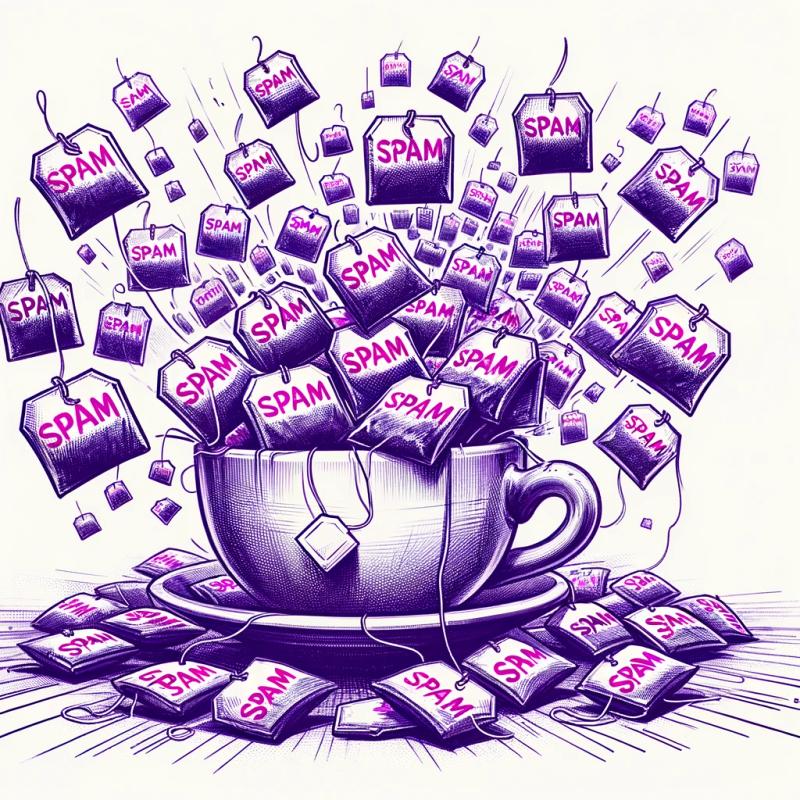
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@react-hook/intersection-observer
Advanced tools
Readme
npm i @react-hook/intersection-observer
The Intersection Observer API provides a way to asynchronously observe changes in the intersection of a target element with an ancestor element or with a top-level document's viewport. The ancestor element or viewport is referred to as the root.
When an IntersectionObserver is created, it's configured to watch for given ratios of visibility within the root. The configuration cannot be changed once the IntersectionObserver is created, so a given observer object is only useful for watching for specific changes in degree of visibility.
import * as React from 'react'
import useIntersectionObserver from '@react-hook/intersection-observer'
const Component = () => {
const [ref, setRef] = React.useState()
const {isIntersecting} = useIntersectionObserver(ref)
return <div ref={setRef}>Is intersecting? {isIntersecting}</div>
}
useIntersectionObserver(target, options)
function useIntersectionObserver<T extends HTMLElement = HTMLElement>(
target: React.RefObject<T> | T | null,
options: IntersectionObserverOptions = {}
): IntersectionObserverEntry
Argument | Type | Required? | Description |
---|---|---|---|
target | React.RefObject | T | null | Yes | A React ref created by useRef() or an HTML element |
options | IntersectionObserverOptions | No | Configuration options for the IntersectionObserver |
Property | Type | Default | Description |
---|---|---|---|
root | DOMElement | document | A specific ancestor of the target element being observed. If no value was passed to the constructor or this is null , the top-level document's viewport is used |
rootMargin | string | "0 0 0 0" | Margin around the root. Can have values similar to the CSS margin property, e.g. "10px 20px 30px 40px" (top, right, bottom, left). The values can be percentages. This set of values serves to grow or shrink each side of the root element's bounding box before computing intersections. |
threshold | number|number[] | 0 | Either a single number or an array of numbers which indicate at what percentage of the target's visibility the observer's callback should be executed. If you only want to detect when visibility passes the 50% mark, you can use a value of 0.5 . If you want the callback to run every time visibility passes another 25%, you would specify the array [0, 0.25, 0.5, 0.75, 1] . The default is 0 (meaning as soon as even one pixel is visible, the callback will be run). A value of 1.0 means that the threshold isn't considered passed until every pixel is visible. |
pollInterval | number | null | The frequency in which the polyfill polls for intersection changes. |
useMutationObserver | bool | true | You can also choose to not check for intersections in the polyfill when the DOM changes by setting this to false |
initialIsIntersecting | bool | false | Changes the default value of isIntersecting for use in places like SSR |
IntersectionObserverEntry
Type | Description |
---|---|
IntersectionObserverEntry | This is the IntersectionObserverEntry object returned by the IntersectionObserver callback. |
MIT
FAQs
A React hook for the IntersectionObserver API that uses a polyfill when the native API is not available
The npm package @react-hook/intersection-observer receives a total of 80,329 weekly downloads. As such, @react-hook/intersection-observer popularity was classified as popular.
We found that @react-hook/intersection-observer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.