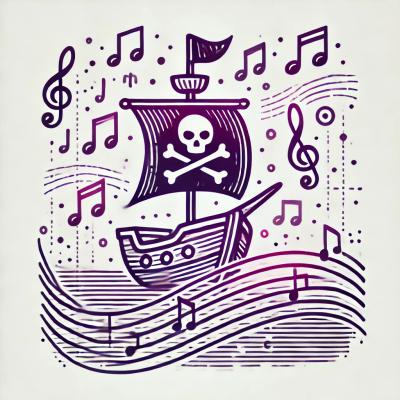
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
@nativescript/apple-pay
Advanced tools
Plugin that provides Apple Pay support for NativeScript iOS applications.
ns plugin add @nativescript/apple-pay
In order for Apple Pay to work in your iOS application you will need to complete the following steps. These steps are also outlined in the Apple PassKit documentation.
Create a merchant ID.
Create a Payment Processing certificate.
Enable Apple Pay in Xcode.
Follow the instructions in Configure Apple Pay (iOS, watchOS), which guide you to create the following:
Merchant ID. An identifier you register with Apple that uniquely identifies your business as a merchant able to accept payments. This ID never expires, and can be used in multiple websites and iOS apps. See Create a merchant identifier for the setup steps.
Payment Processing Certificate. A certificate associated with your merchant ID, used to secure transaction data. Apple Pay servers use the certificate’s public key to encrypt payment data. You (or your payment service provider) use the private key to decrypt the data to process payments. See Create a payment processing certificate for the setup steps.
Finally, you need to enable the Apple Pay capability in your Xcode project. See Enable Apple Pay for the setup steps.
For a video showing the configuration steps, see: Configuring Your Developer Account for Apple Pay.
Once that configuration is done for your Apple developer account, you will be able to use the PassKit framework for Apple Pay inside your iOS application.
<ios>
<ApplePayBtn
tap="onApplePayTap"
buttonType="InStore"
></ApplePayBtn>
</ios>
import { ApplePayBtn, ApplePayContactFields, ApplePayEvents, ApplePayItems, ApplePayMerchantCapability, ApplePayNetworks, ApplePayPaymentItemType, ApplePayRequest, ApplePayTransactionStatus, AuthorizePaymentEventData } from '@nativescript/apple-pay';
export function onApplePayTap() {
try {
// just ensuring this runs only on iOS
if (global.isIOS) {
const applePayBtn = args.object as ApplePayBtn;
// setup the event listeners for the delegate for apple pay for our button
applePayBtn.once(ApplePayEvents.DidAuthorizePaymentHandler, async (args: AuthorizePaymentEventData) => {
console.log(ApplePayEvents.DidAuthorizePaymentHandler);
// this is where you do backend processing with your payment provider (Stripe, PayPal, etc.)
// this payload is just a sample, your payload to a provider will likely be different
// you can see here how to access the encrypted values from Apple Pay inside the `args.data.paymentData`
const payloadToBackend = {
transaction_type: 'purchase',
merchant_ref: args.data.paymentData.header.transactionId,
method: '3DS',
'3DS': {
merchantIdentifier: <SomeIdentifierFromPaymentProvider>,
data: args.data.paymentData.data,
signature: args.data.paymentData.signature,
version: args.data.paymentData.version,
header: args.data.paymentData.header
}
};
const result = await someHttpMethodToYourProviderBackend(payloadToBackend);
if (result && result.value === true) {
// need this to call when the payment is successful to close the payment sheet correctly on iOS
args.completion(ApplePayTransactionStatus.Success);
// now you can follow through with your user flow since the transactin has been successful with your provider
} else {
// payment failed on the backend, so show the FAILURE to close the Apple Pay sheet
args.completion(ApplePayTransactionStatus.Failure);
}
});
// these are the items your customer is paying for
const paymentItems = [
{
amount: 20.50,
label: 'Balance',
type: ApplePayPaymentItemType.Final,
},
] as ApplePayItems[];
const request = {
paymentItems,
merchantId: <Your_Apple_Pay_Merchant_ID>, // the merchant ID for this app
merchantCapabilities: ApplePayMerchantCapability.ThreeDS,
countryCode: 'US',
currencyCode: 'USD',
shippingContactFields: [ApplePayContactFields.Name, ApplePayContactFields.PostalAddress],
billingContactFields: [ApplePayContactFields.Name, ApplePayContactFields.PostalAddress],
supportedNetworks: [ApplePayNetworks.Amex, ApplePayNetworks.Visa, ApplePayNetworks.Discover, ApplePayNetworks.MasterCard],
} as ApplePayRequest;
// `createPaymentRequest` will call into the Apple Pay SDK and present the user with the payment sheet for the configuration provided
