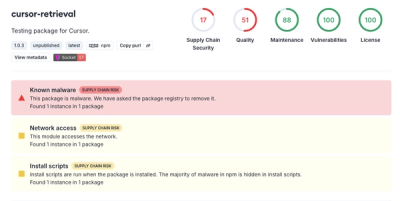
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@graphql-inspector/commands
Advanced tools
Plugin system for commands in GraphQL Inspector
@graphql-inspector/commands is a toolset for inspecting, validating, and comparing GraphQL schemas. It helps developers ensure the integrity and compatibility of their GraphQL APIs by providing various commands to check for changes, validate schemas, and more.
Schema Diff
The `diff` command allows you to compare two GraphQL schemas and identify the differences between them. This is useful for understanding what has changed between schema versions.
const { diff } = require('@graphql-inspector/commands');
const oldSchema = `type Query { hello: String }`;
const newSchema = `type Query { hello: String, goodbye: String }`;
const result = diff(oldSchema, newSchema);
console.log(result);
Schema Validation
The `validate` command checks a GraphQL schema for errors and inconsistencies. This helps ensure that the schema is correctly defined and adheres to GraphQL specifications.
const { validate } = require('@graphql-inspector/commands');
const schema = `type Query { hello: String }`;
const result = validate(schema);
console.log(result);
Schema Coverage
The `coverage` command analyzes how well your GraphQL queries cover your schema. This helps identify parts of the schema that are not being used or tested.
const { coverage } = require('@graphql-inspector/commands');
const schema = `type Query { hello: String }`;
const documents = [{ query: `{ hello }` }];
const result = coverage(schema, documents);
console.log(result);
graphql-cli is a command-line tool that provides various utilities for working with GraphQL schemas and queries. It offers functionalities like schema validation, introspection, and code generation. Compared to @graphql-inspector/commands, graphql-cli is more focused on providing a broad set of tools for different aspects of GraphQL development.
graphql-schema-linter is a tool for linting GraphQL schemas. It helps enforce best practices and coding standards by checking the schema against a set of rules. While @graphql-inspector/commands offers schema validation as one of its features, graphql-schema-linter is dedicated solely to linting and enforcing schema quality.
Apollo provides a suite of tools for building, managing, and monitoring GraphQL APIs. It includes features like schema validation, performance monitoring, and client-server communication. Apollo is a more comprehensive solution compared to @graphql-inspector/commands, which is focused specifically on schema inspection and validation.
FAQs
Plugin system for commands in GraphQL Inspector
We found that @graphql-inspector/commands demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.