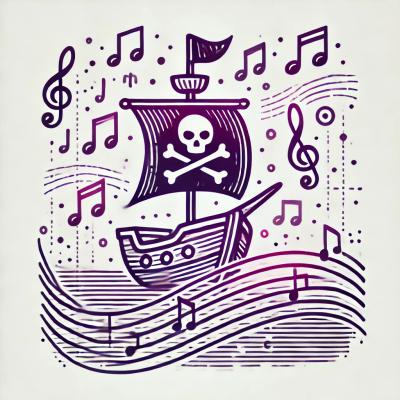
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
@stacks/bns
Advanced tools
@stacks/bns
A package for interacting with the BNS contract on the Stacks blockchain.
The Blockchain Naming System (BNS) is a network system that binds Stacks usernames to off-chain state without relying on any central points of control.
npm install --save @stacks/bns
Check if name can be registered
import { canRegisterName } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
const result = await canRegisterName({ fullyQualifiedName, network });
// true / false
Get price of name registration in microstacks
import { getNamePrice } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
const price = await getNamePrice({ fullyQualifiedName, network });
// price of name registration in microstacks
Send two transaction to secure a name, preorder and then register.
import { buildPreorderNameTx } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
import {
TxBroadcastResult,
broadcastTransaction,
publicKeyToString,
TransactionSigner,
createStacksPrivateKey,
pubKeyfromPrivKey,
} from '@stacks/transactions';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
const salt = 'salt';
const stxToBurn = 200n;
// warning: Do not expose your private key by hard coding in code. Use env variables to load private keys.
const privateKey = '<insert private key here>'; // process.env.privateKey
const publicKey = publicKeyToString(pubKeyfromPrivKey(privateKey));
// construct an unsigned bns priorder-name transaction
// note: builder functions build transactions with AnchorMode set to Any
const unsignedTX = await buildPreorderNameTx({
fullyQualifiedName,
salt,
stxToBurn,
publicKey,
network,
});
// Sign the transaction with private key and broadcast to the network
const signer = new TransactionSigner(unsignedTX);
signer.signOrigin(createStacksPrivateKey(privateKey));
const reply: TxBroadcastResult = await broadcastTransaction(signer.transaction, network);
// reply.txid
// Wait for the transaction to be confirmed before sending register transaction
import { buildRegisterNameTx } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
import {
TxBroadcastResult,
broadcastTransaction,
publicKeyToString,
TransactionSigner,
createStacksPrivateKey,
pubKeyfromPrivKey,
} from '@stacks/transactions';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
// warning: Do not expose your private key by hard coding in code. Use env variables to load private keys.
const privateKey = '<insert private key here>'; // process.env.privateKey
const zonefile = 'zonefile';
const salt = 'salt';
const publicKey = publicKeyToString(pubKeyfromPrivKey(privateKey));
// construct an unsigned bns register-name transaction
// note: builder functions build transactions with AnchorMode set to Any
const unsignedTX = await buildRegisterNameTx({
fullyQualifiedName,
publicKey,
salt,
zonefile,
network,
});
// Sign the transaction with private key and broadcast to the network
const signer = new TransactionSigner(unsignedTX);
signer.signOrigin(createStacksPrivateKey(privateKey));
const reply: TxBroadcastResult = await broadcastTransaction(
signer.transaction,
network,
Buffer.from(zonefile)
);
// reply.txid
Transfer the ownership to other address
import { buildTransferNameTx } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
import {
TxBroadcastResult,
broadcastTransaction,
publicKeyToString,
TransactionSigner,
createStacksPrivateKey,
pubKeyfromPrivKey,
} from '@stacks/transactions';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
const newOwnerAddress = 'ST1HB1T8WRNBYB0Y3T7WXZS38NKKPTBR3EG9EPJKR';
// warning: Do not expose your private key by hard coding in code. Use env variables to load private keys.
