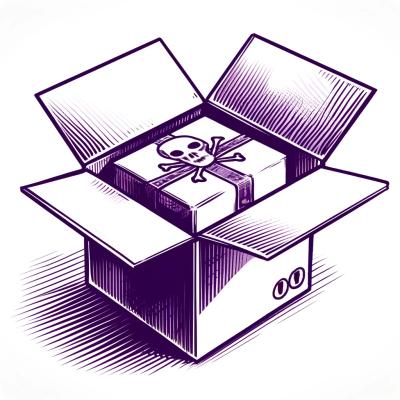
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
DigitalFemsa - the Ruby gem for the Femsa API
Femsa sdk
This SDK is automatically generated by the OpenAPI Generator project:
To build the Ruby code into a gem:
gem build digital_femsa.gemspec
Then either install the gem locally:
gem install ./digital_femsa-1.0.0.gem
(for development, run gem install --dev ./digital_femsa-1.0.0.gem
to install the development dependencies)
or publish the gem to a gem hosting service, e.g. RubyGems.
Finally add this to the Gemfile:
gem 'digital_femsa', '~> 1.0.0'
If the Ruby gem is hosted at a git repository: https://github.com/digitalfemsa/digitalfemsa-ruby, then add the following in the Gemfile:
gem 'digital_femsa', :git => 'https://github.com/digitalfemsa/digitalfemsa-ruby.git'
Include the Ruby code directly using -I
as follows:
ruby -Ilib script.rb
Please follow the installation procedure and then run the following code:
# Load the gem
require 'digital_femsa'
# Setup authorization
DigitalFemsa.configure do |config|
# Configure Bearer authorization: bearerAuth
config.access_token = 'YOUR_BEARER_TOKEN'
# Configure a proc to get access tokens in lieu of the static access_token configuration
config.access_token_getter = -> { 'YOUR TOKEN GETTER PROC' }
# Configure faraday connection
config.configure_faraday_connection { |connection| 'YOUR CONNECTION CONFIG PROC' }
end
api_instance = DigitalFemsa::ApiKeysApi.new
api_key_request = DigitalFemsa::ApiKeyRequest.new({role: 'private'}) # ApiKeyRequest | requested field for a api keys
opts = {
accept_language: 'es', # String | Use for knowing which language to use
x_child_company_id: '6441b6376b60c3a638da80af' # String | In the case of a holding company, the company id of the child company to which will process the request.
}
begin
#Create Api Key
result = api_instance.create_api_key(api_key_request, opts)
p result
rescue DigitalFemsa::ApiError => e
puts "Exception when calling ApiKeysApi->create_api_key: #{e}"
end
All URIs are relative to https://api.digitalfemsa.io
Class | Method | HTTP request | Description |
---|---|---|---|
DigitalFemsa::ApiKeysApi | create_api_key | POST /api_keys | Create Api Key |
DigitalFemsa::ApiKeysApi | delete_api_key | DELETE /api_keys/{id} | Delete Api Key |
DigitalFemsa::ApiKeysApi | get_api_key | GET /api_keys/{id} | Get Api Key |
DigitalFemsa::ApiKeysApi | get_api_keys | GET /api_keys | Get list of Api Keys |
DigitalFemsa::ApiKeysApi | update_api_key | PUT /api_keys/{id} | Update Api Key |
DigitalFemsa::BalancesApi | get_balance | GET /balance | Get a company's balance |
DigitalFemsa::ChargesApi | get_charges | GET /charges | Get A List of Charges |
DigitalFemsa::ChargesApi | orders_create_charge | POST /orders/{id}/charges | Create charge |
DigitalFemsa::ChargesApi | update_charge | PUT /charges/{id} | Update a charge |
DigitalFemsa::CompaniesApi | get_companies | GET /companies | Get List of Companies |
DigitalFemsa::CompaniesApi | get_company | GET /companies/{id} | Get Company |
DigitalFemsa::CustomersApi | create_customer | POST /customers | Create customer |
