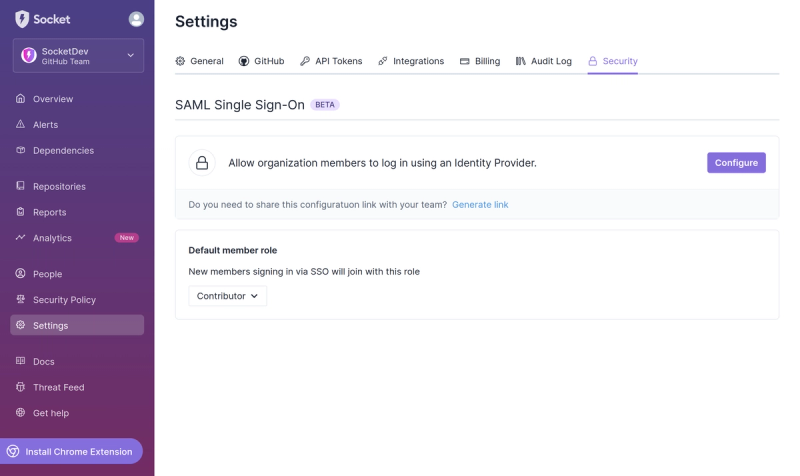
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@woosmap/react-native-woosmap
Advanced tools
Readme
The Woosmap react SDK is a library that use to embed interactive maps directly into your application. You can also add stores overlay to the map to call out points of interest and get relative information.
The SDK offers an interface to manage the Indoor Mapview and to subscribe to events that happen on the map.
Please get your token from your account. You may ask for one if necessary or you can test with our developers credentials if you lack time.
Android | iOS | |
---|---|---|
Support SDK | 19+ or 20+ | 9.0+ |
npm install @woosmap/react-native-woosmap
npm install react-native-webview
npm install react-native-uuid
import {
WoosmapView,
MapController,
LatLngBounds,
WoosPadding,
LatLng,
} from '@woosmap/react-native-woosmap';
// ...
<WoosmapView
ref={mapRef}
mapOptions={mapOptions}
wooskey={'Your Woosmap public key'}
click={() => {
console.log('click');
}}
/>
import {
WoosmapView,
MapController,
LatLngBounds,
WoosPadding,
LatLng,
} from '@woosmap/react-native-woosmap';
// ...
<WoosmapView
ref={mapRef}
mapOptions={mapOptions}
wooskey={Platform.OS === 'android'
? 'Woosmap android private key'
: 'Woosmap iOS private key'}
click={() => {
console.log('click');
}}
/>
await mapRef.current?.fitBounds(
LatLngBounds.jsonToObj({
ne: { lat: 48.844437932920535, lng: 2.3743880269761393 },
sw: { lat: 48.854437932920535, lng: 2.3843880269761393 },
}),
WoosPadding.jsonToObj({ top: 2, left: 2, right: 3, bottom: 3 })
);
const result:LatLng = await mapRef.current?.getCenter();
const result: LatLngBounds = await mapRef.current?.getBounds(
WoosPadding.jsonToObj({top: 2, left: 2, right: 3, bottom: 3}));
const result: numeric = (await mapRef.current?.getHeading()) as numeric;
const result: numeric = (await mapRef.current?.getTilt()) as numeric;
const result: numeric = (await mapRef.current?.getZoom()) as numeric;
await mapRef.current?.panBy(20, 10);
await mapRef.current?.panTo(
LatLng.jsonToObj({lat: 48.844437932920535, lng: 2.3743880269761393}),
WoosPadding.jsonToObj({top: 2, left: 2, right: 3, bottom: 3}));
{currentMapSizeInPx} - {padding}
.await mapRef.current?.panToBounds(
LatLngBounds.jsonToObj({
ne: {lat: 48.844437932920535, lng: 2.3743880269761393},
sw: {lat: 48.854437932920535, lng: 2.3843880269761393}
}),
WoosPadding.jsonToObj({top: 2, left: 2, right: 3, bottom: 3}));
await mapRef.current?.setCenter(
LatLng.jsonToObj({lat: 48.844437932920535, lng: 2.3743880269761393}),
WoosPadding.jsonToObj({top: 2, left: 2, right: 3, bottom: 3}));
await mapRef.current?.setHeading(20);
await mapRef.current?.setTilt(5);
await mapRef.current?.setZoom(20);
You can calculate directions and plot by using the WoosmapView.route
method. This method is an interface to the Route endpoint of Woosmap Distance API. This service compute travel distance, time and path for a pair of origin and destination.
