What is @ui5/project?
@ui5/project is a Node.js-based toolset for managing UI5 projects. It provides functionalities for building, developing, and managing UI5 applications and libraries. The package is part of the UI5 Tooling ecosystem, which aims to streamline the development process for UI5 applications.
What are @ui5/project's main functionalities?
Project Initialization
This feature allows you to initialize a new UI5 project with a specified ID, version, and path. It sets up the necessary project structure and configuration.
const { project } = require('@ui5/project');
const myProject = project.create({
id: 'my.project.id',
version: '1.0.0',
path: './path/to/project'
});
Building Projects
This feature allows you to build your UI5 project, compiling and bundling the resources into a distribution folder. It ensures that your project is ready for deployment.
const { builder } = require('@ui5/project');
const myProject = project.create({
id: 'my.project.id',
version: '1.0.0',
path: './path/to/project'
});
builder.build({
project: myProject,
destPath: './path/to/dist'
});
Dependency Management
This feature allows you to manage dependencies for your UI5 project. You can add, remove, or update dependencies, ensuring that your project has all the necessary libraries and modules.
const { project } = require('@ui5/project');
const myProject = project.create({
id: 'my.project.id',
version: '1.0.0',
path: './path/to/project'
});
myProject.dependencies.add({
id: 'some.dependency.id',
version: '1.2.3'
});
Other packages similar to @ui5/project
gulp
Gulp is a toolkit for automating painful or time-consuming tasks in your development workflow. It is similar to @ui5/project in that it can be used to build and manage projects, but it is more general-purpose and not specifically tailored to UI5 applications.
grunt
Grunt is a JavaScript task runner that automates repetitive tasks like minification, compilation, unit testing, and linting. Like @ui5/project, it can be used to build and manage projects, but it is not specialized for UI5 applications.
webpack
Webpack is a module bundler for modern JavaScript applications. It takes modules with dependencies and generates static assets representing those modules. While it can be used to build UI5 projects, it is more complex and general-purpose compared to @ui5/project.
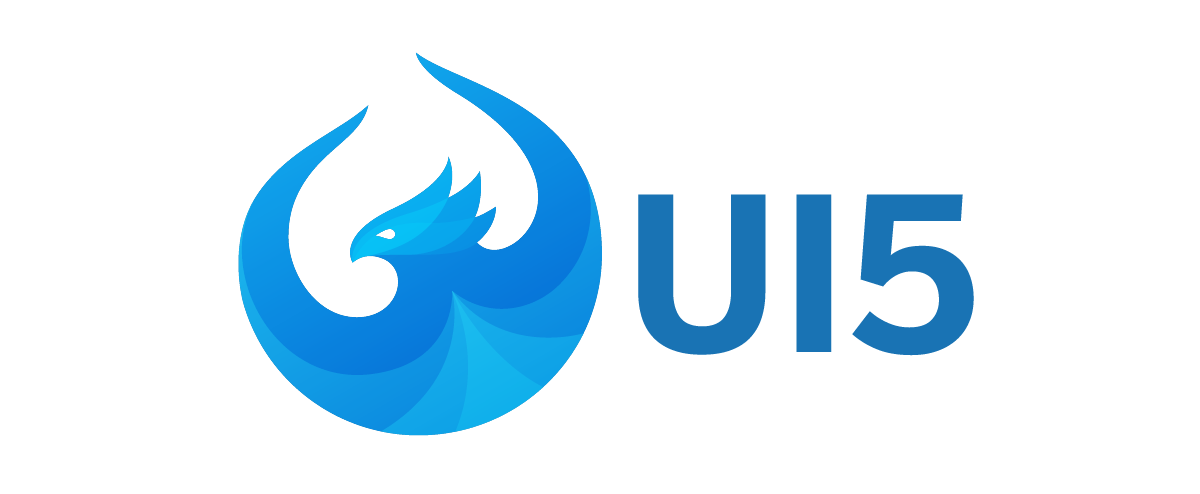
ui5-project
Modules for building a UI5 projects dependency tree, including configuration.
Part of the UI5 Build and Development Tooling

This is an alpha release!
The UI5 Build and Development Tooling described here is not intended for productive use yet. Breaking changes are to be expected.
Normalizer
The purpose of the normalizer is to collect dependency information and to enrich it with project configuration (generateProjectTree).
Translators are used to collect dependency information. The Project Preprocessor enriches this dependency information with project configuration, typically from a ui5.yaml
file. A development server and build process can use this information to locate project and dependency resources.
If you want to retrieve the project dependency graph only, use (generateDependencyTree).
Translators
Translators collect recursively all dependencies on a package manager specific layer and return information about them in a well-defined tree structure.
Tree Structure Returned by a Translator
The following dependency tree is the expected input structure of the Project Preprocessor:
{
"id": "projectA",
"version": "1.0.0",
"path": "/absolute/path/to/projectA",
"dependencies": [
{
"id": "projectB",
"version": "1.0.0",
"path": "/path/to/projectB",
"dependencies": [
{
"id": "projectD",
"path": "/path/to/different/projectD"
}
]
},
{
"id": "projectD",
"version": "1.0.0",
"path": "/path/to/projectD"
},
{
"id": "myStaticServerTool",
"version": "1.0.0",
"path": "/path/to/some/dependency"
}
]
}
npm Translator
The npm translator is currently the default translator and looks for dependencies defined in the package.json
file of a certain project. dependencies
, devDepedencies
, and napa dependencies (Git repositories which don't have a package.json
file) are located via the Node.js module resolution logic.