await applePayBtn.createPaymentRequest(request).catch((err) => {
console.log('Apple Pay Error', err);
});
}
} catch (error) {
console.log(error);
}
}
Name | Description |
---|---|
createPaymentRequest(request: ApplePayRequest) | Creates the Apple Pay payment request and presents the user with the payment sheet. |
Key | Description |
---|---|
DidAuthorizePaymentHandler | Tells the delegate that the user has authorized the payment request and asks for a result. |
AuthorizationDidFinish | Tells the delegate that payment authorization has completed. |
Key | Description |
---|---|
EmailAddress | Indicates an email address field. |
Name | Indicates a name field. |
PhoneNumber | Indicates a phone number field. |
PhoneticName | Indicates a phonetic name field. |
PostalAddress | Indicates a postal address field. |
Key |
---|
Amex |
CarteBancaire |
CarteBancaires |
ChinaUnionPay |
Discover |
Eftpos |
Electron |
Elo |
IDCredit |
Interac |
Jcb |
Mada |
Maestro |
MasterCard |
PrivateLabel |
QuicPay |
Suica |
Visa |
VPay |
Key | Value |
---|---|
ThreeDS | PKMerchantCapability.Capability3DS |
EMV | PKMerchantCapability.CapabilityEMV |
Credit | PKMerchantCapability.CapabilityCredit |
Debit | PKMerchantCapability.CapabilityDebit |
Key | Value |
---|---|
Success | PKPaymentAuthorizationStatus.Success |
Failure | PKPaymentAuthorizationStatus.Failure |
InvalidBillingPostalAddress | PKPaymentAuthorizationStatus.InvalidBillingPostalAddress |
InvalidShippingPostalAddress | PKPaymentAuthorizationStatus.InvalidShippingPostalAddress |
InvalidShippingContact | PKPaymentAuthorizationStatus.InvalidShippingContact |
PINRequired | PKPaymentAuthorizationStatus.PINRequired |
PINIncorrect | PKPaymentAuthorizationStatus.PINIncorrect |
PINLockout | PKPaymentAuthorizationStatus.PINLockout, |
Key | Value |
---|---|
Final | PKPaymentSummaryItemType.Final |
Pending | PKPaymentSummaryItemType.Pending |
Key | Value |
---|---|
Plain | PKPaymentButtonType.Plain |
Buy | PKPaymentButtonType.Buy |
Book | PKPaymentButtonType.Book |
Checkout | PKPaymentButtonType.Checkout |
Donate | PKPaymentButtonType.Donate |
InStore | PKPaymentButtonType.Book |
Subscribe | PKPaymentButtonType.Subscribe |
Key | Value |
---|---|
White | PKPaymentButtonStyle.White |
WhiteOutline | PKPaymentButtonStyle.WhiteOutline |
Black | PKPaymentButtonStyle.Black |
interface ApplePayRequest {
paymentItems: Array<ApplePayItems>;
merchantId: string; // the merchant ID for this app
merchantCapabilities: number;
countryCode: string;
currencyCode: string;
supportedNetworks: Array<ApplePayNetworks>;
billingContactFields?: Array<ApplePayContactFields>;
shippingContactFields?: Array<ApplePayContactFields>;
shippingMethods?: Array<ApplePayShippingMethods>;
}
interface ApplePayItems {
label: string;
amount: number;
type: ApplePayPaymentItemType;
}
interface AuthorizePaymentEventData extends EventData {
eventName: string;
object: any;
data?: {
payment: PKPayment;
token: PKPaymentToken;
paymentData: ApplePayPaymentData;
billingAddress;
billingContact: PKContact;
shippingAddress;
shippingContact: PKContact;
shippingMethod: PKShippingMethod;
};
completion: (status: ApplePayTransactionStatus) => void;
}
interface AuthorizationDidFinishEventData extends EventData {
eventName: string;
object: any;
}
interface ApplePayPaymentData {
/**
* Encrypted payment data.
*/
data;
/**
* Additional version-dependent information used to decrypt and verify the payment.
*/
header;
/**
* Signature of the payment and header data. The signature includes the signing certificate, its intermediate CA certificate, and information about the signing algorithm.
*/
signature;
/**
* Version information about the payment token.
* The token uses EC_v1 for ECC-encrypted data, and RSA_v1 for RSA-encrypted data.
*/
version: string;
}
Apache License Version 2.0
FAQs
Plugin that provides Apple Pay support for NativeScript iOS applications.
The npm package @nativescript/apple-pay receives a total of 2 weekly downloads. As such, @nativescript/apple-pay popularity was classified as not popular.
We found that @nativescript/apple-pay demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 19 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.