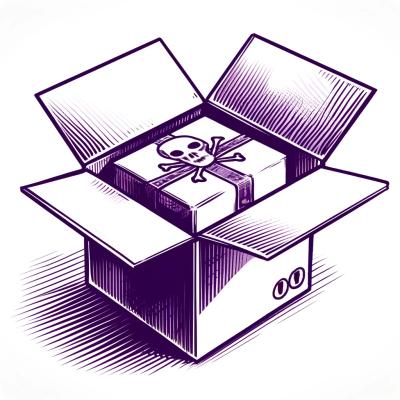
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
This gem offers a Ruby interface for the Voicemeeter Remote API.
For an outline of past/future changes refer to: CHANGELOG
bundle add voicemeeter
bundle install
Use
Simplest use case, request a Remote class of a kind, then pass a block to run.
Login and logout are handled for you in this scenario.
main.rb
require "voicemeeter"
class Main
def run
Voicemeeter::Remote.new(:banana).run do |vm|
vm.strip[0].label = "podmic"
vm.strip[0].mute = true
puts "strip 0 #{vm.strip[0].label} mute was set to #{vm.strip[0].mute}"
vm.bus[3].gain = -6.3
vm.bus[4].eq.on = true
info = [
"bus 3 gain has been set to #{vm.bus[3].gain}",
"bus 4 eq has been set to #{vm.bus[4].eq.on}"
]
puts info
end
end
end
Main.new.run if $PROGRAM_NAME == __FILE__
Otherwise you must remember to call vm.login
vm.logout
at the start/end of your code.
KIND_ID
Pass the kind of Voicemeeter as an argument. KIND_ID may be:
:basic
:banana
:potato
Available commands
The following attributes are available.
mono
: booleansolo
: booleanmute
: booleangain
: float, from -60.0 to 12.0audibility
: float, from 0.0 to 10.0limit
: int, from -40 to 12A1 - A5
, B1 - B3
: booleanlabel
: stringmc
: booleankaraoke
: int, from 0 to 4bass
: float, from -12.0 to 12.0mid
: float, from -12.0 to 12.0treble
: float, from -12.0 to 12.0reverb
: float, from 0.0 to 10.0delay
: float, from 0.0 to 10.0fx1
: float, from 0.0 to 10.0fx2
: float, from 0.0 to 10.0pan_x
: float, from -0.5 to 0.5pan_y
: float, from 0.0 to 1.0color_x
: float, from -0.5 to 0.5color_y
: float, from 0.0 to 1.0fx_x
: float, from -0.5 to 0.5fx_y
: float, from 0.0 to 1.0postreverb
: booleanpostdelay
: booleanpostfx1
: booleanpostfx2
: booleanexample:
vm.strip[3].gain = 3.7
puts vm.strip[0].label
The following methods are available.
appgain(name, value)
: string, float, from 0.0 to 1.0Set the gain in db by value for the app matching name.
appmute(name, value)
: string, boolSet mute state as value for the app matching name.
example:
vm.strip[5].appgain("Spotify", 0.5)
vm.strip[5].appmute("Spotify", true)
The following attributes are available.
knob
: float, from 0.0 to 10.0gainin
: float, from -24.0 to 24.0ratio
: float, from 1.0 to 8.0threshold
: float, from -40.0 to -3.0attack
: float, from 0.0 to 200.0release
: float, from 0.0 to 5000.0knee
: float, from 0.0 to 1.0gainout
: float, from -24.0 to 24.0makeup
: booleanexample:
puts vm.strip[4].comp.knob
Strip Comp parameters are defined for PhysicalStrips.
knob
defined for all versions, all other parameters potato only.
The following attributes are available.
knob
: float, from 0.0 to 10.0threshold
: float, from -60.0 to -10.0damping
: float, from -60.0 to -10.0bpsidechain
: int, from 100 to 4000attack
: float, from 0.0 to 1000.0hold
: float, from 0.0 to 5000.0release
: float, from 0.0 to 5000.0example:
vm.strip[2].gate.attack = 300.8
Strip Gate parameters are defined for PhysicalStrips.
knob
defined for all versions, all other parameters potato only.
The following attributes are available.
knob
: float, from 0.0 to 10.0example:
vm.strip[0].denoiser.knob = 0.5
Strip Denoiser parameters are defined for PhysicalStrips, potato version only.
