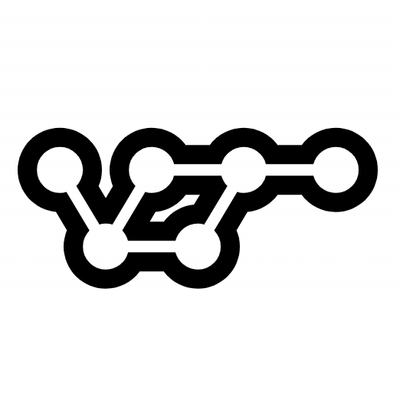
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@betonyou/react-native-unity-play
Advanced tools
React Native package to use Unity as library with React Native app
$ npm install react-native-unity-play --save
UNITY PLAYER SETTINGS
Multitasking
-> Requires Fullscreen
-> no selection set !Status Bar
-> Status Bar Hidden
-> no selection set !BUILD SETTINGS
Export project
-> selection set !UNITY PLAYER SETTINGS
Resolution and Presentation
-> Start in fullscreen mode
-> no selection set !Resolution and Presentation
-> Render outside safe area
-> no selection set !npm
unity
folder at project rootpod install
[project_root]/unity/builds/ios
Unity-iPhone.xcodeproj
to your workspace: Menu
-> File
-> Add Files to [workspace_name]...
-> [project_root]/unity/builds/ios/Unity-iPhone.xcodeproj
UnityFramework.framework
to Frameworks, Libraries, and Embedded Content
:
your_app
target in workspaceGeneral
/ Frameworks, Libraries, and Embedded Content
press +
Unity-iPhone/Products/UnityFramework.framework
Build Phases
remove UnityFramework.framework
from Linked Frameworks and Libraries
( select it and press -
)Build Phases
move Embedded Frameworks
before Compile Sources
( drag and drop )Add following lines to your project main.m
file (located at same folder with AppDelegate
)
#import <UIKit/UIKit.h>
+++ #import <RNUnity/RNUnity.h>
#import "AppDelegate.h"
int main(int argc, char * argv[]) {
@autoreleasepool {
+++ [RNUnity setArgc:argc];
+++ [RNUnity setArgv:argv];
return UIApplicationMain(argc, argv, nil, NSStringFromClass([AppDelegate class]));
}
}
Add following lines to your project AppDelegate.m
file
#import "AppDelegate.h"
#import <React/RCTBridge.h>
#import <React/RCTBundleURLProvider.h>
#import <React/RCTRootView.h>
+++ #import <RNUnity/RNUnity.h>
+++ - (void)applicationWillResignActive:(UIApplication *)application { [[[RNUnity ufw] appController] applicationWillResignActive: application]; }
+++ - (void)applicationDidEnterBackground:(UIApplication *)application { [[[RNUnity ufw] appController] applicationDidEnterBackground: application]; }
+++ - (void)applicationWillEnterForeground:(UIApplication *)application { [[[RNUnity ufw] appController] applicationWillEnterForeground: application]; }
+++ - (void)applicationDidBecomeActive:(UIApplication *)application { [[[RNUnity ufw] appController] applicationDidBecomeActive: application]; }
+++ - (void)applicationWillTerminate:(UIApplication *)application { [[[RNUnity ufw] appController] applicationWillTerminate: application]; }
@end
Create directory into android/app/libs
Copy libs from <project_name>/unity/builds/android/unityLibrary/libs/*
to android/app/libs
Add ndk support into android/app/build.gradle
defaultConfig {
...
ndk {
abiFilters "armeabi-v7a", "arm64-v8a"
}
}
Append the following lines to android/settings.gradle
:
include ':unityLibrary'
project(':unityLibrary').projectDir=new File('..\\unity\\builds\\android\\unityLibrary')
Insert the following lines inside the dependencies block in android/app/build.gradle
:
implementation project(':unityLibrary')
implementation files("${project(':unityLibrary').projectDir}/libs/unity-classes.jar")
Add strings to res/values/strings.xml
<string name="game_view_content_description">Game view</string>
<string name="unity_root">unity_root</string>
Update .MainActivity
into AndroidManifest.xml
<application
...
android:extractNativeLibs="true"
<activity
android:name=".MainActivity"
...
android:configChanges="mcc|mnc|locale|touchscreen|keyboard|keyboardHidden|navigation|orientation|screenLayout|uiMode|screenSize|smallestScreenSize|fontScale|layoutDirection|density"
android:hardwareAccelerated="true"
>
Setup minSdkVersion
greater than or equal to 21
Remove <intent-filter>...</intent-filter>
from <project_name>/unity/builds/android/unityLibrary/src/main/AndroidManifest.xml
at unityLibrary to leave only integrated version.
Add to android/gradle.properties
unityStreamingAssets=.unity3d
Add to build.gradle
allprojects {
repositories {
flatDir {
dirs "$rootDir/app/libs"
}
<project_name>/unity/builds/android/unityLibrary/src/main/AndroidManifest.xml
delete android:icon="@mipmap/app_icon"
and android:theme="@style/UnityThemeSelector"
if they are installed
import { StyleSheet, View, Dimensions, Button, } from 'react-native';
import UnityView, {
UnityModule,
UnityResponderView,
} from 'react-native-unity-play';
const {width, height} = Dimensions.get('window');
const App: () => Node = () => {
const [isVisible, setVisible] = useState(false);
let unityElement
if (Platform.OS === 'android') {
unityElement = (
<UnityView style={{flex: 1}} />
);
} else {
unityElement = (
<UnityResponderView
fullScreen={true}
style={{width: width, height: height}}
/>
);
}
return (
<View>
{!isVisible && (
<Button title={'Start'} onPress={() => setVisible(true)} />
)}
{isVisible && (
<>
{unityElement}
<View
style={{
position: 'absolute',
top: 45,
left: 20,
zIndex: 2,
}}>
<Button
title={'Close'}
onPress={() => {
if (Platform.OS === 'android') {
UnityModule.quit();
}
setVisible(false);
}}
style={{color: '#fff'}}
/>
</View>
</>
)}
</View>
);
};
FAQs
React Native package to use Unity as library with React Native app
We found that @betonyou/react-native-unity-play demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.