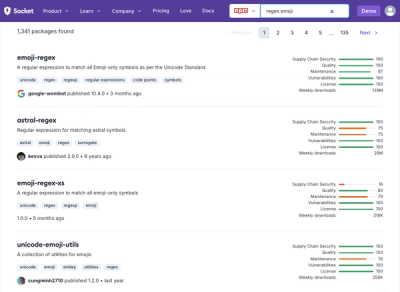
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
The Ola Maps Python library provides convenient access to the Ola Maps API from any Python 3.9+ application. The library currently includes type definitions for Routing APIs, Roads APIs, Places APIs, Geocoding and Maps Tiles details can be found here.
# install from PyPI
pip install py_olamaps
Follow the official documentation of Ola Maps to generate the API Key.
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
# OR
client = OlaMaps(client_id=os.environ.get("OLA_MAPS_CLIENT_ID"),
client_secret=os.environ.get("OLA_MAPS_CLIENT_SECRET"))
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
routing_direction = client.routing.directions(
"12.993103152916301,77.54332622119354",
"12.972006793201695,77.5800850011884"
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
distance_matrix = client.routing.distance_matrix(
"28.71866756826579,77.03699668376802|28.638555357785652,76.96550156007675",
"28.638555357785652,76.96550156007675|28.53966907108812,77.05190669909288"
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
snap_to_Road = client.roads.snap_to_road(
"12.99927894246456,77.67323803525812|12.992086564113583,77.65899014102202|12.992567456375086,77.65989136324778|12.992672238708593,77.64337109685341|12.99127113597667,77.65716623889841",
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
nearest_roads = client.roads.nearest_roads(
"12.99927894246456,77.67323803525812|12.992086564113583,77.65899014102202|12.992567456375086,77.65989136324778",
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
autocomplete = client.places.autocomplete(
"kempe"
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
place_details = client.places.place_details(
"ola-platform:a79ed32419962a11a588ea92b83ca78e"
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
nearby_search = client.places.nearby_search(
"venue",
"12.931544865377818,77.61638622280486"
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
text_search = client.places.text_search(
"Cafes in Koramangala"
)
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
forward_geocode = client.geocode.forward_geocode("Mumbai")
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
reverse_geocode = client.geocode.reverse_geocode("12.931316595874005,77.61649243443775")
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
array_of_data = client.map_tiles.array_of_data("planet")
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
styles = client.map_tiles.get_map_style()
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
detail_of_style = client.map_tiles.get_style_details("default-light-standard")
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
# note - the below returns an object as it contains an image
smi_center_point = client.map_tiles.static_map_image_based_on_center_point("default-light-standard", 77.61, 12.93, 15,
800, 600, "png")
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
# note - the below returns an object as it contains an image
smi_bounding_box = client.map_tiles.static_map_image_based_on_bounding_box("default-light-standard", 77.611182859373,
12.93219851203095, 77.61513567417848,
12.935739723360513, 800, 600, "png")
import os
from py_olamaps.OlaMaps import OlaMaps
client = OlaMaps(
api_key=os.environ.get("OLA_MAPS_API_KEY"),
)
# note - the below returns an object as it contains an image
static_map_image = client.map_tiles.static_map_image("default-light-standard", 800, 600, "png",
"77.61,12.93|77.61190639293811,12.937637130956137|width:6|stroke:#00ff44")
While you can provide an api_key
keyword argument,
we recommend using python-dotenv
to add OLA_MAPS_API_KEY="My API Key"
or OLA_MAPS_CLIENT_ID="My Client Id"
and OLA_MAPS_CLIENT_SECRET="My Client Secret"
to your .env
file
so that your API Key is not stored in source control.
Error codes are as followed:
Status Code | Error Type |
---|---|
400 | Bad Request |
401 | Unauthorized |
403 | Forbidden |
404 | Not Found |
409 | Conflict |
422 | Unprocessable Entity |
429 | Too Many Requests |
>=500 | InternalServerError |
Coming Soon...
Coming Soon...
This package generally follows SemVer conventions, though certain backwards-incompatible changes may be released as minor versions:
We take backwards-compatibility seriously and work hard to ensure you can rely on a smooth upgrade experience.
We are keen for your feedback; please open an issue with questions, bugs, or suggestions.
Python 3.9 or higher.
FAQs
Python client library for Ola Maps API Services
We found that py-olamaps demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.