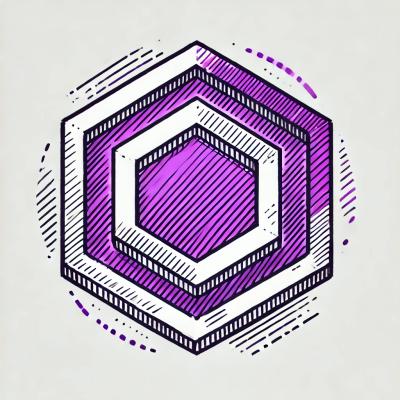
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
npm install --save @cknow/vfg
In the example below will be generated dry fields, that is, without style.
<template>
<vfg :model="model" :schema="schema"></vfg>
</template>
<script>
import vfg from '@cknow/vfg';
Vue.use(vfg);
export default {
data() {
return {
model: {
name: 'Your name',
subject: 'Option 1'
},
schema: [{
// input text is default
label: 'Name',
model: 'name'
},{
type: 'select',
label: 'Subject',
model: 'subject',
items: ['Option 1', 'Option 2', 'Option 3']
},{
type: 'textarea',
label: 'Message',
model: 'message'
}]
}
}
}
</script>
Available fields
...
schema: [
{
type: 'input',
inputType: 'INPUT_TYPE',
class: ['foo', 'bar'],
id: 'fieldID',
enabled: true,
autocomplete: false,
autofocus: false,
disabled: false,
max: 150,
maxlength: 150,
min: 50,
name: 'fieldName',
placeholder: 'Field Placeholder',
readonly: false,
required: true
size: 50,
step: 1,
width: 80,
// custom attrs
attrs: {
'data-foo': 'bar'
},
// events
events: {
click: 'clickHanlder'
}
}
]
....
Input type list:
...
schema: [
{
type: 'select',
items: ['Option 1', 'Option 2', 'Option 3'],
classes: ['foo', 'bar'],
id: 'fieldId',
enabled: true,
autofocus: false,
disabled: false,
multiple: false,
name: 'fieldName',
required: true,
// custom attrs
attrs: {
'data-foo': 'bar'
},
// events
events: {
click: 'clickHanlder'
}
},
// with value and name
{
type: 'select',
items: [{
id: 1, // value
name: 'Option 1' // name
}, {
id: 2, // value
name: 'Option 2' // name
}, {
id: 3, // value
name: 'Option 3' // name
}]
},
// with optgroup
{
type: 'select',
items: [{
name: 'Option 1',
options: [
'Option 1-1',
'Option 1-2',
'Option 1-3'
]
}, {
name: 'Option 2',
options: [
'Option 2-1',
'Option 2-2',
'Option 2-3'
]
}]
}
]
....
...
schema: [
{
type: 'textarea',
classes: ['foo', 'bar'],
id: 'fieldId',
enabled: true,
autofocus: false,
cols: 80,
disabled: false,
maxlength: 500,
name: 'fieldName',
placeholder: 'Field Placeholder',
readonly: false,
required: true,
rows: 5,
// custom attrs
attrs: {
'data-foo': 'bar'
},
// events
events: {
click: 'clickHanlder'
}
}
]
....
The big difference in this package is that we can use themes in a very simple way.
But do not worry, we already have the most well-known themes developed:
In the example below the fields will be generated with the style of Bootstrap:
Note: Do not forget to add the style sheets of theme to the header of the page.
npm install --save @cknow/vfg-theme-bootstrap
<template>
<vfg :options="options" :model="model" :schema="schema"></vfg>
</template>
<script>
import vfg from '@cknow/vfg';
import vfgThemeBootstrap from '@cknow/vfg-theme-bootstrap';
Vue.use(vfg);
Vue.use(vfgThemeBootstrap); // register bootstrap theme
export default {
data() {
return {
options: {
theme: 'bootstrap' // set theme
},
model: {
...
},
schema: [
...
]
}
}
}
</script>
You can use a global theme, as in the example below:
import vfg from '@cknow/vfg';
Vue.use(vfg, {
theme: 'THEME_NAME'
});
You can also mix themes and styles if necessary. By placing the theme option in the field you want, you will see in example below:
Note: Do not forget to add the style sheets of each theme to the header of the page.
npm install --save @cknow/vfg-theme-bootstrap
npm install --save @cknow/vfg-theme-bulma
<template>
<vfg :options="options" :schema="schema"></vfg>
</template>
<script>
import vfg from '@cknow/vfg';
import vfgThemeBootstrap from '@cknow/vfg-theme-bootstrap';
import vfgThemeBulma from '@cknow/vfg-theme-bulma';
Vue.use(vfg);
Vue.use(vfgThemeBootstrap); // register bootstrap theme
Vue.use(vfgThemeBulma); // register bulma theme
export default {
data() {
return {
options: {
// default theme is Bootstrap
theme: 'bootstrap'
},
schema: [{
label: 'Name with Bootstrap'
},{
theme: 'bulma' // set Bulma theme
label: 'Name with Bulma'
}]
}
}
}
</script>
FAQs
A schema-based form generator component for Vue.js
We found that @cknow/vfg demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.