const privateKey = '<insert private key here>'; // process.env.privateKey
const zonefile = 'zonefile';
const publicKey = publicKeyToString(pubKeyfromPrivKey(privateKey));
// construct an unsigned bns transfer-name transaction
// note: builder functions build transactions with AnchorMode set to Any
const unsignedTX = await buildTransferNameTx({
fullyQualifiedName,
newOwnerAddress,
publicKey,
zonefile,
network,
});
// Sign the transaction with private key and broadcast to the network
const signer = new TransactionSigner(unsignedTX);
signer.signOrigin(createStacksPrivateKey(privateKey));
const reply: TxBroadcastResult = await broadcastTransaction(
signer.transaction,
network,
Buffer.from(zonefile)
);
// reply.txid
Generates a name update transaction. This changes the zonefile for the registered name.
import { buildUpdateNameTx } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
import {
TxBroadcastResult,
broadcastTransaction,
publicKeyToString,
TransactionSigner,
createStacksPrivateKey,
pubKeyfromPrivKey,
} from '@stacks/transactions';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
// warning: Do not expose your private key by hard coding in code. Use env variables to load private keys.
const privateKey = '<insert private key here>'; // process.env.privateKey
const zonefile = 'zonefile';
const publicKey = publicKeyToString(pubKeyfromPrivKey(privateKey));
// construct an unsigned bns update-name transaction
// note: builder functions build transactions with AnchorMode set to Any
const unsignedTX = await buildUpdateNameTx({
fullyQualifiedName,
zonefile,
publicKey,
network,
});
// Sign the transaction with private key and broadcast to the network
const signer = new TransactionSigner(unsignedTX);
signer.signOrigin(createStacksPrivateKey(privateKey));
const reply: TxBroadcastResult = await broadcastTransaction(
signer.transaction,
network,
Buffer.from(zonefile)
);
// reply.txid
Generates a name renew transaction. This renews a name registration.
import { buildRenewNameTx } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
import {
TxBroadcastResult,
broadcastTransaction,
publicKeyToString,
TransactionSigner,
createStacksPrivateKey,
pubKeyfromPrivKey,
} from '@stacks/transactions';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
const stxToBurn = 10n;
const newOwnerAddress = 'ST1HB1T8WRNBYB0Y3T7WXZS38NKKPTBR3EG9EPJKR';
// warning: Do not expose your private key by hard coding in code. Use env variables to load private keys.
const privateKey = '<insert private key here>'; // process.env.privateKey
const zonefile = 'zonefile';
const publicKey = publicKeyToString(pubKeyfromPrivKey(privateKey));
// construct an unsigned bns renew-name transaction
// note: builder functions build transactions with AnchorMode set to Any
const unsignedTX = await buildRenewNameTx({
fullyQualifiedName,
stxToBurn,
newOwnerAddress,
zonefile,
publicKey,
network,
});
// Sign the transaction with private key and broadcast to the network
const signer = new TransactionSigner(unsignedTX);
signer.signOrigin(createStacksPrivateKey(privateKey));
const reply: TxBroadcastResult = await broadcastTransaction(
signer.transaction,
network,
Buffer.from(zonefile)
);
// reply.txid
Generates a name revoke transaction. This revokes a name registration.
import { buildRevokeNameTx } from '@stacks/bns';
import { StacksTestnet, StacksMainnet } from '@stacks/network';
import {
TxBroadcastResult,
broadcastTransaction,
publicKeyToString,
TransactionSigner,
createStacksPrivateKey,
pubKeyfromPrivKey,
} from '@stacks/transactions';
// for mainnet, use `StacksMainnet()`
const network = new StacksTestnet();
const fullyQualifiedName = 'name.id';
// warning: Do not expose your private key by hard coding in code. Use env variables to load private keys.
const privateKey = '<insert private key here>'; // process.env.privateKey
const publicKey = publicKeyToString(pubKeyfromPrivKey(privateKey));
// construct an unsigned bns revoke-name transaction
// note: builder functions build transactions with AnchorMode set to Any
const unsignedTX = await buildRevokeNameTx({
fullyQualifiedName,
publicKey,
network,
});
// Sign the transaction with private key and broadcast to the network
const signer = new TransactionSigner(unsignedTX);
signer.signOrigin(createStacksPrivateKey(privateKey));
const reply: TxBroadcastResult = await broadcastTransaction(signer.transaction, network);
// reply.txid
FAQs
Library for working with the Stacks Blockchain Naming System BNS.
The npm package @stacks/bns receives a total of 51 weekly downloads. As such, @stacks/bns popularity was classified as not popular.
We found that @stacks/bns demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.