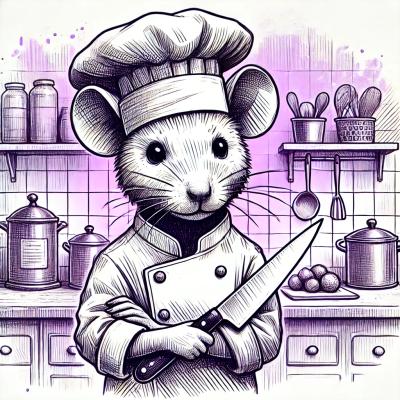
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@notabene/pii-sdk
Advanced tools
Encryption & Decryption Library for Personal Identifiable Information (PII)
Documentation • Getting started • Installation • Configuration
yarn
yarn add @notabene/pii-sdk
npm
npm install @notabene/pii-sdk
Parameter | Description |
---|---|
NOTABENE_URL | Notabene directory URL to get VASP DIDs and keys |
NOTABENE_PII_URL | Default Notabene PII escrow service to share encrypted audited data |
import PIIsdk from '@notabene/pii-sdk';
//Init Notabene Key Toolset
const toolset = new PIIsdk({
NOTABENE_URL: 'https://api.notabene.id',
NOTABENE_PII_URL: 'https://pii.notabene.id',
});
//Create cryptography keypair
const myKey = await toolset.createKey();
//Encrypt to VASP with key did:key:z6MkhaX...
const encryptedPII = await toolset.encryptPII({
senderDIDKey: myKey.did,
recipientDIDkeys: ['did:key:z6MkhaX...'],
body: piiData,
keypair: myKey,
});
//Decrypt PII for VASP
const decryptedPII = await toolset.decryptPII({encryptedMessage: encryptedPII, keypair: myKey});
IVMS objects are complex nested JSON objects, to encrypt its data we first flatten the IVMS structure to a key-value pair. Where the key is the path to the value in the JSON structure.
// the IVMS object:
const pii = {
originator: {
originatorPersons: [
{
naturalPerson: {
name: [
{
nameIdentifier: [
{
primaryIdentifier: 'Frodo',
secondaryIdentifier: 'Baggins',
nameIdentifierType: 'LEGL',
},
],
},
],
},
},
],
},
};
// flatten the IVMS object
const flatPII = toolset.flattenPII(pii);
console.log(flatPII);
/*
[
{
key: 'originator.originatorPersons[0].naturalPerson.name[0].nameIdentifier[0].primaryIdentifier',
value: 'Frodo'
},
{
key: 'originator.originatorPersons[0].naturalPerson.name[0].nameIdentifier[0].secondaryIdentifier',
value: 'Baggins'
},
{
key: 'originator.originatorPersons[0].naturalPerson.name[0].nameIdentifier[0].nameIdentifierType',
value: 'LEGL'
}
]
*/
// import or create a new DIDKey keypair
const myKey = getMyPrivateDIDKey() || await toolset.createKey();
// encrypting the PII (IVMS) values
for (const obj of flatPII) {
obj.value = await toolset.encryptPII({
senderDIDKey: myKey.did,
recipientDIDkeys: [], // DIDKeys (public keys) of other recipients
