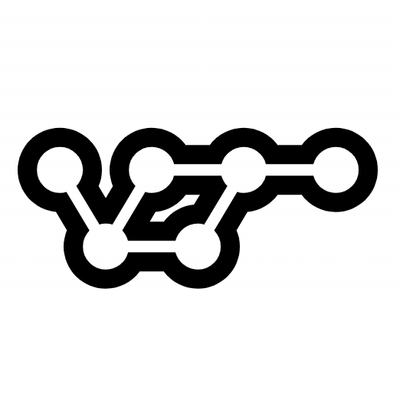
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@keg-hub/re-theme
Advanced tools
Tools for styling React or React Native components
"re-theme": "git+https://github.com/keg-hub/re-theme"
ReThemeProvider
, e.g.:import { ReThemeProvider } from 're-theme'
const myCustomTheme = {
// theme styles go here
}
export const App from Component {
state = { theme: myCustomTheme }
componentDidUpdate = () => {
this.props.theme !== this.state.theme &&
this.setState({ theme: this.props.theme })
}
render(){
return (
<ReThemeProvider theme={this.state.theme} merge={false}>
<App />
</ReThemeProvider>
)
}
}
ReThemeProvider
accepts two props
theme
: the theme object, containing any global styles you want to use across your appmerge
: a boolean indicating whether or not you want to merge the passed in theme prop with the current themereStyle
is the preferred utility in ReTheme for styling your components ...
import { reStyle } from '@keg-hub/re-theme/reStyle'
// basic styling with an object
const StyledButton = reStyle(Button)({
position: 'absolute'
})
// using global theme
const StyledButton = reStyle(Button)(theme => ({
// reStyle also supports style aliases. View these aliases in src/constants/ruleHelpers.js
pos: 'absolute',
c: theme.colors.red
}))
// using theme and props
const StyledButton = reStyle(Button)((theme, props) => ({
position: 'absolute',
color: theme.colors.red
borderColor: props.outline || theme.colors.borderColor
})
// for styling components with any props, set the default props
const StyledIcon = reStyle(SomeSvgIcon)(
{ position: 'absolute' }
theme => ({
// these are default props passed to `SomeSvgIcon`, not style attributes
width: 32,
height: 32
className: 'some-class',
customProp: theme.customValue
})
)
/**
* For specifying a specific prop for styles, pass in a string for the 2nd argument.
* This is equivalent to:
* const styles = {
* main: { position: 'absolute' },
* content: { margin: 32 }
* }
* <Container styles={styles}>
*/
const StyledContainer = reStyle(Container, 'styles')({
main: {
position: 'absolute'
},
content: {
margin: 32
}
})
Checkout the Example App for documentation.
FAQs
Simple Theme switcher / builder for React and React Native
The npm package @keg-hub/re-theme receives a total of 1 weekly downloads. As such, @keg-hub/re-theme popularity was classified as not popular.
We found that @keg-hub/re-theme demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.