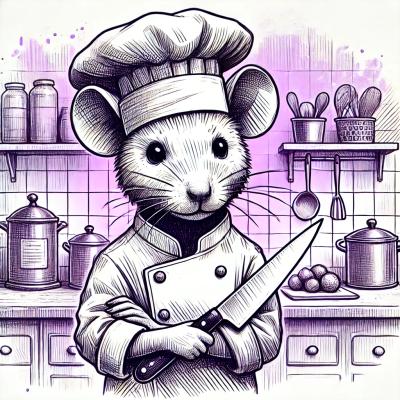
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@types/command-line-args
Advanced tools
TypeScript definitions for command-line-args
@types/command-line-args provides TypeScript type definitions for the command-line-args package, which is used to parse command-line arguments in Node.js applications.
Define Command Line Options
This feature allows you to define the command-line options your application will accept. In this example, 'file' is a string option and 'verbose' is a boolean option.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'file', type: String },
{ name: 'verbose', type: Boolean }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Default Option Values
You can set default values for options. In this example, if 'file' or 'verbose' are not provided, they will default to 'default.txt' and 'false' respectively.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'file', type: String, defaultValue: 'default.txt' },
{ name: 'verbose', type: Boolean, defaultValue: false }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Multiple Option Values
This feature allows you to accept multiple values for a single option. In this example, 'files' can accept multiple string values.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'files', type: String, multiple: true }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Yargs is a popular library for parsing command-line arguments in Node.js. It provides a rich set of features including command handling, argument validation, and more. Compared to command-line-args, yargs offers more advanced features but can be more complex to use.
Commander is another widely-used library for command-line argument parsing in Node.js. It is known for its simplicity and ease of use. While it may not offer as many features as yargs, it is more straightforward and easier to get started with compared to command-line-args.
Minimist is a lightweight library for parsing command-line arguments. It is very simple and fast, making it suitable for smaller projects or scripts. However, it lacks some of the advanced features found in command-line-args and other more comprehensive libraries.
npm install --save @types/command-line-args
This package contains type definitions for command-line-args (https://github.com/75lb/command-line-args).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/command-line-args.
These definitions were written by Lloyd Brookes.
FAQs
TypeScript definitions for command-line-args
The npm package @types/command-line-args receives a total of 224,894 weekly downloads. As such, @types/command-line-args popularity was classified as popular.
We found that @types/command-line-args demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.