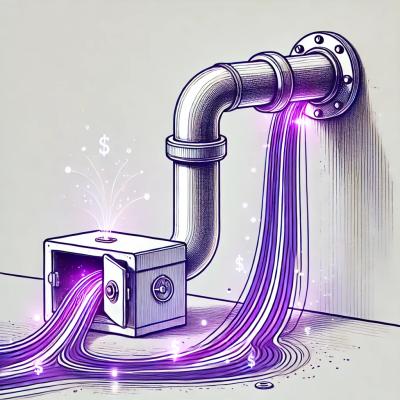
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@sigmacomputing/plugin
Advanced tools
Sigma Computing Plugins provides an API for third-party applications add additional functionality into an existing Sigma workbook.
Plugins are built using Sigma’s Plugin API. This API communicates data and interaction events between a Sigma workbook and the plugin. Plugins are hosted by their developer and rendered in an iframe in Sigma.
@sigmacomputing/plugin
has moved to https://github.com/sigmacomputing/plugin and
is now open source. Please read our
CHANGELOG.md
to review any breaking changes that have been made.
To test your plugin in Sigma Plugin Dev Playground, you must:
To test a development version of a registered plugin, you must:
Have either:
Have "can edit" permission on the workbook
Be in the workbook’s Edit mode
Your plugin must be a Javascript-based project and run in the browser.
Provided you have already followed the steps to create a plugin and a plugin
development environment, you can install @sigmacomputing/plugin
using one of
the following commands
yarn add @sigmacomputing/plugin
# or
npm install @sigmacomputing/plugin
If you have yet to set up your development environment, follow one of the setup guides below
At Sigma, we use React for all of our frontend development. This was taken into consideration when building Sigma’s Plugin feature.
While you are not required to use React for your plugin, it must be written in Javascript and React is recommended. We support both a standard Javascript API and a React Hooks API.
Open your terminal and navigate to the directory you want to create your project in.
Create your new project. We recommend using Facebook’s
create-react-app
.
npx create-react-app <my-cool-plugin>
Navigate to the project's main directory.
cd <my-cool-plugin>
Use your package manager to install Sigma’s plugin library. We recommend
using yarn
.
yarn add @sigmacomputing/plugin
Spin up your local development server.
yarn && yarn start
Start developing:
Plugin developers should have access to a special plugin called Sigma Plugin Dev Playground. This plugin is available from any workbook and points to http://localhost:3000, by default.
If you cannot find this plugin, or would like a development playground with an alternative default host, please contact your Organization Admin with a request to Register a Plugin with its production URL set to your preferred development URL.
Before you start:
Set your plugin’s development URL to http://localhost:3000.
Start your plugin locally
Note: If you followed our recommendations under #create-a-development-project, enter the following command in your terminal:
yarn && yarn start
Note: The editor panel will only display content if you have configured your plugin using Sigma’s plugin Configuration API. Likewise, the element will only display content if your plugin is configured to display content. If you change a plugin's configuration options, input values will need to be re-added in the editor panel.
Now what?
