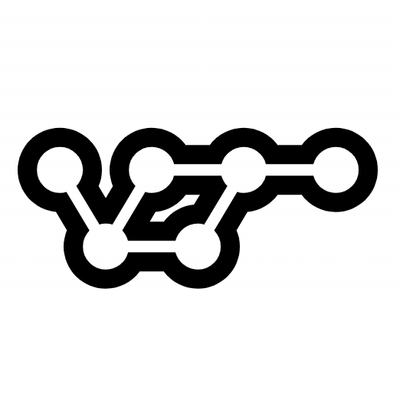
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@oncordjs/client
Advanced tools
OnCord is a simple and intuitive framework built on top of discord.js, designed to simplify your Discord bot development.
OnCord is a simple and intuitive framework built on top of discord.js, designed to simplify your Discord bot development.
Install the package via npm:
npm install @oncordjs/client
We've simplified bot creation with a single command. Follow these steps to install:
Install the CLI globally:
npm install -g @oncordjs/generate
Create a new project:
create-oncord-project my-project-name
This will generate a new directory called my-project-name
with the necessary files and structure for your Oncord bot.
cd my-project-name
Your project is now set up and ready for development. Follow the steps in the documentation to start building your Discord bot with @oncordjs/client
.
The recommended project structure is as follows:
my-bot/
├── src/
│ ├── index.ts
│ ├── commands/
│ │ └── util/
│ │ └── ping.ts
├── tsconfig.json
└── package.json
my-bot/src/index.js
const { Gateway } = require('@oncordjs/client')
const client = new Gateway({
token: "YOUR_BOT_TOKEN",
intents: ['Guilds']
});
client.handleCommands(__dirname+"/commands", ".js")
client.login()
module.exports = {
data: {
name: "ping",
description: 'Replies with Pong!'
},
execute: async (interaction) => {
interaction.reply("Pong!")
}
}
my-bot/src/index.ts
import { Gateway } from '@oncordjs/client';
const client = new Gateway({
token: "YOUR_BOT_TOKEN",
intents: ['Guilds']
});
client.handleCommands(__dirname + "/commands", ".ts"); // <Gateway>.handleCommands(folderPath, fileExtension);
client.login();
my-bot/src/commands/util/ping.ts
import { CommandType } from '@oncordjs/client';
import { CommandInteraction } from 'discord.js';
const command: CommandType = {
data: {
name: 'ping',
description: 'Replies with Pong!'
},
execute: async (interaction: CommandInteraction) => {
await interaction.reply('Pong!');
}
};
export default command;
Contributions are welcome! If you have any questions or want to contribute, you can find the project on GitHub. Feel free to open issues or submit pull requests.
Thank you for using OnCord! We still have a lot more to add, so stay tuned for the upcoming updates!
FAQs
OnCord is a simple and intuitive framework built on top of discord.js, designed to simplify your Discord bot development.
The npm package @oncordjs/client receives a total of 2 weekly downloads. As such, @oncordjs/client popularity was classified as not popular.
We found that @oncordjs/client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.