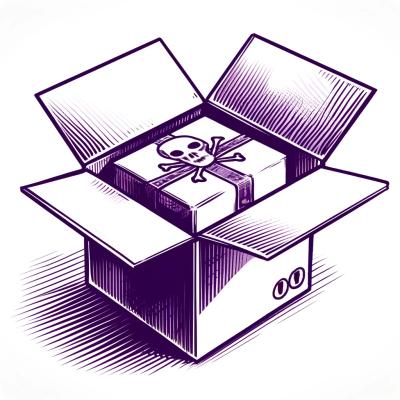
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
notifo-io-easierlife
Advanced tools
SwaggerClient - the Ruby gem for the Notifo API
No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
This SDK is automatically generated by the Swagger Codegen project:
To build the Ruby code into a gem:
gem build swagger_client.gemspec
Then either install the gem locally:
gem install ./swagger_client-1.0.0.gem
(for development, run gem install --dev ./swagger_client-1.0.0.gem
to install the development dependencies)
or publish the gem to a gem hosting service, e.g. RubyGems.
Finally add this to the Gemfile:
gem 'swagger_client', '~> 1.0.0'
If the Ruby gem is hosted at a git repository: https://github.com/GIT_USER_ID/GIT_REPO_ID, then add the following in the Gemfile:
gem 'swagger_client', :git => 'https://github.com/GIT_USER_ID/GIT_REPO_ID.git'
Include the Ruby code directly using -I
as follows:
ruby -Ilib script.rb
Please follow the installation procedure and then run the following code:
# Load the gem
require 'swagger_client'
api_instance = SwaggerClient::AppsApi.new
app_id = 'app_id_example' # String | The id of the app.
contributor_id = 'contributor_id_example' # String | The contributor to remove.
begin
#Delete an app contributor.
result = api_instance.apps_delete_contributor(app_id, contributor_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_delete_contributor: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
app_id = 'app_id_example' # String | The id of the app where the email templates belong to.
language = 'language_example' # String | The language.
begin
#Delete an app email template.
api_instance.apps_delete_email_template(app_id, language)
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_delete_email_template: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
app_id = 'app_id_example' # String | The id of the app.
begin
#Get app by id.
result = api_instance.apps_get_app(app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_get_app: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
begin
#Get the user apps.
result = api_instance.apps_get_apps
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_get_apps: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
app_id = 'app_id_example' # String | The id of the app where the email templates belong to.
begin
#Get the app email templates.
result = api_instance.apps_get_email_templates(app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_get_email_templates: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
body = SwaggerClient::UpsertAppDto.new # UpsertAppDto | The request object.
begin
#Create an app.
result = api_instance.apps_post_app(body)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_post_app: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
body = SwaggerClient::AddContributorDto.new # AddContributorDto | The request object.
app_id = 'app_id_example' # String | The id of the app.
begin
#Add an app contributor.
result = api_instance.apps_post_contributor(body, app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_post_contributor: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
body = SwaggerClient::CreateEmailTemplateDto.new # CreateEmailTemplateDto | The request object.
app_id = 'app_id_example' # String | The id of the app where the email templates belong to.
begin
#Create an app email template.
result = api_instance.apps_post_email_template(body, app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_post_email_template: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
body = SwaggerClient::UpsertAppDto.new # UpsertAppDto | The request object.
app_id = 'app_id_example' # String | The app id to update.
begin
#Update an app.
result = api_instance.apps_put_app(body, app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_put_app: #{e}"
end
api_instance = SwaggerClient::AppsApi.new
body = SwaggerClient::EmailTemplateDto.new # EmailTemplateDto | The request object.
app_id = 'app_id_example' # String | The id of the app where the email templates belong to.
language = 'language_example' # String | The language.
begin
#Update an app email template.
api_instance.apps_put_email_template(body, app_id, language)
rescue SwaggerClient::ApiError => e
puts "Exception when calling AppsApi->apps_put_email_template: #{e}"
end
api_instance = SwaggerClient::ConfigsApi.new
begin
#Get all supported languages.
result = api_instance.configs_get_languages
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling ConfigsApi->configs_get_languages: #{e}"
end
api_instance = SwaggerClient::ConfigsApi.new
begin
#Get all supported timezones.
result = api_instance.configs_get_timezones
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling ConfigsApi->configs_get_timezones: #{e}"
end
api_instance = SwaggerClient::EventsApi.new
app_id = 'app_id_example' # String | The app where the events belongs to.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query events.
