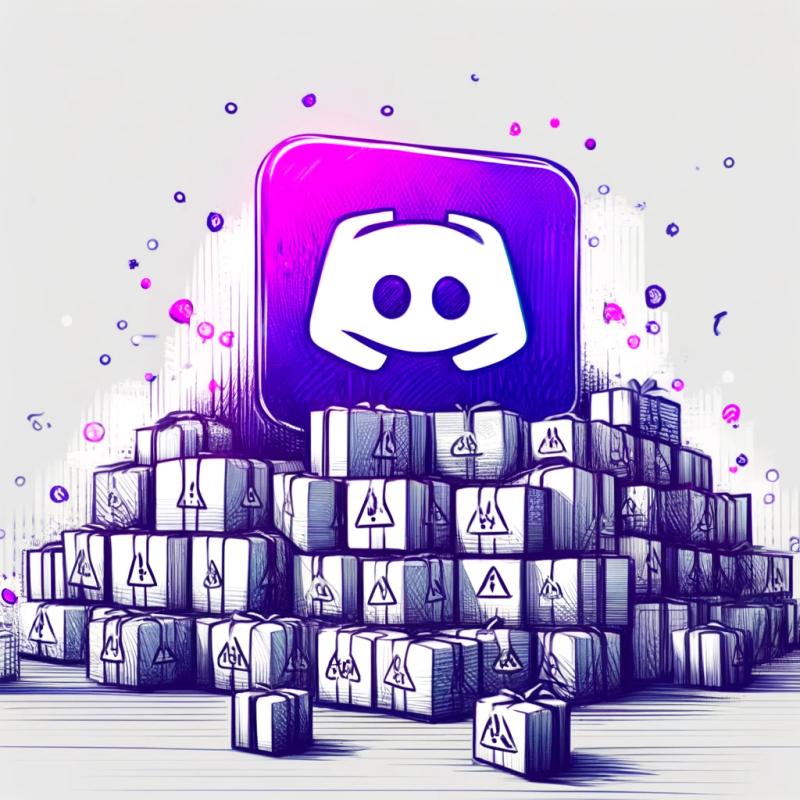
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
@request/api
Advanced tools
Readme
var api = require('@request/api')
var request = api({
// required
type: 'basic', // or 'chain'
// required
define: {
// HTTP request function
// that accepts @request/interface options object
request: require('@request/client')
},
// optional
config: {
// define your own methods and method aliases
}
})
request('url')
request({options})
request('url', function callback (err, res, body) {})
request({options}, function callback (err, res, body) {})
request('url', {options}, function callback (err, res, body) {})
request.[HTTP_VERB]('url')
request.[HTTP_VERB]({options})
request.[HTTP_VERB]('url', function callback (err, res, body) {})
request.[HTTP_VERB]({options}, function callback (err, res, body) {})
request.[HTTP_VERB]('url', {options}, function callback (err, res, body) {})
var api = require('@request/api')
var client = require('@request/client')
var request = api({
type: 'basic',
define: {
request: client
}
})
// GET http://localhost:6767?a=1
request.get('http://localhost:6767', {qs: {a: 1}}, (err, res, body) => {
// request callback
})
var api = require('@request/api')
var client = require('@request/client')
var request = api({
type: 'chain',
define: {
request: client
}
})
// GET http://localhost:6767?a=1
request
.get('http://localhost:6767')
.qs({a: 1})
.callback((err, res, body) => {
// request callback
})
.request()
var api = require('@request/api')
var client = require('@request/client')
var request = api({
type: 'chain',
// API methods configuration
config: {
// HTTP methods
method: {
get: ['select'], // list of aliases
// ...
},
// @request/interface option methods
option: {
qs: ['where'], // list of aliases
// ...
},
// custom methods
custom: {
request: ['fetch', 'snatch', 'submit'], // list of aliases
// ...
}
},
// custom methods implementation
define: {
// `options` is always prepended as first argument
// any other custom arguments follows after that
request: (options, callback) => {
if (callback) {
// `options` contains the generated options object
options.callback = callback
}
// omit the return value if you want to chain further
return client(options)
}
}
})
// GET http://localhost:6767?a=1
request
.select('http://localhost:6767')
.where({a: 1})
.fetch((err, res, body) => {
// request callback
})
var api = require('@request/api')
var client = require('@request/client')
function wrap (options) {
var promise = new Promise((resolve, reject) => {
options.callback = (err, res, body) => {
;(err) ? reject(err) : resolve([res, body])
}
})
client(options)
return promise
}
var request = api({
type: 'basic',
define: {
request: wrap
}
})
// GET http://localhost:6767?a=1
request.get('http://localhost:6767', {qs: 1})
.catch((err) => {})
.then((result) => {})
var request = api({
type: 'chain',
define: {
request: wrap
}
})
// GET http://localhost:6767?a=1
request
.get('http://localhost:6767')
.qs({a: 1})
.request()
.catch((err) => ())
.then((result) => ())
FAQs
Sugar API for @request/interface consumers
We found that @request/api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.