You may either display these directions results using custom overlays or use the WoosmapView.setRoute
method to render these results.
import {
WoosmapView,
MapController,
LatLngBounds,
WoosPadding,
LatLng,
DirectionRequest
} from '@woosmap/react-native-woosmap';
// ...
<WoosmapView
ref={mapRef}
mapOptions={mapOptions}
wooskey={'Your Woosmap key'}
routeIndex_changed={(routeInfo) => {
console.log(`route Index changed ${JSON.stringify(routeInfo)}`);
}}}
/>
To display route on map.
mapRef.current?.route(
{
origin: { lat: 48.86288, lng: 2.34946 },
destination: { lat: 52.52457, lng: 13.42347 },
provideRouteAlternatives: true,
travelMode: 'driving',
unitSystem: 'metric',
}
)
.then((result) => {
console.log(JSON.stringify(result));
mapRef.current?.setRoute(result);
});
To clear displayed route on map.
mapRef.current?.setRoute(undefined);
Please check the javascript guide for more implementation. javascript guide
The Woosmap Map provides an overlay layer dedicated to display your assets over the Map.
To ensure maximum readability and navigation, your data are displayed combining tiled images and markers. Tiled images are useful to render large amount of assets or high store density. This view makes the map displayed faster and provide a clean view. Markers are relevant to render branded pictogram after a specified level of zoom (breakpoint).
Assets are retrieved based on the Public API Key attached to your Woosmap Project and set when loading the Map.
const add = function () {
if (storesOverlay === undefined) {
if (mapRef.current) {
storesOverlay = new StoreOverlay(
{
breakPoint: 14,
rules: [
{
color: '#1D1D1D',
type: 'takeaway',
icon: {
url: 'https://images.woosmap.com/starbucks-marker-black.svg',
scaledSize: { height: 40, width: 34 },
},
selectedIcon: {
url: 'https://images.woosmap.com/starbucks-marker-selected.svg',
scaledSize: { height: 50, width: 43 },
},
},
],
default: {
color: '#008a2f',
size: 8,
minSize: 1,
icon: {
url: 'https://images.woosmap.com/starbucks-marker.svg',
scaledSize: { height: 40, width: 34 },
},
selectedIcon: {
url: 'https://images.woosmap.com/starbucks-marker-selected.svg',
scaledSize: { height: 50, width: 43 },
},
},
},
mapRef.current
);
storesOverlay.add();
}
}
};
<WoosmapView
ref={mapRef}
mapOptions={storeOverlayOptions}
wooskey={'Your Woosmap key'}
loaded={() => {
add();
}}
store_unselected={() => {
console.log('store_unselected');
}}
store_selected={() => {
console.log('store_selected');
}}
/>
To utilize local images for the store icon, in place of the web Image, simply load a local image via StoreOverlay.image
and configure it accordingly.
Promise.all([
StoreOverlay.image(require('../starbucks-marker-black.svg')),
StoreOverlay.image(require('../starbucks-marker-selected.svg')),
StoreOverlay.image(require('../starbucks-marker.svg')),
),
]).then((images) => {
if (mapRef.current) {
storesOverlay = new StoreOverlay(
{
breakPoint: 14,
rules: [
{
color: '#1D1D1D',
type: 'takeaway',
icon: {
url: images[0],
scaledSize: { height: 40, width: 34 },
},
selectedIcon: {
url: images[1],
scaledSize: { height: 50, width: 43 },
},
},
],
default: {
color: '#008a2f',
size: 8,
minSize: 1,
icon: {
url: images[2],
scaledSize: { height: 40, width: 34 },
},
selectedIcon: {
url: images[1],
scaledSize: { height: 50, width: 43 },
},
},
},
mapRef.current
);
storesOverlay.add();
}
});
Please check the javascript guide for more implementation. javascript guide
Display markers to identify locations on Woosmap Map. Markers are used to display clickable/draggable icons on the map. You can attach a wide variety of event listeners to control user interaction.