result = api_instance.events_get_events(app_id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling EventsApi->events_get_events: #{e}"
end
api_instance = SwaggerClient::EventsApi.new
body = SwaggerClient::PublishManyDto.new # PublishManyDto | The publish request.
app_id = 'app_id_example' # String | The app where the events belongs to.
begin
#Publish events.
api_instance.events_post_events(body, app_id)
rescue SwaggerClient::ApiError => e
puts "Exception when calling EventsApi->events_post_events: #{e}"
end
api_instance = SwaggerClient::LogsApi.new
app_id = 'app_id_example' # String | The app where the log entries belongs to.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query log entries.
result = api_instance.logs_get_logs(app_id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling LogsApi->logs_get_logs: #{e}"
end
api_instance = SwaggerClient::MediaApi.new
app_id = 'app_id_example' # String | The app id where the media belongs to.
file_name = 'file_name_example' # String | The file name of the media.
begin
#Delete a media.
api_instance.media_delete(app_id, file_name)
rescue SwaggerClient::ApiError => e
puts "Exception when calling MediaApi->media_delete: #{e}"
end
api_instance = SwaggerClient::MediaApi.new
app_id = 'app_id_example' # String | The app id where the media belongs to.
file_name = 'file_name_example' # String | The name of the media to download.
opts = {
cache: 789, # Integer | The cache duration.
download: 56, # Integer | Set it to 1 to create a download response.
width: 56, # Integer | The target width when an image.
height: 56, # Integer | The target height when an image.
quality: 56, # Integer | The target quality when an image.
preset: 'preset_example', # String | A preset dimension.
mode: SwaggerClient::ResizeMode.new, # ResizeMode | The resize mode.
focus_x: 3.4, # Float | The x position of the focues point.
focus_y: 3.4, # Float | The y position of the focues point.
force: true # BOOLEAN | True to resize it and clear the cache.
}
begin
#Download a media object.
result = api_instance.media_download(app_id, file_name, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling MediaApi->media_download: #{e}"
end
api_instance = SwaggerClient::MediaApi.new
app_id = 'app_id_example' # String | The app id where the media belongs to.
file_name = 'file_name_example' # String | The name of the media to download.
opts = {
cache: 789, # Integer | The cache duration.
download: 56, # Integer | Set it to 1 to create a download response.
width: 56, # Integer | The target width when an image.
height: 56, # Integer | The target height when an image.
quality: 56, # Integer | The target quality when an image.
preset: 'preset_example', # String | A preset dimension.
mode: SwaggerClient::ResizeMode.new, # ResizeMode | The resize mode.
focus_x: 3.4, # Float | The x position of the focues point.
focus_y: 3.4, # Float | The y position of the focues point.
force: true # BOOLEAN | True to resize it and clear the cache.
}
begin
#Download a media object.
result = api_instance.media_download2(app_id, file_name, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling MediaApi->media_download2: #{e}"
end
api_instance = SwaggerClient::MediaApi.new
app_id = 'app_id_example' # String | The app where the media belongs to.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query media items.
result = api_instance.media_get_medias(app_id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling MediaApi->media_get_medias: #{e}"
end
api_instance = SwaggerClient::MediaApi.new
app_id = 'app_id_example' # String | The app id where the media belongs to.
opts = {
file: 'file_example' # String |
}
begin
#Upload a media object.
api_instance.media_upload(app_id, opts)
rescue SwaggerClient::ApiError => e
puts "Exception when calling MediaApi->media_upload: #{e}"
end
api_instance = SwaggerClient::TemplatesApi.new
app_id = 'app_id_example' # String | The app where the templates belong to.
code = 'code_example' # String | The template code to delete.
begin
#Delete a template.
result = api_instance.templates_delete_template(app_id, code)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling TemplatesApi->templates_delete_template: #{e}"
end
api_instance = SwaggerClient::TemplatesApi.new
app_id = 'app_id_example' # String | The app where the templates belongs to.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query templates.
result = api_instance.templates_get_templates(app_id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling TemplatesApi->templates_get_templates: #{e}"
end
api_instance = SwaggerClient::TemplatesApi.new
body = SwaggerClient::UpsertTemplatesDto.new # UpsertTemplatesDto | The upsert request.
app_id = 'app_id_example' # String | The app where the templates belong to.
begin
#Upsert templates.
result = api_instance.templates_post_templates(body, app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling TemplatesApi->templates_post_templates: #{e}"
end
api_instance = SwaggerClient::TopicsApi.new
app_id = 'app_id_example' # String | The app where the topics belongs to.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query topics.