A plugin can be configured with any number of configuration fields. Each field type has its own configuration options. Each field type is also guaranteed to have the following options:
name : string
- the name of the fieldtype : string
- the field typelabel : string (optional)
- a display name for the fieldtype CustomPluginConfigOptions =
| {
type: 'group';
name: string;
label?: string;
}
| {
type: 'element';
name: string;
label?: string;
}
| {
type: 'column';
name: string;
label?: string;
allowedTypes?: ValueType[];
source: string;
allowMultiple: boolean;
}
| {
type: 'text';
name: string;
label?: string;
source?: string; // can point to a group or element config
secure?: boolean; // if true will omit from prehydrated configs
multiline?: boolean;
placeholder?: string;
defaultValue?: string;
}
| {
type: 'toggle';
name: string;
label?: string;
source?: string;
defaultValue?: boolean;
}
| {
type: 'checkbox';
name: string;
label?: string;
source?: string;
defaultValue?: boolean;
}
| {
type: 'radio';
name: string;
label?: string;
source?: string;
values: string[];
singleLine?: boolean;
defaultValue?: string;
}
| {
type: 'dropdown';
name: string;
label?: string;
source?: string;
width?: string;
values: string[];
defaultValue?: string;
}
| {
type: 'color';
name: string;
label?: string;
source?: string;
}
| {
type: 'variable';
name: string;
label?: string;
allowedTypes?: ControlType[];
}
| {
type: 'interaction';
name: string;
label?: string;
}
| {
type: 'action-trigger';
name: string;
label?: string;
}
| {
type: 'action-effect';
name: string;
label?: string;
};
Group
Can be used to identify a group of related fields
Element
A custom element that is added by your plugin
Column
A custom column configuration that your plugin uses
Additional Fields
allowedTypes : ValueType[] (optional)
- the allowed data types that this
column can contain where ValueType
has the following type:
type ValueType =
| 'boolean'
| 'datetime'
| 'number'
| 'integer'
| 'text'
| 'variant'
| 'link'
| 'error';
source : string
- the data source that should be used to supply this field
allowMultiple : boolean
- whether multiple columns should be allowed as
input for this field
Text
A configurable text input for your plugin
Additional Fields
source : string (optional)
- the data source that should be used to supply this fieldsecure : boolean (optional)
- whether to omit input from pre-hydrated configsmultiline : boolean (optional)
- whether this text input should allow
multiple linesplaceholder : string (optional)
- the placeholder for this input fielddefaultValue : string (optional)
- the default value for this input fieldToggle
A configurable toggle for your plugin
Additional Fields
source : string (optional)
- the data source that should be used to supply this fielddefaultValue : boolean (optional)
- the default value for this input fieldCheckbox
A configurable checkbox for your plugin
Additional Fields
source : string (optional)
- the data source that should be used to supply this fielddefaultValue : boolean (optional)
- the default value for this input fieldRadio
A configurable radio button for your plugin
Additional Fields
source : string (optional)
- the data source that should be used to supply
this fieldvalues : string[]
- the options to show for this input fieldsingleLine : boolean (optional)
- whether to display options on a single
line. Good for (2-3) optionsdefaultValue : boolean (optional)
- the default value for this input fieldDropdown
A configurable dropdown for your plugin
Additional Fields
source : string (optional)
- the data source that should be used to supply
this fieldvalues : string[]
- the options to show for this input fieldwidth : string (optional)
- how wide the dropdown should be in pixelsdefaultValue : boolean (optional)
- the default value for this input fieldColor
A configurable color picker for your plugin
Additional Fields
source : string (optional)
- the data source that should be used to supply
this fieldVariable
A configurable workbook variable to control other elements within your workbook
Additional Fields
allowedTypes : ControlType[] (optional)
- the allowed control types that this
variable can use where ControlType
has the following type:
type ControlType =
| 'boolean'
| 'date'
| 'number'
| 'text'
| 'text-list'
| 'number-list'
| 'date-list'
| 'number-range'
| 'date-range';
Interaction
A configurable workbook interaction to interact with other charts within your workbook
Action Trigger
A configurable action trigger to trigger actions in other elements within your workbook
Action Effect
A configurable action effect that can be triggered by other elements within your workbook