let marker: Marker | null;
const mapOptions: Object = {
center: { lat: 51.515, lng: -0.13 },
zoom: 10,
};
const add = function () {
if (marker === undefined) {
if (mapRef.current) {
marker = new Marker(
{
position: { lat: 51.522, lng: -0.13 },
icon: {
url: 'https://images.woosmap.com/dot-marker.png',
scaledSize: { height: 64, width: 46 },
},
},
mapRef.current,
{
click: () => {
console.log('marker click');
},
}
);
marker.add();
}
}
};
<WoosmapView
ref={mapRef}
mapOptions={mapOptions}
wooskey={'Your Woosmap key'}
loaded={() => {
add();
}}
/>
Please check the javascript guide for more implementation. javascript guide
You need to integrate the Map Js library to use the Indoor Renderer and render indoor tiles into your application.
API Key Map JS API requires authorization via API Key for initialization and API calls. You can obtain it by following this steps.
const indoorRendererConfiguration: IndoorRendererOptions = {
centerMap: true,
defaultFloor: 3,
};
const indoorWidgetConfiguration: IndoorWidgetOptions = {
units: 'metric',
ui: {
primaryColor: '#318276',
secondaryColor: '#004651',
},
};
<WoosmapView
ref={mapRef}
wooskey={'Your Woosmap key'}
indoorRendererConfiguration={indoorRendererConfiguration}
indoorWidgetConfiguration={indoorWidgetConfiguration}
widget={true}
activateIndoorProduct={true}
defaultIndoorVenueKey={'mtp'}
loaded={() => {
//add();
}}
indoor_venue_loaded={(info) => {
console.log(JSON.stringify(info));
}}
indoor_level_changed={(info) => {
console.log('Level changed ' + JSON.stringify(info));
}}
indoor_feature_selected={(info) => {
console.log('Feature selected ' + JSON.stringify(info));
}}
indoor_user_location={(info) => {
console.log('User location ' + JSON.stringify(info));
}}
indoor_highlight_step={(info) => {
console.log('Step info ' + JSON.stringify(info));
}}
indoor_navigation_started={() => {
console.log('Navigation Started');
}}
indoor_navigation_exited={() => {
console.log('Navigation ended');
mapRef.current?.clearDirections();
}}
/>
mapRef.current?.directions.
If you know lat/lng of POI
mapRef.current?
.directions(
{
venueId: activeVenue,
origin: { lat: 43.60664187325, lng: 3.921814671575 },
originLevel: 3,
destination: { lat: 43.60665215333, lng: 3.921680093435 },
destinationLevel: 3,
language: 'en',
units: 'metric',
mode: 'wheelchair',
}
)
.then((result) => {
if (result) {
mapRef.current?.setDirections(result);
} else {
Alert.alert(`Indoor Direction`, `No Route found`);
}
});
If you know id of POI
mapRef.current?
.directions(
{
venueId: activeVenue,
originId: 614309,
destinationId: 614165,
language: 'en',
units: 'metric',
mode: 'wheelchair',
}
)
.then((result) => {
if (result) {
mapRef.current?.setDirections(result);
} else {
Alert.alert(`Indoor Direction`, `No Route found`);
}
});
If you know reference of POI
mapRef.current?
.directions(
{
venueId: activeVenue,
originId: 'ref:meeting001',
destinationId: 'ref:tropiques',
language: 'en',
units: 'metric',
mode: 'wheelchair',
}
)
.then((result) => {
if (result) {
mapRef.current?.setDirections(result);
} else {
Alert.alert(`Indoor Direction`, `No Route found`);
}
});
mapRef.current?