The following attributes are available.
on
: booleanab
: booleanexample:
vm.strip[0].eq.ab = true
Strip EQ parameters are defined for PhysicalStrips, potato version only.
gain
: float, from -60.0 to 12.0example:
vm.strip[3].gainlayer[3].gain = 3.7
Gainlayers are defined for potato version only.
The following attributes are available.
prefader
postfader
postmute
example:
puts vm.strip[3].levels.prefader
Level properties will return -200.0 if no audio detected.
The following attributes are available.
mono
: booleanmute
: booleansel
: booleangain
: float, from -60.0 to 12.0label
: stringreturnreverb
: float, from 0.0 to 10.0returndelay
: float, from 0.0 to 10.0returnfx1
: float, from 0.0 to 10.0returnfx2
: float, from 0.0 to 10.0monitor
: booleanexample:
vm.bus[3].gain = 3.7
puts vm.bus[0].label
vm.bus[4].mono = true
The following attributes are available.
on
: booleanab
: booleanexample:
vm.bus[4].eq.on = true
The following attributes are available.
normal
: booleanamix
: booleanbmix
: booleancomposite
: booleantvmix
: booleanupmix21
: booleanupmix41
: booleanupmix61
: booleancenteronly
: booleanlfeonly
: booleanrearonly
: booleanThe following methods are available.
get
example:
vm.bus[4].mode.amix = true
puts vm.bus[3].mode.get
The following attributes are available.
all
example:
puts vm.bus[0].levels.all
levels.all
will return -200.0 if no audio detected.
The following methods are available
fadeto(amount, time)
: float, intfadeby(amount, time)
: float, intModify gain to or by the selected amount in db over a time interval in ms.
example:
vm.strip[0].fadeto(-10.3, 1000)
vm.bus[3].fadeby(-5.6, 500)
The following attributes are available
name
: strsr
: intwdm
: strks
: strmme
: strasio
: strexample:
puts vm.strip[0].device.name
vm.bus[0].device.asio = "Audient USB Audio ASIO Driver"
strip|bus device parameters are defined for physical channels only.
name, sr are read only. wdm, ks, mme, asio are write only.
Three modes defined: state, stateonly and trigger.
state
: booleanstateonly
: booleantrigger
: booleanexample:
vm.button[37].state = true
vm.button[55].trigger = false
The following attributes accept boolean values.
loop
: booleanA1 - A5
: booleanB1 - A3
: booleanThe following methods are available
play
stop
pause
record
ff
rew
load(filepath)
: stringexample:
vm.recorder.play
vm.recorder.stop
# Enable loop play
vm.recorder.loop = true
# Disable recorder out channel B2
vm.recorder.B2 = false
# filepath as string
vm.recorder.load('C:\music\mytune.mp3')
vm.vban.enable
| vm.vban.disable
Turn VBAN on or offThe following attributes are available.
on
: booleanname
: stringip
: stringport
: int, range from 1024 to 65535sr
: int, (11025, 16000, 22050, 24000, 32000, 44100, 48000, 64000, 88200, 96000)channel
: int, from 1 to 8bit
: int, 16 or 24quality
: int, from 0 to 4route
: int, from 0 to 8SR, channel and bit are defined as readonly for instreams. Attempting to write to those parameters will throw an error. They are read and write for outstreams.
example:
# turn VBAN on
vm.vban.enable
# turn on vban instream 0
vm.vban.instream[0].on = true
# set bit property for outstream 3 to 24
vm.vban.outstream[3].bit = 24
Certain 'special' commands are defined by the API as performing actions rather than setting values. The following methods are available:
show
: Bring Voiceemeter GUI to the fronthide
: Hide Voicemeeter GUIshutdown
: Shuts down the GUIrestart
: Restart the audio engineThe following attributes are write only and accept boolean values.
showvbanchat
: booleanlock
: booleanexample:
vm.command.restart
vm.command.showvbanchat = true
ins
outs
: Returns the number of input/output devicesinput(i)
output(i)
: Returns a hash of device properties for device[i]example:
vm.run { |vm| (0...vm.device.ins).each { puts vm.device.input(_1) } }
The following attributes are available:
on
: booleanab
: booleanexample:
vm.fx.reverb.on = true
vm.fx.delay.ab = true
The following attributes are available:
postfadercomposite
: booleanpostfxinsert
: booleanexample:
vm.patch.postfxinsert = false
get
: intset(patch_in)
: int, valid range determined by connected device.example:
vm.patch.asio[3].set(4)
i, from 0 to 10
get
: intset(patch_out)
: int, valid range determined by connected device.example:
vm.patch.A3[5].set(3)
i, from 0 to 8.
get
: intset(channel)
: int, from 0 up to number of channels depending on version.example:
vm.patch.composite[7].set(4)
i, from 0 to 8.
on
: booleanexample:
vm.patch.insert[18].on = true
i, from 0 up to number of channels depending on version.
