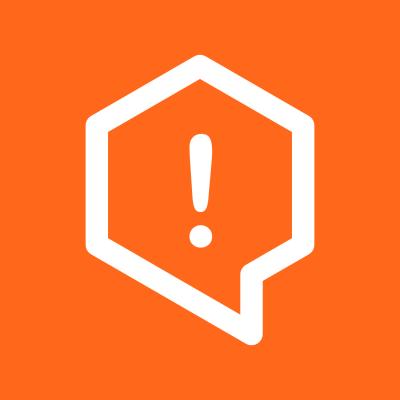
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@jet-pie/react-native
Advanced tools
This package implements the PIE design system for React Native.
This package implements the PIE design system for React Native.
Via package managers, install PIE React Native
and its dependencies:
yarn add @jet-pie/react-native @jet-pie/theme styled-components polished
// if it is a TypeScript application
yarn add -D @types/styled-components
Copy the fonts inside of fonts
folder to android/app/src/main/assets/font
folder of your project.
Drag and drop the fonts inside of fonts
folder onto your project in Xcode.
After that, add these entries to Info.plist
:
<key>UIAppFonts</key>
<array>
<string>JETSansDigital-Bold.ttf</string>
<string>JETSansDigital-BoldItalic.ttf</string>
<string>JETSansDigital-ExtraBold.ttf</string>
<string>JETSansDigital-ExtraBoldItalic.ttf</string>
<string>JETSansDigital-Italic.ttf</string>
<string>JETSansDigital-Regular.ttf</string>
</array>
Also, make sure to add these fonts to Copy Bundle Resources
in Build Phases
tab of your app in Xcode.
Our components need specific colors, fonts and styles, which are provided via styled-components
’s ThemeProvider
API. The PIEThemeProvider
component takes care of providing the theme.
To use it, add this component to the top of you component tree, wrapping the rest of the application as children
components. It also allows you to consume the Theme in your application using styled-components
's template functions. All tokens values will be accessible via the theme property.
NOTE: Make sure every @pie/react-native
component and your components that consume the theme to be wrapped by the PIEThemeProvider
.
TYPING YOUR THEME PROVIDER
To have full TypeScript support on your theme, follow the next steps:
styled.d.ts
and make sure it is included by your tsconfig.json
import 'styled-components/native';
import { ThemeWithMode } from '@jet-pie/react-native';
declare module 'styled-components' {
export interface DefaultTheme extends ThemeWithMode {}
}
When using a color make sure to use the alias token for it instead of hardcodding the color value,
you can use them by accesing the themeAlias
property from the theme.
export const Wrapper = styled.View`
background-color: ${({ theme }) => theme.themeAlias.containerDefault};
`;
We have a PIEText
component that already has the font definitions (font-family, spacing, and size).
So instead of using Text
component from React Native use the PIEText
.
import { PIEText } from '@jet-pie/react-native';
export const TextExample = () => (
<View>
<PIEText fontSize="size20">Regular Text</PIEText>
<PIEText.Bold>Bold Text</PIEText.Bold>
</View>
);
On PIE have 11 spacing variants, that goes from s00
(0px) to s10
(80px).
We have an API called getSpacing
that allows you to get those values from the theme
import { getSpacing } from '@jet-pie/react-native';
export const Wrapper = styled.View`
padding: ${getSpacing('s05')}; //padding: 24px;
width: 100%;
`;
You can also concat up to 4 values for better customization of your spacing values:
export const Wrapper = styled.View`
padding: ${getSpacing(
's04',
's02',
's02',
's05'
)}; //padding: 16px 8px 8px 24px;
margin: ${getSpacing('s05', 's00')}; //margin: 24px 0px;
width: 100%;
`;
We also have an API called getElevation
to make it easier to use the elevation/shadow on iOS and Android devices,
it has 5 elevations levels from e1
to e5
and it returns an style object.
import { getElevation } from '@jet-pie/react-native';
export const DefaultCard = css`
background: ${({ theme }) => theme.themeAlias.containerDefault};
${getElevation('e1')}
`;
yarn install
from the project's root folder to do it so.yarn build:packages
from the root folder to run a build for each of them.In order to develop new components and to manually test PRs we have an example app that runs a storybook with our components. It can be found in the examples/react-native-pie-example folder.
To run it go to the react-native-pie-example
and run yarn ios:setup
, and yarn ios
or yarn android
to run each version.
To create a new component and make it available in the storybook folder.
Create your new component in the packages/react-native
project, make sure to export it in the src/index.ts
file.
Go to the examples/react-native-pie-example
project and create a new story for it inside the storybook/stories
folder. Eg: YourComponentName.stories.tsx
.
In the index file inside the storybook/stories
folder, import you newly created file.
import './YourComponentName.stories';
Reload the running app and a new story for it should be available on the left panel.
Make sure the component is available in the Example App and bump the library version.
For version tracking, we use Semantic Versioning to bump versions on our package. Until the writing of this document, this process in entirely manual. So when creating a new version of the PIE React-Native package:
package.json
based on Semantic VersioningTo have a component added and merged into the production build we need to follow some PR guidelines:
PIE React Native
andThis way we can ensure the transparency of our design system and a clear communication with the teams that consume it.
FAQs
This package implements the PIE design system for React Native.
The npm package @jet-pie/react-native receives a total of 21 weekly downloads. As such, @jet-pie/react-native popularity was classified as not popular.
We found that @jet-pie/react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.