☀️ pyopenuv: A simple Python API for data from openuv.io

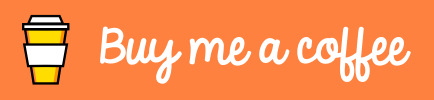
pyopenuv
is a simple Python library for retrieving UV-related information from
openuv.io.
Installation
pip install pyopenuv
Python Versions
pyopenuv
is currently supported on:
- Python 3.10
- Python 3.11
- Python 3.12
API Key
You can get an API key from the OpenUV console.
Usage
import asyncio
from pyopenuv import Client
from pyopenuv.errors import OpenUvError
async def main():
client = Client(
"<OPENUV_API_KEY>", "<LATITUDE>", "<LONGITUDE>", altitude="<ALTITUDE>"
)
try:
print(await client.api_status())
print(await client.uv_index())
print(await client.uv_forecast())
print(await client.uv_protection_window())
print(await client.api_statistics())
except OpenUvError as err:
print(f"There was an error: {err}")
asyncio.run(main())
Checking API Status Before Requests
If you would prefer to not call api_status
manually, you can configure the Client
object
to automatically check the status of the OpenUV API before executing any of the API
methods—simply pass the check_status_before_request
parameter:
import asyncio
from pyopenuv import Client
from pyopenuv.errors import ApiUnavailableError, OpenUvError
async def main():
client = Client(
"<OPENUV_API_KEY>",
"<LATITUDE>",
"<LONGITUDE>",
altitude="<ALTITUDE>",
check_status_before_request=True,
)
try:
print(await client.uv_index())
except ApiUnavailableError:
print("The API is unavailable")
except OpenUvError as err:
print(f"There was an error: {err}")
asyncio.run(main())
Connection Pooling
By default, the library creates a new connection to OpenUV with each coroutine. If you
are calling a large number of coroutines (or merely want to squeeze out every second of
runtime savings possible), an aiohttp
ClientSession
can be used for
connection pooling:
import asyncio
from aiohttp import ClientSession
from pyopenuv import Client
from pyopenuv.errors import OpenUvError
async def main():
async with ClientSession() as session:
client = Client(
"<OPENUV_API_KEY>",
"<LATITUDE>",
"<LONGITUDE>",
altitude="<ALTITUDE>",
session=session,
)
try:
print(await client.uv_index())
except OpenUvError as err:
print(f"There was an error: {err}")
asyncio.run(main())
Contributing
Thanks to all of our contributors so far!
- Check for open features/bugs or initiate a discussion on one.
- Fork the repository.
- (optional, but highly recommended) Create a virtual environment:
python3 -m venv .venv
- (optional, but highly recommended) Enter the virtual environment:
source ./.venv/bin/activate
- Install the dev environment:
script/setup
- Code your new feature or bug fix on a new branch.
- Write tests that cover your new functionality.
- Run tests and ensure 100% code coverage:
poetry run pytest --cov pyopenuv tests
- Update
README.md
with any new documentation. - Submit a pull request!