result = api_instance.topics_get_topics(app_id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling TopicsApi->topics_get_topics: #{e}"
end
api_instance = SwaggerClient::UserApi.new
topic = 'topic_example' # String | The topic path.
begin
#Deletes a user subscription.
api_instance.user_delete_subscription(topic)
rescue SwaggerClient::ApiError => e
puts "Exception when calling UserApi->user_delete_subscription: #{e}"
end
api_instance = SwaggerClient::UserApi.new
topic = 'topic_example' # String | The topic path.
begin
#Gets a user subscription.
result = api_instance.user_get_subscription(topic)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UserApi->user_get_subscription: #{e}"
end
api_instance = SwaggerClient::UserApi.new
begin
#Get the current user.
result = api_instance.user_get_user
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UserApi->user_get_user: #{e}"
end
api_instance = SwaggerClient::UserApi.new
body = SwaggerClient::SubscriptionDto.new # SubscriptionDto | The subscription settings.
begin
#Creates a user subscription.
api_instance.user_post_subscription(body)
rescue SwaggerClient::ApiError => e
puts "Exception when calling UserApi->user_post_subscription: #{e}"
end
api_instance = SwaggerClient::UserApi.new
body = SwaggerClient::UpdateProfileDto.new # UpdateProfileDto | The upsert request.
begin
#Update the user.
result = api_instance.user_post_user(body)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UserApi->user_post_user: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
app_id = 'app_id_example' # String | The app where the users belong to.
id = 'id_example' # String | The user id.
prefix = 'prefix_example' # String | The topic prefix.
begin
#Remove an allowed topic.
api_instance.users_delete_allowed_topic(app_id, id, prefix)
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_delete_allowed_topic: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
app_id = 'app_id_example' # String | The app where the user belongs to.
id = 'id_example' # String | The user id.
prefix = 'prefix_example' # String | The topic prefix.
begin
#Remove a user subscriptions.
api_instance.users_delete_subscription(app_id, id, prefix)
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_delete_subscription: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
app_id = 'app_id_example' # String | The app where the users belongs to.
id = 'id_example' # String | The user id to delete.
begin
#Delete a user.
result = api_instance.users_delete_user(app_id, id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_delete_user: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
app_id = 'app_id_example' # String | The app where the user belongs to.
id = 'id_example' # String | The user id.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query user subscriptions.
result = api_instance.users_get_subscriptions(app_id, id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_get_subscriptions: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
app_id = 'app_id_example' # String | The app where the user belongs to.
id = 'id_example' # String | The user id.
begin
#Get a user.
result = api_instance.users_get_user(app_id, id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_get_user: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
app_id = 'app_id_example' # String | The app where the users belongs to.
opts = {
query: 'query_example', # String | The optional query to search for items.
take: 56, # Integer | The number of items to return.
skip: 56 # Integer | The number of items to skip.
}
begin
#Query users.
result = api_instance.users_get_users(app_id, opts)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_get_users: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
body = SwaggerClient::AddAllowedTopicDto.new # AddAllowedTopicDto | The upsert request.
app_id = 'app_id_example' # String | The app where the users belong to.
id = 'id_example' # String | The user id.
begin
#Add an allowed topic.
api_instance.users_post_allowed_topic(body, app_id, id)
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_post_allowed_topic: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
body = SwaggerClient::SubscriptionDto.new # SubscriptionDto | The subscription object.
app_id = 'app_id_example' # String | The app where the user belongs to.
id = 'id_example' # String | The user id.
begin
#Upsert a user subscriptions.
api_instance.users_post_subscription(body, app_id, id)
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_post_subscription: #{e}"
end
api_instance = SwaggerClient::UsersApi.new
body = SwaggerClient::UpsertUsersDto.new # UpsertUsersDto | The upsert request.
app_id = 'app_id_example' # String | The app where the users belong to.
begin
#Upsert users.
