feedback-js

Simple self-hosted feedback modal for any website
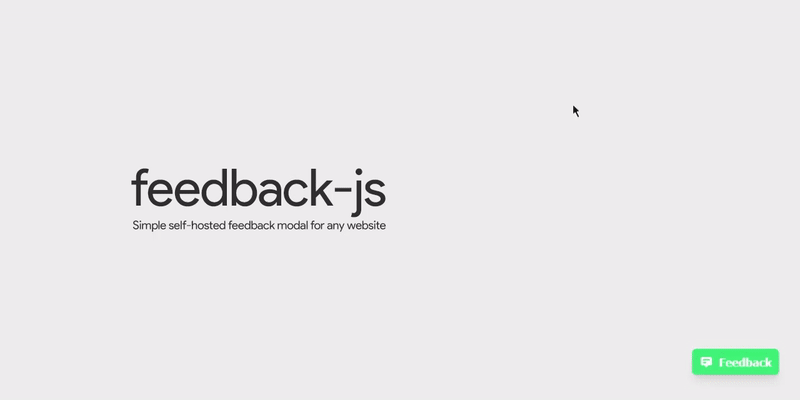
🔮 Live Demo
👋 Introduction
feedback-js lets you add a feedback modal to any website. Just include the script below to your website and run the example Node.js server to handle the form submission.
🚀 Get started
JSDelivr
Add this to your HTML page:
<script src="https://cdn.jsdelivr.net/npm/feedback-js/dist/feedback-js.min.js"></script>
<script>
function addFeedback() {
const options = {
id: 'example',
endpoint: 'https://example.com/feedback'
}
new Feedback(options).attach();
}
window.addEventListener('load', addFeedback);
</script>
NPM
Install feedback-js using NPM
npm install feedback-js
Then add the following JavaScript code:
import Feedback from 'feedback-js';
const options = {
id: 'example',
endpoint: 'https://example.com/feedback'
}
new Feedback(options).attach();
By default feedback-js will add a feedback button to the bottom right corner of your page with the default colors and text. This can be configured using the options object.
📚 Setup
If you include feedback-js via the cdn or as an npm package and run:
const options = {
id: 'example',
endpoint: 'https://example.com/feedback'
}
new Feedback(options).attach();
feedback-js will automatically add the feedback form to your page.
You will have to handle the submission on the backend yourself. feedback-js will make a POST request to your specified endpoint with the following body:
{
"id": "example",
"email": "hello@mxis.ch",
"feedbackType": "issue",
"url": "https://example.com",
"message": "When I click x nothing happens."
}
Node.js example:
const express = require('express')
const app = express()
const port = 3000
app.post('/feedback', async (req, res) => {
const { id, feedbackType, message, email, url } = req.body
console.log(`New ${ feedbackType } feedback for form ${ id } from user ${ email } on page ${ url }: ${ message }`)
res.send('ok')
})
app.listen(port, () => {
console.log(`Listening at http://localhost:${ port }`)
})
🛠️ Manuall usage
If you don't want to show the button and send feedback programatically you can use the method sendFeedback()
:
const feedback = new Feedback(options);
feedback.send('feedbackType', 'message', 'url', 'email');
⚙️ Options
You can customize feedback-js by passing a options object to new Feedback()
:
const options = {
id: 'feedback',
endpoint: 'https://example.com/feedback',
emailField: true,
btnTitle: 'Feedback',
title: 'Company Feedback',
contactText: 'Or send an email!',
contactLink: 'mailto:hello@mxis.ch',
typeMessage: 'What feedback do you have?',
success: 'Thanks! 👊',
failedTitle: 'Oops, an error ocurred!',
failedMessage: 'Please try again. If this keeps happening, try to send an email instead.',
position: 'right',
primary: 'rgb(53, 222, 118)',
background: '#fff',
color: '#000'
}
const feedback = new Feedback(options);
feedback.attach();
💻 Development
Issues and PRs are very welcome!
The actual source code of this library is in the feedback.js
file in the src
folder.
- run
yarn lint
or npm run lint
to run eslint. - run
yarn watch
or npm run watch
to watch for changes and build to the dist
folder. - run
yarn build
or npm run build
to produce a production version of feedback-js in the dist
folder.
❔ About
This library was developed by me (@betahuhn) in my free time. If you want to support me:

Credits
The design of the feedback form was inspired by @kangabru's feedback form on the Panda Snap dashboard.
License
Copyright 2020 Maximilian Schiller
This project is licensed under the MIT License - see the LICENSE file for details