interface PluginInstance<T> {
sigmaEnv: 'author' | 'viewer' | 'explorer';
config: {
/**
* Getter for entire Plugin Config
*/
get(): Partial<T> | undefined;
/**
* Performs a shallow merge between current config and passed in config
*/
set(config: Partial<T>): void;
/**
* Getter for key within plugin config
*/
getKey<K extends keyof T>(key: K): Pick<T, K>;
/**
* Assigns key value pair within plugin
*/
setKey<K extends keyof T>(key: K, value: Pick<T, K>): void;
/**
* Subscriber for Plugin Config
*/
subscribe(listener: (arg0: T) => void): Unsubscriber;
/**
* Set possible options for plugin config
*/
configureEditorPanel(options: CustomPluginConfigOptions[]): void;
/**
* Gets a static image of a workbook variable
*/
getVariable(configId: string): WorkbookVariable;
/**
* Setter for workbook variable passed in
*/
setVariable(configId: string, ...values: unknown[]): void;
/**
* Getter for interaction selection state
*/
getInteraction(configId: string): WorkbookSelection[];
/**
* Setter for interaction selection state
*/
setInteraction(
configId: string,
elementId: string,
selection: WorkbookSelection[],
): void;
/**
* Triggers an action based on the provided action trigger ID
*/
triggerAction(configId: string): void;
/**
* Registers an effect with the provided action effect ID
*/
registerEffect(configId: string, effect: Function): void;
/**
* Overrider function for Config Ready state
*/
setLoadingState(ready: boolean): void;
/**
* Allows users to subscribe to changes in the passed in variable
*/
subscribeToWorkbookVariable(
configId: string,
callback: (input: WorkbookVariable) => void,
): Unsubscriber;
/**
* @deprecated Use Action API instead
* Allows users to subscribe to changes in the passed in interaction ID
*/
subscribeToWorkbookInteraction(
configId: string,
callback: (input: WorkbookSelection[]) => void,
): Unsubscriber;
};
elements: {
/**
* Getter for Column Data by parent sheet ID
*/
getElementColumns(configId: string): Promise<WbElementColumns>;
/**
* Subscriber to changes in column data by ID
*/
subscribeToElementColumns(
configId: string,
callback: (cols: WbElementColumns) => void,
): Unsubscriber;
/**
* Subscriber for the data within a given sheet
*/
subscribeToElementData(
configId: string,
callback: (data: WbElementData) => void,
): Unsubscriber;
};
/**
* Destroys plugin instance and removes all subscribers
*/
destroy(): void;
}
The client is a pre-initialized plugin instance. You can use this instance
directly or create your own instance using initialize
const client: PluginInstance = initialize();
Usage
import { client } from '@sigmacomputing/plugin';
client.config.configureEditorPanel([
{ name: 'source', type: 'element' },
{ name: 'dimension', type: 'column', source: 'source', allowMultiple: true },
]);
Instead of using the pre-initialized plugin instance, you can create your own plugin instance.
function initialize<T = {}>(): PluginInstance<T>;
Usage
import { initialize } from '@sigmacomputing/plugin';
const myClient: PluginInstance = initialize();
myClient.config.configureEditorPanel([
{ name: 'source', type: 'element' },
{ name: 'dimension', type: 'column', source: 'source', allowMultiple: true },
]);
A context provider your plugin that enables all of the other React API hooks. You should wrap your plugin with this provider if your want to use the plugin hook API.
interface SigmaClientProviderProps {
client: PluginInstance;
children?: ReactNode;
}
function SigmaClientProvider(props: SigmaClientProviderProps): ReactNode;
Gets the entire plugin instance
function usePlugin(): PluginInstance;
Provides a setter for the plugin's configuration options
function useEditorPanelConfig(nextOptions: CustomPluginConfigOptions[]): void;
Provides a setter for the Plugin's Config Options
Arguments
nextOptions : CustomPluginConfigOptions[]
- Updated possible Config OptionsGets the current plugin's loading stat. Returns a value and a setter allowing you to update the plugin's loading state
function useLoadingState(
initialState: boolean,
): [boolean, (nextState: boolean) => void];
Arguments
initialState : boolean
- Initial value to set loading state toProvides the latest column values from corresponding sheet
function useElementColumns(elementId: string): WorkbookElementColumns;
Arguments
elementId : string
- A workbook element’s unique identifier.Returns the column information from the specified element.
interface WorkbookElementColumn {
id: string;
name: string;
columnType: ValueType;
}
interface WorkbookElementColumns {
[colId: string]: WbElementColumn;
}
Provides the latest data values from corresponding sheet, up to 25000 values.