result = api_instance.users_post_users(body, app_id)
p result
rescue SwaggerClient::ApiError => e
puts "Exception when calling UsersApi->users_post_users: #{e}"
end
All URIs are relative to https://app.notifo.io
Class | Method | HTTP request | Description |
---|---|---|---|
SwaggerClient::AppsApi | apps_delete_contributor | POST /api/apps/{appId}/contributors/{contributorId} | Delete an app contributor. |
SwaggerClient::AppsApi | apps_delete_email_template | POST /api/apps/{appId}/email-templates/{language} | Delete an app email template. |
SwaggerClient::AppsApi | apps_get_app | GET /api/apps/{appId} | Get app by id. |
SwaggerClient::AppsApi | apps_get_apps | GET /api/apps | Get the user apps. |
SwaggerClient::AppsApi | apps_get_email_templates | GET /api/apps/{appId}/email-templates | Get the app email templates. |
SwaggerClient::AppsApi | apps_post_app | POST /api/apps | Create an app. |
SwaggerClient::AppsApi | apps_post_contributor | POST /api/apps/{appId}/contributors | Add an app contributor. |
SwaggerClient::AppsApi | apps_post_email_template | POST /api/apps/{appId}/email-templates | Create an app email template. |
SwaggerClient::AppsApi | apps_put_app | POST /api/apps/{appId} | Update an app. |
SwaggerClient::AppsApi | apps_put_email_template | PUT /api/apps/{appId}/email-templates/{language} | Update an app email template. |
SwaggerClient::ConfigsApi | configs_get_languages | GET /api/languages | Get all supported languages. |
SwaggerClient::ConfigsApi | configs_get_timezones | GET /api/timezones | Get all supported timezones. |
SwaggerClient::EventsApi | events_get_events | GET /api/apps/{appId}/events | Query events. |
SwaggerClient::EventsApi | events_post_events | POST /api/apps/{appId}/events | Publish events. |
SwaggerClient::LogsApi | logs_get_logs | GET /api/apps/{appId}/logs | Query log entries. |
SwaggerClient::MediaApi | media_delete | DELETE /api/apps/{appId}/media/{fileName} | Delete a media. |
SwaggerClient::MediaApi | media_download | GET /api/apps/{appId}/media/{fileName} | Download a media object. |
SwaggerClient::MediaApi | media_download2 | GET /api/assets/{appId}/{fileName} | Download a media object. |
SwaggerClient::MediaApi | media_get_medias | GET /api/apps/{appId}/media | Query media items. |
SwaggerClient::MediaApi | media_upload | POST /api/apps/{appId}/media | Upload a media object. |
SwaggerClient::TemplatesApi | templates_delete_template | DELETE /api/apps/{appId}/templates/{code} | Delete a template. |
SwaggerClient::TemplatesApi | templates_get_templates | GET /api/apps/{appId}/templates | Query templates. |
SwaggerClient::TemplatesApi | templates_post_templates | POST /api/apps/{appId}/templates | Upsert templates. |
SwaggerClient::TopicsApi | topics_get_topics | GET /api/apps/{appId}/topics | Query topics. |
SwaggerClient::UserApi | user_delete_subscription | DELETE /api/me/subscriptions/{topic} | Deletes a user subscription. |
SwaggerClient::UserApi | user_get_subscription | GET /api/me/subscriptions/{topic} | Gets a user subscription. |
SwaggerClient::UserApi | user_get_user | GET /api/me | Get the current user. |
SwaggerClient::UserApi | user_post_subscription | POST /api/me/subscriptions | Creates a user subscription. |
SwaggerClient::UserApi | user_post_user | POST /api/me | Update the user. |
SwaggerClient::UsersApi | users_delete_allowed_topic | DELETE /api/apps/{appId}/users/{id}/allowed-topics/{prefix} | Remove an allowed topic. |
SwaggerClient::UsersApi | users_delete_subscription | DELETE /api/apps/{appId}/users/{id}/subscriptions/{prefix} | Remove a user subscriptions. |
SwaggerClient::UsersApi | users_delete_user | DELETE /api/apps/{appId}/users/{id} | Delete a user. |
SwaggerClient::UsersApi | users_get_subscriptions | GET /api/apps/{appId}/users/{id}/subscriptions | Query user subscriptions. |
SwaggerClient::UsersApi | users_get_user | GET /api/apps/{appId}/users/{id} | Get a user. |
SwaggerClient::UsersApi | users_get_users | GET /api/apps/{appId}/users | Query users. |
SwaggerClient::UsersApi | users_post_allowed_topic | POST /api/apps/{appId}/users/{id}/allowed-topics | Add an allowed topic. |
SwaggerClient::UsersApi | users_post_subscription | POST /api/apps/{appId}/users/{id}/subscriptions | Upsert a user subscriptions. |
SwaggerClient::UsersApi | users_post_users | POST /api/apps/{appId}/users | Upsert users. |
All endpoints do not require authorization.
FAQs
Unknown package
We found that notifo-io-easierlife demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.