body: obj.value,
keypair: myKey
});
}
// ... sending this array of KeyValue pairs to the PII Service API
// The PII (IVMS) object with encrypted values
const encryptedIVMS = {
originator: {
originatorPersons: [
{
naturalPerson: {
name: [
{
nameIdentifier: [
{
primaryIdentifier:
'{"protected":"eyJ0eXAiOiJhcHBsaWNhdGlvbi9kaWRjb21tLWVuY3J5cHRlZCtqc29uIiwic2tpZCI6ImRpZDprZXk6ejZNa3VDQXRWclIxU3hLVGtxQnhSMUJ0VDd2MWJ5aUJ0bVkxNFd0b2t4Q0U2aW52I3o2TFNkM3NObWdrV3hwdFBXVGU4ZGdMQ3ZXMVRqRlcyYWlwRnFMbUxUREJQZ3VkYSIsImVuYyI6IlhDMjBQIn0","iv":"XgtpNKM7_hRovx4Kwk5I6c3q5ocrRYXb","ciphertext":"L7_fQrZfQF2dCkjXdHnGM_jdq2dV1IGNzAgyjISg_pPS5pVV6Fi81kfZdbtu_XSgV4h085ZNQ-0iPXYiYYvDLkZcDJpYQrl88Raa48Mw1okSWQR7auH3VF6FeV5ugDnBLBoSjStbLFj2HEVwKtc9vrSjVr2iWLSs9OVCfcDtKV3Q9RJZVEY-higlpm1b7b6pS8-bwJ7HbcRS0hW_8NNkXPn4846jKrB28sDnfrQ_9X62wMP0Em_b86FT9dud78SGjIharesdT8HQPo-ypzLqKeIR7mnt4eLtPGUfc8f6HeA","tag":"FafOxzJmdBPABAC49LdCFw","recipients":[{"encrypted_key":"H76hGHBmkxOgwUuPwQzx6o1LOv6B0HhINhWj9ESqOFs","header":{"alg":"ECDH-1PU+XC20PKW","iv":"yENsMskcLxwPz4mUGMVlTGPI7liaerRT","tag":"IdCE7h6HvQdYQNlVPbvl0w","epk":{"kty":"OKP","crv":"X25519","x":"PgXPvaJ2BcnooB5B-76p0z47IQyEIIesDZVcTagBxTk"},"kid":"did:key:z6MkuCAtVrR1SxKTkqBxR1BtT7v1byiBtmY14WtokxCE6inv#z6LSd3sNmgkWxptPWTe8dgLCvW1TjFW2aipFqLmLTDBPguda"}}]}',
secondaryIdentifier:
'{"protected":"eyJ0eXAiOiJhcHBsaWNhdGlvbi9kaWRjb21tLWVuY3J5cHRlZCtqc29uIiwic2tpZCI6ImRpZDprZXk6ejZNa3VDQXRWclIxU3hLVGtxQnhSMUJ0VDd2MWJ5aUJ0bVkxNFd0b2t4Q0U2aW52I3o2TFNkM3NObWdrV3hwdFBXVGU4ZGdMQ3ZXMVRqRlcyYWlwRnFMbUxUREJQZ3VkYSIsImVuYyI6IlhDMjBQIn0","iv":"9vpvSjvn1A7DolkoMB18jfsODtZkmbVC","ciphertext":"Y6R8GMR-_4nQpCYUxxPj4oUGDW3AvWMU2Nutw-ZC0cdtvPyTVqXK4hjVtKSws_DqqrALrAW-YB7g2j_LoOC32-qkU90eNVsnZhn7CWDZk6BQaDfJL1gG37p0PLOuOJJajeUXtneILO2G02ytYcLB74DIXXYITI32TkxednFWLX3JgMXldRtCOLqWqJbZwKZHW9HdbTd3TD0MIOtDCUX82Xmqh-VT_5l0xqFUf-OifiF-ut5PN6ws8eOJX_ortdReWx6frlH4BFHzsg3nhUbrjSSYPbt9Aj7YnP03aE9o8mHqqw","tag":"VpNFV5SSjh4lhCyze1yCWg","recipients":[{"encrypted_key":"ACrfRGnadMVw2Wh_IONGOaWvg-hYkJ7goh4fnmBASzo","header":{"alg":"ECDH-1PU+XC20PKW","iv":"msGsdcbjUrChT25PHU25mYcXYaIZLGnX","tag":"n6K5RRtfUFHTWOT8dAeb_Q","epk":{"kty":"OKP","crv":"X25519","x":"kFIlnnroGJAEmjbXX5ZVpgm5xDwMyI3hAhZRWbyrNTI"},"kid":"did:key:z6MkuCAtVrR1SxKTkqBxR1BtT7v1byiBtmY14WtokxCE6inv#z6LSd3sNmgkWxptPWTe8dgLCvW1TjFW2aipFqLmLTDBPguda"}}]}',