Static Translator
This translator is currently intended for testing purposes only.
Can be used to supply the full dependency information of a project in a single structured file.
Example: ui5 serve -b static:/path/to/projectDependencies.yaml
projectDependencies.yaml
contains something like:
---
id: testsuite
version: "",
path: "./"
dependencies:
- id: sap.f
version: "",
path: "../sap.f"
- id: sap.m
version: "",
path: "../sap.m"
Project Preprocessor
Enhances a given dependency tree based on a projects configuration.
Enhanced Dependency Tree Structure Returned by the Project Preprocessor
{
"id": "projectA",
"version": "1.0.0",
"path": "/absolute/path/to/projectA",
"specVersion": "0.1",
"type": "application",
"metadata": {
"name": "sap.projectA",
"copyright": "Some copyright ${currentYear}"
},
"resources": {
"configuration": {
"paths": {
"webapp": "app"
}
},
"pathMappings": {
"/": "app"
}
},
"dependencies": [
{
"id": "projectB",
"version": "1.0.0",
"path": "/path/to/projectB",
"specVersion": "0.1",
"type": "library",
"metadata": {
"name": "sap.ui.projectB"
},
"resources": {
"configuration": {
"paths": {
"src": "src",
"test": "test"
}
},
"pathMappings": {
"/resources/": "src",
"/test-resources/": "test"
}
},
"dependencies": [
{
"id": "projectD",
"version": "1.0.0",
"path": "/path/to/different/projectD",
"specVersion": "0.1",
"type": "library",
"metadata": {
"name": "sap.ui.projectD"
},
"resources": {
"configuration": {
"paths": {
"src": "src/main/uilib",
"test": "src/test"
}
},
"pathMappings": {
"/resources/": "src/main/uilib",
"/test-resources/": "src/test"
}
},
"dependencies": []
}
]
},
{
"id": "projectD",
"version": "1.0.0",
"path": "/path/to/projectD",
"specVersion": "0.1",
"type": "library",
"metadata": {
"name": "sap.ui.projectD"
},
"resources": {
"configuration": {
"paths": {
"src": "src/main/uilib",
"test": "src/test"
}
},
"pathMappings": {
"/resources/": "src/main/uilib",
"/test-resources/": "src/test"
}
},
"dependencies": []
}
]
}
Configuration
Project Configuration
Typically located in a ui5.yaml
file per project.
Structure
YAML
---
specVersion: "0.1"
type: application|library|custom
metadata:
name: testsuite
copyright: |-
UI development toolkit for HTML5 (OpenUI5)
* (c) Copyright 2009-${currentYear} SAP SE or an SAP affiliate company.
* Licensed under the Apache License, Version 2.0 - see LICENSE.txt.
resources:
configuration:
paths:
"<virtual path>": "<physical path>"
"<virtual path 2>": "<physical path 2>"
shims:
some-dependency-name:
type: library
metadata:
name: library-xy
resources:
configuration:
paths:
"src": "source"
builder:
customTasks:
- name: custom-task-name-1
beforeTask: standard-task-name
configuration:
configuration-key: value
- name: custom-task-name-2
afterTask: custom-task-name-1
configuration:
color: blue
server:
settings:
port: 8099
Properties
<root>
specVersion
: Version of the specificationtype
: Either application
, library
or custom
(custom not yet implemented); defines the default path mappings and build steps. Custom doesn't define any specific defaults.
metadata
Some general information:
name
: Name of the application/library/resourcecopyright
(optional): String to be used for replacement of copyright placeholders in the project
resources (optional)
configuration
paths
: Mapping between virtual and physical paths
- For type
application
there can be a setting for mapping the virtual path webapp
to a physical path within the project - For type
library
there can be a setting for mapping the virtual paths src
and test
to physical paths within the project
shims
: Can be used to define, extend, or override UI5 configs of dependencies. Inner structure equals the general structure. It is a key-value map where the key equals the project ID as supplied by the translator.
builder (optional)
customTasks
(optional, list): In this block, you define additional custom build tasks, see here for a detailed explanation and examples of the build extensibility. Each entry in the customTasks
list consists of the following options:
name
(mandatory): The name of the custom taskafterTask
or beforeTask
(only one, mandatory): The name of the build task after or before which your custom task will be executed.configuration
(optional): Additional configuration that is passed to the custom build task
server (optional)
settings
(not yet implemented)
port
: Project default server port; can be overwritten via CLI parameters
Contributing
Please check our Contribution Guidelines.
Support
Please follow our Contribution Guidelines on how to report an issue.
Release History
See CHANGELOG.md.
License
This project is licensed under the Apache Software License, Version 2.0 except as noted otherwise in the LICENSE file.