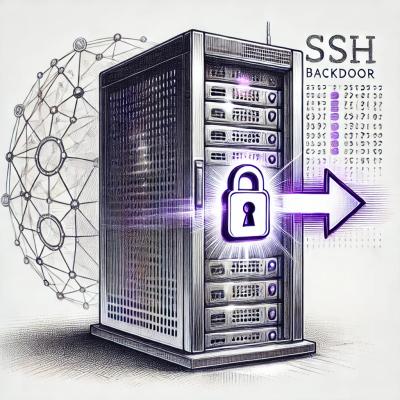
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Pyromenu is an easy-to-extend object-oriented library for building keyboard menus for telegram bots on the Pyrogram
from pyrogram import Client, Filters, ReplyKeyboardRemove
from pyromenu import KButton, KRow, KMenu
app = Client("app")
# it's menu declaration style
menu = KMenu(
KRow(
KButton("English"), KButton("Russian"), KButton("Portugues")
),
KRow(
KButton("exit")
)
)
@app.on_message(Filters.command("start"))
def send_keyboard(clt, msg):
msg.reply("Hi / Привет / Oi", reply_markup=menu.keyboard)
# use KButton update_filter property for creating Filter for Handler's
@app.on_message(KButon("exit").update_filter)
def remove_keyboard(clt, msg):
msg.reply("Bye / Пока / Adeus", reply_markup=ReplyKeyboardRemove)
Pyromenu define 3 abstract classes: Button, Row, Menu
__hash__
__eq__
keyboard_button
(properrty) - return KeyboardButtonupdate_filter
(property) - return Filterkeyboard_row
(property) - return list of KeyboardButton'sbuttons
(property) - return list of Button'skeyboard
(property) - return ReplyKeyboardButtonone_time_keyboard
(property) - same as keyboard
, but with one_time_keyboard flag onfilter
(property) - return composite Filter of all buttonmatched_button
- take message and return first matched buttonPyromenu provides simple built-in implementations of each abstract classes: KButton, KRow, KMenu
Pyromenu has a distinctive menu declaration style inspired by the principles of Elegant Objects.
from pyrogram import Client
from pyromenu import KButton, KRow, KMenu
app = Client("app")
menu = KMenu(
KRow(
KButton("English"), KButton("Russian"), KButton("Portugues")
),
KRow(
KButton("exit")
)
)
app.send_message(chat_id, text, reply_markup=menu.keyboard)
# or
# send one time keyboard
app.send_message(chat_id, text, reply_markup=menu.one_time_keyboard)
@app.on_message(KButton("exit").update_filter)
def exit(clt, msg):
msg.reply("Bye / Пока / Adeus" reply_markup=ReplyKeyboardRemove)
# or
# if you have more complex menu
@app.on_message(menu.filter)
def handle_menu(clt, msg):
matched_button = menu.matched_button(msg)
if matched_button == KButton("exit"):
msg.reply("Bye / Пока / Adeus" reply_markup=ReplyKeyboardRemove)
...
All built-in classes have minimal dependency on others. All you need to do is implement abstract class. For example if you need to create button, which send location request you can create that:
from pyrogram import KeyboardButton, Filters
from pyromenu.abc import Button
class LocationButton(Button):
def __init__(self, text):
self._text = text
def __hash__(self):
return hash(self._text)
def __eq__(self, other_button):
return hash(self) == hash(other_button)
@property
def keyboard_button(self):
return KeyboardButton(self._text, request_location=True)
@property
def update_filter(self):
return Filters.create(
name=f"{self._text}ButtonFilter",
func=lambda flt, msg: flt.btn_txt == msg.text,
btn_txt=self._text,
)
or that:
from pyrogram import KeyboardButton
from pyromenu import KButton
class LocationButton(KButton):
@property
def keyboard_button(self):
btn = super().keyboard_button
btn.request_location = True
return btn
and it's will be works well with any built-in classes
pip3 install pyromenu
FAQs
Object-oriented way to build telegram keyboard-menus
We found that pyromenu demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.