that contain the filter string will be returned in the results.
The document attribute that will be used to sort the results.
The order in which to sort the results. A value for sort must also be set.
A status filter on the get documents query. If this value is set, then only documents with this status will be returned in the results.
next_token: str
A token for pagination. If the number of documents for a given query is larger than n, the response will include a "nextToken" value. That token can be included in this field to retrieve the next batch of n documents.
🔄 Return
DocumentListResponse
🌐 Endpoint
/v1/ingest/documents
get
🔙 Back to Table of Contents
groundx.documents.lookup
lookup the document(s) associated with a processId, bucketId, groupId, or projectId.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
lookup_response = groundx.documents.lookup(
id=1,
n=1,
filter="string_example",
sort="name",
sort_order="asc",
status="queued",
next_token="string_example",
)
⚙️ Parameters
id: int
a processId, bucketId, groupId, or projectId
n: int
The maximum number of returned documents. Accepts 1-100 with a default of 20.
filter: str
Only documents with names that contain the filter string will be returned in the results.
The document attribute that will be used to sort the results.
The order in which to sort the results. A value for sort must also be set.
A status filter on the get documents query. If this value is set, then only documents with this status will be returned in the results.
next_token: str
A token for pagination. If the number of documents for a given query is larger than n, the response will include a "nextToken" value. That token can be included in this field to retrieve the next batch of n documents.
🔄 Return
DocumentLookupResponse
🌐 Endpoint
/v1/ingest/documents/{id}
get
🔙 Back to Table of Contents
groundx.groups.add_bucket
Add an existing bucket to an existing group. Buckets and groups can be associated many to many.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
add_bucket_response = groundx.groups.add_bucket(
group_id=1,
bucket_id=1,
)
⚙️ Parameters
group_id: int
The groupId of the group which the bucket will be added to.
bucket_id: int
The bucketId of the bucket being added to the group.
🔄 Return
MessageResponse
🌐 Endpoint
/v1/group/{groupId}/bucket/{bucketId}
post
🔙 Back to Table of Contents
groundx.groups.create
create a new group, a group being a collection of buckets which can be searched.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
create_response = groundx.groups.create(
name="your_group_name",
bucket_name="your_new_bucket_name",
)
⚙️ Parameters
name: str
The name of the group being created.
bucket_name: str
Specify bucketName to automatically create a bucket, by the name specified, and add it to the created group.
⚙️ Request Body
GroupCreateRequest
🔄 Return
GroupResponse
🌐 Endpoint
/v1/group
post
🔙 Back to Table of Contents
groundx.groups.delete
Delete a group.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
delete_response = groundx.groups.delete(
group_id=1,
)
⚙️ Parameters
group_id: int
The groupId of the group to be deleted.
🔄 Return
MessageResponse
🌐 Endpoint
/v1/group/{groupId}
delete
🔙 Back to Table of Contents
groundx.groups.get
look up a specific group by its groupId.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
get_response = groundx.groups.get(
group_id=1,
)
⚙️ Parameters
group_id: int
The groupId of the group to look up.
🔄 Return
GroupResponse
🌐 Endpoint
/v1/group/{groupId}
get
🔙 Back to Table of Contents
groundx.groups.list
list all groups within your GroundX account.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
list_response = groundx.groups.list(
n=1,
next_token="string_example",
)
⚙️ Parameters
n: int
The maximum number of returned groups. Accepts 1-100 with a default of 20.
next_token: str
A token for pagination. If the number of groups for a given query is larger than n, the response will include a "nextToken" value. That token can be included in this field to retrieve the next batch of n groups.
🔄 Return
GroupListResponse
🌐 Endpoint
/v1/group
get
🔙 Back to Table of Contents
groundx.groups.remove_bucket
remove a bucket from a group. Buckets and groups can be associated many to many, this removes one bucket to group association without disturbing others.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
remove_bucket_response = groundx.groups.remove_bucket(
group_id=1,
bucket_id=1,
)
⚙️ Parameters
group_id: int
The groupId of the group which the bucket will be removed from.
bucket_id: int
The bucketId of the bucket which will be removed from the group.