.directions(
{
venueId: activeVenue,
origin: { lat: 43.60664187325, lng: 3.921814671575 },
originLevel: 3,
destination: { lat: 43.60665215333, lng: 3.921680093435 },
destinationLevel: 3,
language: 'en',
units: 'metric',
mode: 'wheelchair',
waypoints: ['ref:meeting001', 'ref:tropiques'],
}
)
.then((result) => {
if (result) {
mapRef.current?.setDirections(result);
} else {
Alert.alert(`Indoor Direction`, `No Route found`);
}
});
To initiate navigation experience you need to call following methods of the MapController
object in the same order as given below.
directions
- As explained in the earlier section directions
method will find the route between two points inside an indoor venue. This method returns a route
object upon it's completion.
setDirections
- This method will plot the given route
on the map. You can pass the route
object returned by directions
method.
startNavigation
- This method will initiate the "Turn By Turn" navigation experience.
mapRef.current?.startNavigation();
exitNavigation
- This method will result in the exit from "Turn By Turn" navigation mode.mapRef.current?.exitNavigation();
clearDirections
- Removes the plotted path on the map. If the navigation experience is started then it first exits from the navigation then removes the path.mapRef.current?.clearDirections();
Please note that setDirections
method will no longer trigger navigation. Method startNavigation
should explicitly be called to initiate the navigation.
Please check the javascript guide for more implementation. javascript guide for Indoor Renderer
The Woosmap API is a RESTful API built on HTTP. It has predictable resource URLs. It returns HTTP response codes to indicate errors. It also accepts and returns JSON in the HTTP body.
Woosmap Localities API is a web service that returns a great amount of geographical places in response to an HTTP request. Among others are city names, postal codes, suburbs, addresses or airports. Request is done over HTTPS using GET. Response is formatted as JSON. You must specify a key in your request, included as the value of a key parameter for your public key or private_key for your private key. This key identifies your application for purposes of quota management.
Autocomplete on worldwide suggestions for a for text-based geographic searches. It can match on full words as well as substrings. You can therefore send queries as the user types, to provide on-the-fly city names, postal codes or suburb name suggestions.
Request parameters
input
: The text string on which to search, for example: "london"types
: "locality" "postal_code" "address" "admin_level" "country" "airport" "train_station" "metro_station" "shopping" "museum" "tourist_attraction" "amusement_park" "art_gallery" "zoo"components
: A grouping of places to which you would like to restrict your results. Components can be used to filter over countries.language
: The language code, using ISO 3166-1 Alpha-2 country codes, indicating in which language the results should be returnedlocation
: This parameter is used to add a bias to the autocomplete feature. The location defines the point around which to retrieve results in priority.radius
: This parameter may be used in addition to the location parameter to define the distance in meters within which the API will return results in priority.data
: Two values for this parameter: standard or advanced. By default, if the parameter is not defined, value is set as standard. The advanced value opens suggestions to worldwide postal codes in addition to postal codes for Western Europe. A dedicated option subject to specific billing on your license is needed to use this parameter.extended
:If set, this parameter allows a refined search over locality names that bears the same postal code.customDescription
: This parameter allows to choose the description format for all or some of the suggestion types selected. The custom formats are described as follows (available fields depend on the returned type): custom_description=type_A:"{field_1}, {field_2}, [...]"|type_B:"{field_1}, {field_2}, [...]"let api = new WoosmapApi({ publicKey: <<public key from woosmap console>> }); //or new WoosmapApi({ privateKey: <<private key from woosmap console>> });
let apiInput: LocalitiesAutocompleteRequest = { input: 'landon' };
api.Localities.autocomplete(apiInput)
.then((apiResult) => {
console.log(JSON.stringify(apiResult, undefined, 4));
})
.catch((error) => {
console.log(
`${error.message} \n\n ${JSON.stringify(error.cause, undefined, 4)}`
);
});
Provides details of an autocomplete suggestion (using the suggestion’s publicId
).