nameIdentifierType:
'{"protected":"eyJ0eXAiOiJhcHBsaWNhdGlvbi9kaWRjb21tLWVuY3J5cHRlZCtqc29uIiwic2tpZCI6ImRpZDprZXk6ejZNa3VDQXRWclIxU3hLVGtxQnhSMUJ0VDd2MWJ5aUJ0bVkxNFd0b2t4Q0U2aW52I3o2TFNkM3NObWdrV3hwdFBXVGU4ZGdMQ3ZXMVRqRlcyYWlwRnFMbUxUREJQZ3VkYSIsImVuYyI6IlhDMjBQIn0","iv":"S0Zvv6KjUnETSkyEIJg5gIounC-QByrr","ciphertext":"PTI9E-yCLvZvy8HC-LRSeBc34XDSAmBQhjrvnJ9pli1i6Bcn29ei_jKNNPH9Rkit6PirwDSQDV2Ydn87GqTIi1WgfQM0uZQ9z1y1s2vEDE6Cy3NRe5qmdfC9VUAz57o8n81u5ReW2-NbywNTCwuteaxEFC3ikmrqtUuJLNA0ZcY0QaX0RWHluJR2TvAcydR8ZiMkFycDa4ChLXIsUAwOcQfOLPoICEjn4ebqpe-MPS0kC27FgdxC5L7Xn7yc3szbm419bQuoeOWWj-C1fnRWV7Kcryi8LnowjDSPiupPLA","tag":"8OlyFnEW6lomzRMsxnwUtw","recipients":[{"encrypted_key":"pF5T6K3O_xM0GTZK_AhBADNYAglDk4KyRyMtMCDUsSE","header":{"alg":"ECDH-1PU+XC20PKW","iv":"dDoBimtXAA0J_Z71KraB1cPZWcUxMDyN","tag":"D_Yzl2Ubi3VgdPZqikCnDg","epk":{"kty":"OKP","crv":"X25519","x":"X-5sm5nr-59EyepvvstkeCABemRo9tl7CPNrF5kNThE"},"kid":"did:key:z6MkuCAtVrR1SxKTkqBxR1BtT7v1byiBtmY14WtokxCE6inv#z6LSd3sNmgkWxptPWTe8dgLCvW1TjFW2aipFqLmLTDBPguda"}}]}',
},
],
},
],
},
},
],
},
};
// flattening the encrypted IVMS object
const flatEncryptedIVMS = toolset.unflattenPII(encryptedIVMS);
// decrypting the values
for (const obj of flatEncryptedIVMS) {
obj.value = await toolset.decryptPII({ encryptedMessage: obj.value, keypair: myKey });
}
console.log(toolset.unflattenPII(flatPII));
/*
{
originator: {
originatorPersons: [
{
naturalPerson: {
name: [
{
nameIdentifier: [
{
primaryIdentifier: 'Frodo',
secondaryIdentifier: 'Baggins',
nameIdentifierType: 'LEGL'
}
]
}
]
}
}
]
}
}
*/
Contributions are welcome!
Want to file a bug, request a feature or contribute some code?
yarn
- To install dependenciesyarn dlx @yarnpkg/sdks vscode
- Sets up VSCode to support ESLint/Prettier/TypeScript using Yarn 3 (make sure to install ZipFS
vscode extension too)yarn watch
- Hot reloading for developmentyarn build
- Compile the library to the dist/
folderyarn lint
- Lints the library using ESLint/Prettieryarn test
- Run unit testsyarn release
- Release the project using semantic-release (only run in CI)MIT © Notabene
FAQs
SDK to manage PII encryption
We found that @notabene/pii-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.