function useElementData(configId: string): WorkbookElementData;
Arguments
configId : string
- A workbook element’s unique identifier from the plugin config.Returns the row data from the specified element.
interface WorkbookElementData {
[colId: string]: any[];
}
Provides the latest data values from the corresponding sheet (initially 25000), and provides a callback for fetching more data in chunks of 25000 values.
function useElementData(configId: string): [WorkbookElementData, () => void];
Arguments
configId : string
- A workbook element’s unique identifier from the plugin config.Returns the row data from the specified element, and a callback for fetching more data.
interface WorkbookElementData {
[colId: string]: any[];
}
Returns a given variable's value and a setter to update that variable
function useVariable(
configId: string,
): [WorkbookVariable | undefined, (...values: unknown[]) => void];
Arguments
configId : string
- The config ID corresponding to the workbook control variableThe returned setter function accepts 1 or more variable values expressed as an array or multiple parameters
function setVariableCallback(...values: unknown[]): void;
Returns a given interaction's selection state and a setter to update that interaction
function useInteraction(
configId: string,
elementId: string,
): [WorkbookSelection | undefined, (value: WorkbookSelection[]) => void];
Arguments
configId : string
- The config ID corresponding to the workbook interactionelementId : string
- The ID of the element that this interaction is
associated withThe returned setter function accepts an array of workbook selection elements
function setVariableCallback(value: WorkbookSelection[]): void;
configId : string
- The config ID corresponding to the action triggerReturns a callback function to trigger one or more action effects for a given action trigger
function useActionTrigger(configId: string): () => void;
Arguments
configId : string
- The config ID corresponding to the action triggerThe function that can be called to asynchronously trigger the action
function triggerActionCallback(configId: string): void;
Registers and unregisters an action effect within the plugin
function useActionEffect(configId: string, effect: () => void);
Arguments
configId : string
- The config ID corresponding to the action effecteffect : Function
- The function to be called when the effect is triggeredReturns the workbook element’s current configuration. If a key is provided, only the associated configuration is returned.
function useConfig(key?: string): any;
Arguments
key : string (optional)
- The name of a key within the associated
PluginConfigOptions
objectSigma’s development team has created a set of example plugins, listed below.
All of our example plugins are hosted and can be added to your organization. To view / add an example plugin to your organization, follow the steps to register a plugin using its Production URL, listed below.
You can also visit Sigma’s Sample Plugin repository directly on Github.
Recharts Bar Chart - A basic bar chart built with the Recharts library.
D3 Candlestick - A candlestick visualization built with D3.
Narrative Science Quill - Demonstrates secure text entry.
D3 Graph - Demonstrates usage of multiple data sources and in-memory joins.
D3 Sunburst - A sunburst visualization built with D3.
Frappe Heatmap - A basic Frappe visualization example.
To add an example plugin to your organization, follow the steps to register a plugin using its Production URL, listed in the examples above.
Open your terminal, and navigate to the directory you want to save the example’s in.
Clone Sigma’s Sample Plugin repository.
git clone https://github.com/sigmacomputing/sigma-sample-plugins.git
Navigate to the plugin you would like to try.
cd sigma-sample-plugins/<plugin-name>
Run the plugin.
yarn && yarn start
Note: For additional instructions, visit the README file located in the main directory of any given example plugin.
As a plugin developer, you are responsible for hosting your plugin(s). If you’re new to hosting your own projects, here are a few popular hosting platforms you can get started with:
We welcome contributions to @sigmacomputing/plugin
!
🐛 Issues, 📥 Pull requests and 🌟 Stars are always welcome. Read our contributing guide to get started.
yarn format
Format your code to match the sigmacomputing style guide
yarn test
Check if the unit tests all pass
You can also run the tests in
--watch
mode with yarn test:watch
FAQs
Sigma Computing Plugin Client API
The npm package @sigmacomputing/plugin receives a total of 3,868 weekly downloads. As such, @sigmacomputing/plugin popularity was classified as popular.
We found that @sigmacomputing/plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.