🔄 Return
MessageResponse
🌐 Endpoint
/v1/group/{groupId}/bucket/{bucketId}
delete
🔙 Back to Table of Contents
groundx.groups.update
Rename a group
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
update_response = groundx.groups.update(
new_name="your_group_name",
group_id=1,
)
⚙️ Parameters
new_name: str
The new name of the group being renamed.
group_id: int
The groupId of the group to update.
⚙️ Request Body
GroupUpdateRequest
🔄 Return
GroupResponse
🌐 Endpoint
/v1/group/{groupId}
put
🔙 Back to Table of Contents
groundx.health.get
Look up the current health status of a specific service. Statuses update every 5 minutes.
🛠️ Usage
get_response = groundx.health.get(
service="search",
)
⚙️ Parameters
service: str
The name of the service to look up.
🔄 Return
HealthResponse
🌐 Endpoint
/v1/health/{service}
get
🔙 Back to Table of Contents
groundx.health.list
List the current health status of all services. Statuses update every 5 minutes.
🛠️ Usage
list_response = groundx.health.list()
🔄 Return
HealthResponse
🌐 Endpoint
/v1/health
get
🔙 Back to Table of Contents
groundx.search.content
Search documents on GroundX for the most relevant information to a given query.
The result of this query is typically used in one of two ways; result['search']['text'] can be used to provide context to a language model, facilitating RAG, or result['search']['results'] can be used to observe chunks of text which are relevant to the query, facilitating citation.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
content_response = groundx.search.content(
query="my search query",
id=None,
relevance=10,
n=20,
next_token="eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9",
verbosity=0,
)
⚙️ Parameters
query: str
The search query to be used to find relevant documentation.
id: Union[int
, str
]
The bucketId, groupId, projectId, or documentId to be searched. The document or documents within the specified container will be compared to the query, and relevant information will be extracted.
relevance: Union[int, float]
The minimum search relevance score required to include the result. By default, this is 10.0.
n: int
The maximum number of returned search results. Accepts 1-100 with a default of 20.
next_token: str
A token for pagination. If the number of search results for a given query is larger than n, the response will include a "nextToken" value. That token can be included in this field to retrieve the next batch of n search results.
verbosity: int
The amount of data returned with each search result. 0 == no search results, only the recommended context. 1 == search results but no searchData. 2 == search results and searchData.
⚙️ Request Body
SearchRequest
🔄 Return
SearchResponse
🌐 Endpoint
/v1/search/{id}
post
🔙 Back to Table of Contents
groundx.search.documents
Search documents on GroundX for the most relevant information to a given query by documentId(s).
The result of this query is typically used in one of two ways; result['search']['text'] can be used to provide context to a language model, facilitating RAG, or result['search']['results'] can be used to observe chunks of text which are relevant to the query, facilitating citation.
Interact with the "Request Body" below to explore the arguments of this function. Enter your GroundX API key to send a request directly from this web page. Select your language of choice to structure a code snippet based on your specified arguments.
🛠️ Usage
documents_response = groundx.search.documents(
query="my search query",
document_ids=["docUUID1", "docUUID2"],
relevance=10,
n=20,
next_token="eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9",
verbosity=0,
)
⚙️ Parameters
query: str
The search query to be used to find relevant documentation.
relevance: Union[int, float]
The minimum search relevance score required to include the result. By default, this is 10.0.
n: int
The maximum number of returned search results. Accepts 1-100 with a default of 20.
next_token: str
A token for pagination. If the number of search results for a given query is larger than n, the response will include a "nextToken" value. That token can be included in this field to retrieve the next batch of n search results.
verbosity: int
The amount of data returned with each search result. 0 == no search results, only the recommended context. 1 == search results but no searchData. 2 == search results and searchData.
⚙️ Request Body
SearchDocumentsRequest
🔄 Return
SearchResponse
🌐 Endpoint
/v1/search/documents
post
🔙 Back to Table of Contents
Author
This Python package is automatically generated by Konfig