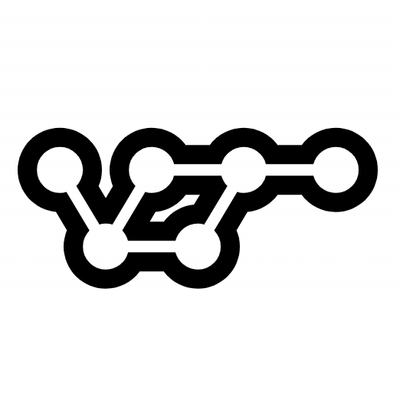
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
sophi-app-internal
Python Packagesophi-app-internal is a Python package designed to facilitate secure and authenticated HTTP request handling, particularly in environments where JWT (JSON Web Token) authentication is used. The package includes utilities for validating JWTs, fetching public keys, constructing HTTP request objects with robust header and parameter management, and interacting with Azure Cosmos DB.
To install the sophi-app-internal package, use the following command:
pip install sophi-app-internal
Before using the package, ensure you have set up the necessary environment variables:
<tenant>.us.auth0.com
.Note: client ID and client secret are not required to validate tokens
You can set these variables in your environment or pass them directly when initializing the Auth0ManagementApiClient
.
Note: You need to register your API on Auth0 before getting a valid audience value.
Here's an example of how to use the token_validator
method:
from sophi_app_internal.integrations.auth0.management_api import Auth0ManagementApiClient
# Initialize the client
auth0_client = Auth0ManagementApiClient()
token = "<your token>" # Ensure you remove the 'Bearer' keyword if present
# Validate the token
try:
claims = auth0_client.token_validator(token)
user_id = claims['sub']
print(user_id)
except Exception as e:
print(e)
Before using the Cosmos DB client, ensure you have set up the necessary variables:
You can set these variables in your environment:
import os
connection_string = os.getenv("COSMOS_CONNECTION_STRING")
db_name = "your_db_name"
container_name = "your_container_name"
Here's an example of how to use the CosmosContainerClient to query and manage documents in Azure Cosmos DB.
import os
import logging
from sophi_app_internal import CosmosContainerClient
# Set up logging
logging.basicConfig(level=logging.INFO)
# Set up connection variables
connection_string = os.getenv("COSMOS_CONNECTION_STRING")
db_name = "your_db_name"
container_name = "your_container_name"
# Initialize the client
client = CosmosContainerClient(connection_string, db_name, container_name)
# Query from the initial container
query = "SELECT * FROM c"
user_id = "1234"
partition_key = [user_id]
documents = client.query_cosmosdb_container(query, partition_key)
print(documents)
# Switch to a different database and container
new_db_name = "another_db_name"
new_container_name = "another_container_name"
client.set_database_and_container(new_db_name, new_container_name)
# Query from the new container
documents = client.query_cosmosdb_container(query)
print(documents)
# Upsert a document
document = {
"id": "1",
"name": "Sample Document",
"description": "This is a sample document."
}
client.upsert_cosmosdb_document(document)
FAQs
Unknown package
We found that sophi-app-internal demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.