DigitalFemsa::CustomersApi | create_customer_fiscal_entities | POST /customers/{id}/fiscal_entities | Create Fiscal Entity |
DigitalFemsa::CustomersApi | delete_customer_by_id | DELETE /customers/{id} | Delete Customer |
DigitalFemsa::CustomersApi | get_customer_by_id | GET /customers/{id} | Get Customer |
DigitalFemsa::CustomersApi | get_customers | GET /customers | Get a list of customers |
DigitalFemsa::CustomersApi | update_customer | PUT /customers/{id} | Update customer |
DigitalFemsa::CustomersApi | update_customer_fiscal_entities | PUT /customers/{id}/fiscal_entities/{fiscal_entities_id} | Update Fiscal Entity |
DigitalFemsa::DiscountsApi | orders_create_discount_line | POST /orders/{id}/discount_lines | Create Discount |
DigitalFemsa::DiscountsApi | orders_delete_discount_lines | DELETE /orders/{id}/discount_lines/{discount_lines_id} | Delete Discount |
DigitalFemsa::DiscountsApi | orders_get_discount_line | GET /orders/{id}/discount_lines/{discount_lines_id} | Get Discount |
DigitalFemsa::DiscountsApi | orders_get_discount_lines | GET /orders/{id}/discount_lines | Get a List of Discount |
DigitalFemsa::DiscountsApi | orders_update_discount_lines | PUT /orders/{id}/discount_lines/{discount_lines_id} | Update Discount |
DigitalFemsa::EventsApi | get_event | GET /events/{id} | Get Event |
DigitalFemsa::EventsApi | get_events | GET /events | Get list of Events |
DigitalFemsa::EventsApi | resend_event | POST /events/{event_id}/webhook_logs/{webhook_log_id}/resend | Resend Event |
DigitalFemsa::LogsApi | get_log_by_id | GET /logs/{id} | Get Log |
DigitalFemsa::LogsApi | get_logs | GET /logs | Get List Of Logs |
DigitalFemsa::OrdersApi | cancel_order | POST /orders/{id}/cancel | Cancel Order |
DigitalFemsa::OrdersApi | create_order | POST /orders | Create order |
DigitalFemsa::OrdersApi | get_order_by_id | GET /orders/{id} | Get Order |
DigitalFemsa::OrdersApi | get_orders | GET /orders | Get a list of Orders |
DigitalFemsa::OrdersApi | order_cancel_refund | DELETE /orders/{id}/refunds/{refund_id} | Cancel Refund |
DigitalFemsa::OrdersApi | order_refund | POST /orders/{id}/refunds | Refund Order |
DigitalFemsa::OrdersApi | orders_create_capture | POST /orders/{id}/capture | Capture Order |
DigitalFemsa::OrdersApi | update_order | PUT /orders/{id} | Update Order |
DigitalFemsa::PaymentLinkApi | cancel_checkout | PUT /checkouts/{id}/cancel | Cancel Payment Link |
DigitalFemsa::PaymentLinkApi | create_checkout | POST /checkouts | Create Unique Payment Link |
DigitalFemsa::PaymentLinkApi | email_checkout | POST /checkouts/{id}/email | Send an email |
DigitalFemsa::PaymentLinkApi | get_checkout | GET /checkouts/{id} | Get a payment link by ID |
DigitalFemsa::PaymentLinkApi | get_checkouts | GET /checkouts | Get a list of payment links |
DigitalFemsa::PaymentLinkApi | sms_checkout | POST /checkouts/{id}/sms | Send an sms |
DigitalFemsa::PaymentMethodsApi | create_customer_payment_methods | POST /customers/{id}/payment_sources | Create Payment Method |
DigitalFemsa::PaymentMethodsApi | delete_customer_payment_methods | DELETE /customers/{id}/payment_sources/{payment_method_id} | Delete Payment Method |
