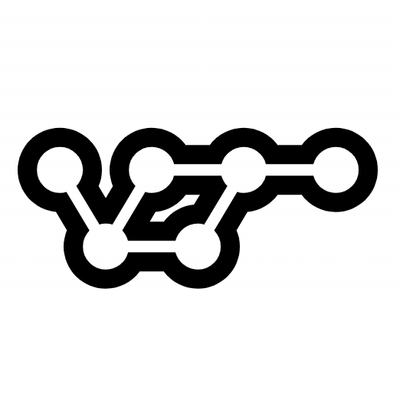
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@anxing131/casdoor-nodejs-sdk
Advanced tools
This is Casdoor's SDK for NodeJS will allow you to easily connect your application to the Casdoor authentication system without having to implement it from scratch.
Casdoor SDK is very simple to use. We will show you the steps below.
Noted that this sdk has been applied to casnode, if you still don’t know how to use it after reading README.md, you can refer to it
# NPM
npm i casdoor-nodejs-sdk
# Yarn
yarn add casdoor-nodejs-sdk
Initialization requires 5 parameters, which are all string type:
Name (in order) | Must | Description |
---|---|---|
issuer | Yes | casdoor OIDC Issuer endpoint |
clientId | Yes | your Casdoor OAuth Client ID |
clientSecret | Yes | your Casdoor OAuth Client Secret |
casdoorEndpoint | No | your Casdoor API endpoint |
import { SDK, AuthConfig } from 'casdoor-nodejs-sdk'
const authCfg: AuthConfig = {
issuer: 'https://b4464f21-7cef-48f1-9591-4acbe1a4590e.mock.pstmn.io/.well-known/openid-configuration',
clientId: '673c704036c6bcd04aaa',
clientSecret: '2ba9708658b3036206b9b96ee8872242d3f9e956',
casdoorEndpoint: 'http://casdoor.anxing.io'
}
const sdk = new SDK(authCfg)
// call sdk to handle
After casdoor verification passed, it will be redirected to your application with code and state, like http://forum.casbin.org?code=xxx&state=yyyy
.
Your web application can get the code
,state
and call getOAuthToken(code, state)
, then parse out jwt token.
The general process is as follows:
import { SDK, AuthConfig } from 'casdoor-nodejs-sdk'
const authCfg: AuthConfig = {
issuer: 'https://b4464f21-7cef-48f1-9591-4acbe1a4590e.mock.pstmn.io/.well-known/openid-configuration',
clientId: '673c704036c6bcd04aaa',
clientSecret: '2ba9708658b3036206b9b96ee8872242d3f9e956',
casdoorEndpoint: 'http://casdoor.anxing.io'
}
const sdk = new SDK(authCfg)
const tokenSet = await sdk.callback({
code: 'your authorization code',
state: 'your casdoor application name'
})
const parseToken = sdk.parseJwtToken(tokenSet.access_token || '')
test env parameters
Name (in order) | Must | Description |
---|---|---|
TEST_CASDOOR_ISSUER | Yes | Casdoor OIDC Issuer endpoint |
TEST_CASDOOR_CLIENT_ID | Yes | your Casdoor OAuth Client ID |
TEST_CASDOOR_CLIENT_SECRET | Yes | your Casdoor OAuth Client Secret |
TEST_AUTHORIZATION_CODE | No | Test Authorization code |
TEST_APP | No | Application.app, like: "built-in" |
TEST_TEST_ORGANIZATION | No | Application.organization, like: "built-in" |
yarn run test
FAQs
Node.js client SDK for Casdoor
The npm package @anxing131/casdoor-nodejs-sdk receives a total of 1 weekly downloads. As such, @anxing131/casdoor-nodejs-sdk popularity was classified as not popular.
We found that @anxing131/casdoor-nodejs-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.