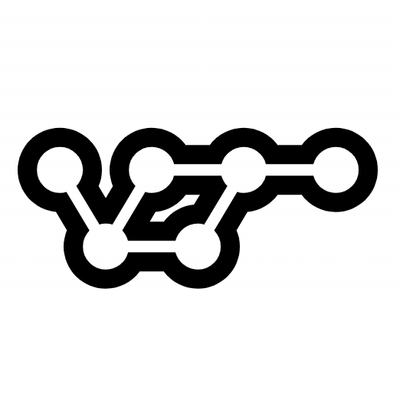
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@oncordjs/generate
Advanced tools
A CLI tool to generate new projects using @oncordjs/client
, inspired by create-next-app
.
You can install the CLI globally using npm:
npm install -g @oncordjs/generate
To create a new Oncord project, run:
create-oncord-app <project-name>
Replace <project-name>
with the name of your new project. This will create a directory with the specified name, containing a basic project structure using @oncordjs/client
.
create-oncord-app my-new-oncord-project
This will generate the following structure:
my-new-oncord-project/
├── package.json
├── tsconfig.json
├── src/
│ ├── index.ts
│ └── commands/
│ └── util/
│ └── ping.ts
└── ...
You can pass additional options to customize your project:
create-oncord-app <project-name> [options]
For example, you can specify a template:
create-oncord-app my-new-oncord-project --template typescript
The recommended project structure for Oncord is as follows:
my-bot/
├── src/
│ ├── index.ts
│ ├── commands/
│ │ └── util/
│ │ └── ping.ts
├── tsconfig.json
└── package.json
my-bot/src/index.js
const { Gateway } = require('@oncordjs/client')
const client = new Gateway("YOUR_BOT_TOKEN", {
intents: ['Guilds']
});
client.handleCommands(__dirname + "/commands", ".js");
client.login();
module.exports = {
data: {
name: "ping",
description: 'Replies with Pong!'
},
execute: async (interaction) => {
interaction.reply("Pong!");
}
}
my-bot/src/index.ts
import { Gateway } from '@oncordjs/client';
const client = new Gateway("YOUR_BOT_TOKEN", {
intents: ['Guilds']
});
client.handleCommands(__dirname + "/commands", ".ts"); // <Gateway>.handleCommands(folderPath, fileExtension);
client.login();
my-bot/src/commands/util/ping.ts
import { CommandType } from '@oncordjs/client';
import { CommandInteraction } from 'discord.js';
const command: CommandType = {
data: {
name: 'ping',
description: 'Replies with Pong!'
},
execute: async (interaction: CommandInteraction) => {
await interaction.reply('Pong!');
}
};
export default command;
To work on the CLI tool itself, follow these steps:
git clone https://github.com/yourusername/create-oncord-app.git
cd create-oncord-app
npm install
npm run build
npm link
You can now use create-oncord-app
locally.
Contributions are welcome! If you have any questions or want to contribute, you can find the project on GitHub. Feel free to open issues or submit pull requests.
This project is licensed under the MIT License. See the LICENSE file for more details.
Thank you for using OnCord!
This README.md
now includes the detailed Oncord documentation, providing clear instructions and examples for setting up a project using @oncordjs/client
. Adjust the content as needed to fit your specific project.
FAQs
@oncord/client project generator.
The npm package @oncordjs/generate receives a total of 0 weekly downloads. As such, @oncordjs/generate popularity was classified as not popular.
We found that @oncordjs/generate demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.