The following attributes are available:
sr
: intasiosr
: booleanmonitoronsel
: booleanslidermode
: booleanexample:
vm.option.sr = 48_000
The following attributes are available:
mme
: intwdm
: intks
: intasio
: intexample:
vm.option.buffer.wdm = 512
get
: intset(delay)
: int, from 0 to 500example:
vm.option.delay[4].set(30)
i, from 0 up to 4.
The following attributes are available:
channel
: int, returns the midi channelcurrent
: int, returns the current (or most recently pressed) keyThe following methods are available:
get(key)
: int, returns most recent velocity value for a keyexample:
puts vm.midi.get(12)
get may return nil if no value for requested key in midi cache
apply
Set many strip/bus/macrobutton/vban parameters at once, for example:vm.apply(
{
"strip-0" => {mute: true, gain: 3.2, A1: true},
"bus-3" => {gain: -3.2, eq: {on: true}},
"button-39" => {stateonly: true},
"vban-outstream-3" => {on: true, bit: 24}
}
)
Or for each class you may do:
vm.strip[0].apply(mute: true, gain: 3.2, A1: true)
vm.vban.outstream[0].apply(on: true, name: "streamname", bit: 24)
vm.apply_config(<configname>)
You may load config files in YAML format. Three example configs have been included with the package. Remember to save current settings before loading a config. To set one you may do:
require "voicemeeter"
Voicemeeter::Remote.new(:banana).run { |vm| vm.apply_config(:example) }
will load a config file at mydir/configs/banana/example.yml for Voicemeeter Banana.
By default, NO events listened for.
Use keyword args pdirty
, mdirty
, midi
, ldirty
to initialize event updates.
example:
require 'voicemeeter'
# Set updates to occur every 50ms
# Listen for level updates
Voicemeeter::Remote.new(:banana, ratelimit: 0.05, ldirty: true).run do
...
vm.on
Use the on
method to register blocks on the vm object to be called back later.
example:
# register a block with the on method
class App():
def initialize(vm)
@vm = vm
@vm.on :pdirty do
...
end
vm.event
Use the event class to toggle updates as necessary.
The following attributes are available:
pdirty
: booleanmdirty
: booleanmidi
: booleanldirty
: booleanexample:
vm.event.ldirty = true
vm.event.pdirty = false
Or add, remove an array of events.
The following methods are available:
add(events)
remove(events)
get
example:
vm.event.add(%i[pdirty mdirty midi ldirty])
vm.event.remove(%i[pdirty mdirty midi ldirty])
# get a list of currently subscribed
p vm.event.get
Voicemeeter Module
You may pass the following optional keyword arguments:
sync
: boolean=false, force the getters to wait for dirty parameters to clear. For most cases leave this as false.ratelimit
: float=0.033, how often to check for updates in ms.pdirty
: boolean=true, parameter updatesmdirty
: boolean=true, macrobutton updatesmidi
: boolean=true, midi updatesldirty
: boolean=false, level updatesbits
: int=64, (may be one of 32 or 64), overrides the type of Voicemeeter GUI {Remote}.run_voicemeeter will launch.Access to lower level Getters and Setters are provided with these functions:
vm.get(param, string=false)
: For getting the value of any parameter. Set string to true if getting a property value expected to return a string.vm.set(param, value)
: For setting the value of any parameter.Access to lower level polling functions are provided with these functions:
vm.pdirty?
: Returns true iff a parameter has been updated.vm.mdirty?
: Returns true iff a macrobutton has been updated.example:
vm.get("Strip[2].Mute")
vm.set("Strip[4].Label", "stripname")
vm.set("Strip[0].Gain", -3.6)
Errors::VMError
: Base Voicemeeter error class.Errors::VMInstallError
: Raised when errors occur during installation.Errors::VMCAPIError
: Raised when the C-API returns error codes.
fn_name
: name of the C-API function that failed.code
: error code.To enable logging set an environmental variable VM_LOG_LEVEL
to the appropriate level.
example in powershell:
$env:VM_LOG_LEVEL="DEBUG"
To run all tests:
Bundle exec rake
FAQs
Unknown package
We found that voicemeeter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.