DigitalFemsa::PaymentMethodsApi | get_customer_payment_methods | GET /customers/{id}/payment_sources | Get Payment Methods |
DigitalFemsa::PaymentMethodsApi | update_customer_payment_methods | PUT /customers/{id}/payment_sources/{payment_method_id} | Update Payment Method |
DigitalFemsa::ProductsApi | orders_create_product | POST /orders/{id}/line_items | Create Product |
DigitalFemsa::ProductsApi | orders_delete_product | DELETE /orders/{id}/line_items/{line_item_id} | Delete Product |
DigitalFemsa::ProductsApi | orders_update_product | PUT /orders/{id}/line_items/{line_item_id} | Update Product |
DigitalFemsa::ShippingContactsApi | create_customer_shipping_contacts | POST /customers/{id}/shipping_contacts | Create a shipping contacts |
DigitalFemsa::ShippingContactsApi | delete_customer_shipping_contacts | DELETE /customers/{id}/shipping_contacts/{shipping_contacts_id} | Delete shipping contacts |
DigitalFemsa::ShippingContactsApi | update_customer_shipping_contacts | PUT /customers/{id}/shipping_contacts/{shipping_contacts_id} | Update shipping contacts |
DigitalFemsa::ShippingsApi | orders_create_shipping | POST /orders/{id}/shipping_lines | Create Shipping |
DigitalFemsa::ShippingsApi | orders_delete_shipping | DELETE /orders/{id}/shipping_lines/{shipping_id} | Delete Shipping |
DigitalFemsa::ShippingsApi | orders_update_shipping | PUT /orders/{id}/shipping_lines/{shipping_id} | Update Shipping |
DigitalFemsa::TaxesApi | orders_create_taxes | POST /orders/{id}/tax_lines | Create Tax |
DigitalFemsa::TaxesApi | orders_delete_taxes | DELETE /orders/{id}/tax_lines/{tax_id} | Delete Tax |
DigitalFemsa::TaxesApi | orders_update_taxes | PUT /orders/{id}/tax_lines/{tax_id} | Update Tax |
DigitalFemsa::TransactionsApi | get_transaction | GET /transactions/{id} | Get transaction |
DigitalFemsa::TransactionsApi | get_transactions | GET /transactions | Get List transactions |
DigitalFemsa::TransfersApi | get_transfer | GET /transfers/{id} | Get Transfer |
DigitalFemsa::TransfersApi | get_transfers | GET /transfers | Get a list of transfers |
DigitalFemsa::WebhookKeysApi | create_webhook_key | POST /webhook_keys | Create Webhook Key |
DigitalFemsa::WebhookKeysApi | delete_webhook_key | DELETE /webhook_keys/{id} | Delete Webhook key |
DigitalFemsa::WebhookKeysApi | get_webhook_key | GET /webhook_keys/{id} | Get Webhook Key |
DigitalFemsa::WebhookKeysApi | get_webhook_keys | GET /webhook_keys | Get List of Webhook Keys |
DigitalFemsa::WebhookKeysApi | update_webhook_key | PUT /webhook_keys/{id} | Update Webhook Key |
DigitalFemsa::WebhooksApi | create_webhook | POST /webhooks | Create Webhook |
DigitalFemsa::WebhooksApi | delete_webhook | DELETE /webhooks/{id} | Delete Webhook |
DigitalFemsa::WebhooksApi | get_webhook | GET /webhooks/{id} | Get Webhook |
DigitalFemsa::WebhooksApi | get_webhooks | GET /webhooks | Get List of Webhooks |
DigitalFemsa::WebhooksApi | test_webhook | POST /webhooks/{id}/test | Test Webhook |
DigitalFemsa::WebhooksApi | update_webhook | PUT /webhooks/{id} | Update Webhook |
Authentication schemes defined for the API:
FAQs
Unknown package
We found that digital_femsa demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.