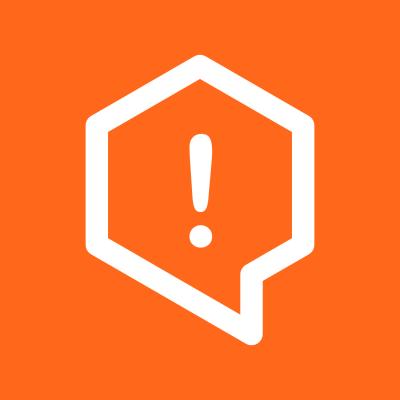
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@sphereon/ssi-sdk-ext.jwt-service
Advanced tools
A plugin that can generate and verify JWTs. It can create/sign JWS in Compact, JSON General and JSON Flattened form as specified in RFC 7515
Currently, it supports the following JWS forms:
The plugin is using the Universal Identifier Resolution module. Both for generating JWS JWTs as well as for verifying JWTs.
When signing a JWS it takes into account any x5c, kid or JWK value already present in the header, as well as the iss
value. When not present but a Managed Identifier is being provided, the signing service will take care of putting the
correct headers into the JWS.
The jwtCreateJswCompactSignature
accepts a protected JWT header. You can put any JWT header properties in there.
The payload
can either be a base64url payload, a JwtPayload
object or a Buffer/Uint8arry. The method will take care
of any relevant conversions
The issuer
object allows you to provide a managed identifier
const publicKeyHex = '037fcdce2770f6c45d4183cbee6fdb4b7b580733357be9ef13bacf6e3c7bd15445'
const kid = publicKeyHex
const example = await agent.jwtCreateJwsCompactSignature({
// Example payloads from IETF spec
issuer: { identifier: kid, noIdentifierInHeader: true }, // do not update any header values with the provided identifier. Just use the identifier for signing
protectedHeader: { alg: 'ES256' },
payload: 'eyJpc3MiOiJqb2UiLA0KICJleHAiOjEzMDA4MTkzODAsDQogImh0dHA6Ly9leGFtcGxlLmNvbS9pc19yb290Ijp0cnVlfQ',
})
Verify the signature:
You can optionally provide a JWK if you want to use the JWK as a key for verification. Otherwise it will automacally resolve the header params like x5c, kid (DID), JWK to perform the resolution with the Universal Identifier Resolution module
const ietfJwk = {
kty: 'EC',
crv: 'P-256',
x: 'f83OJ3D2xF1Bg8vub9tLe1gHMzV76e8Tus9uPHvRVEU',
y: 'x_FEzRu9m36HLN_tue659LNpXW6pCyStikYjKIWI5a0',
// d: 'jpsQnnGQmL-YBIffH1136cspYG6-0iY7X1fCE9-E9LI',
} satisfies JWK
const result = await agent.jwtVerifyJwsSignature({
jws: example.jwt,
jwk: ietfJwk,
})
const result = {
critical: false,
error: false,
jws: {
payload: 'eyJpc3MiOiJqb2UiLA0KICJleHAiOjEzMDA4MTkzODAsDQogImh0dHA6Ly9leGFtcGxlLmNvbS9pc19yb290Ijp0cnVlfQ',
signatures: [
{
identifier: {
jwk: {
crv: 'P-256',
kty: 'EC',
x: 'f83OJ3D2xF1Bg8vub9tLe1gHMzV76e8Tus9uPHvRVEU',
y: 'x_FEzRu9m36HLN_tue659LNpXW6pCyStikYjKIWI5a0',
},
jwks: [
{
jwk: {
crv: 'P-256',
kty: 'EC',
x: 'f83OJ3D2xF1Bg8vub9tLe1gHMzV76e8Tus9uPHvRVEU',
y: 'x_FEzRu9m36HLN_tue659LNpXW6pCyStikYjKIWI5a0',
},
jwkThumbprint: 'oKIywvGUpTVTyxMQ3bwIIeQUudfr_CkLMjCE19ECD-U',
publicKeyHex: '037fcdce2770f6c45d4183cbee6fdb4b7b580733357be9ef13bacf6e3c7bd15445',
},
],
method: 'jwk',
},
protected: 'eyJhbGciOiJFUzI1NiJ9',
signature: 'e4ZrhZdbFQ7630Tq51E6RQiJaae9bFNGJszIhtusEwzvO21rzH76Wer6yRn2Zb34VjIm3cVRl0iQctbf4uBY3w',
},
],
},
message: 'Signature validated',
name: 'jws',
verificationTime: '2024-08-10T23:04:23',
}
pnpm add @sphereon/ssi-sdk-ext.jwt-service
pnpm run build
The test command runs:
prettier
jest
coverage
You can also run only a single section of these tests, using for example yarn test:unit
.
pnmp run test
FAQs
Unknown package
The npm package @sphereon/ssi-sdk-ext.jwt-service receives a total of 9,453 weekly downloads. As such, @sphereon/ssi-sdk-ext.jwt-service popularity was classified as popular.
We found that @sphereon/ssi-sdk-ext.jwt-service demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.