Request parameters
publicId
: A textual identifier that uniquely identifies a locality, returned from a Localities Autocomplete.language
: The language code, using ISO 3166-1 Alpha-2 country codes, indicating in which language the results should be returnedfields
: Used to limit the returning fields, default it is geometrycountryCodeFormat
: To specify the format for the short country code expected to be returned in the address_components field (default is alpha3).let api = new WoosmapApi({ publicKey: <<public key from woosmap console>> }); //or new WoosmapApi({ privateKey: <<private key from woosmap console>> });
let apiInput: LocalitiesDetailsRequest = { publicId: '123456' };
api.Localities.autocomplete(apiInput)
.then((apiResult) => {
console.log(JSON.stringify(apiResult, undefined, 4));
})
.catch((error) => {
console.log(
`${error.message} \n\n ${JSON.stringify(error.cause, undefined, 4)}`
);
});
Provides details for an address or a geographic position. Either parameter address
or latlng
is required.
Request parameters
address
: The input string to geocode. Can represent an address, a street, a locality or a postal code.latLng
: The latlng parameter is used for reverse geocoding, it’s required if the address parameter is missing.components
: A grouping of places to which you would like to restrict your results. Components can be used to filter over countries. Countries must be passed as an ISO 3166-1 Alpha-2 or Alpha-3 compatible country code.language
: The language code, using ISO 3166-1 Alpha-2 country codes, indicating in which language the results should be returnedfields
: Used to limit the returning fieldsdata
: Two values for this parameter: standard or advanced. By default, if the parameter is not defined, value is set as standard. The advanced value opens suggestions to worldwide postal codes in addition to postal codes for Western Europe. A dedicated option subject to specific billing on your license is needed to use this parameter.countryCodeFormat
: To specify the format for the short country code expected to be returned in the address_components field.let api = new WoosmapApi({ publicKey: <<public key from woosmap console>> }); //or new WoosmapApi({ privateKey: <<private key from woosmap console>> });
let apiInput: LocalitiesGeocodeRequest = {address: '123 gold gym'};
api.Localities.geocode(apiInput)
.then((apiResult) => {
console.log(JSON.stringify(apiResult, undefined, 4));
})
.catch((error) => {
console.log(
`${error.message} \n\n ${JSON.stringify(error.cause, undefined, 4)}`
);
});
Stores Search API lets you search among your own Points of Interest. Find stores by geography, by attributes or by autocomplete on store names.
Autocomplete on localizedNames with highlighted results on asset name. Use the field localized in your query parameter to search for localized names.
Request parameters
query
: Search query combining one or more search clauses. Example: query=name:'My cool store'|type:'click_and_collect'
language
: The preferred language to match against name (defaults on Accept-Language header)limit
: You can then request stores by page using limit
parameterslet api = new WoosmapApi({ publicKey: <<public key from woosmap console>> }); //or new WoosmapApi({ privateKey: <<private key from woosmap console>> });
let apiInput: StoresAutocompleteRequest = {query:'name:\'My cool store\'|type:\'click_and_collect\''};
api.Stores.autocomplete(apiInput)
.then((apiResult) => {
console.log(JSON.stringify(apiResult, undefined, 4));
})
.catch((error) => {
console.log(
`${error.message} \n\n ${JSON.stringify(error.cause, undefined, 4)}`
);
});
Used to retrieve assets from query.
Request parameters
query
: Search query combining one or more search clauses. Example: query=name:'My cool store'|type:'click_and_collect'
latLng
: To bias the results around a specific latlngradius
: To bias the results within a given circular area.polyline
: Find stores nearby an encoded polyline and inside a defined radius.storesByPage
: You can then request stores by page using page
and storesByPage
parameters (Default is 100, max is 300).page
: Page number when accessing paginated storeszone
: whether to search for stores intersecting a zonelet api = new WoosmapApi({ publicKey: <<public key from woosmap console>> }); //or new WoosmapApi({ privateKey: <<private key from woosmap console>> });
let apiInput: StoresSearchRequest = {query:'idStore:31625406'};
api.Stores.search(apiInput)
.then((apiResult) => {
console.log(JSON.stringify(apiResult, undefined, 4));
})
.catch((error) => {
console.log(
`${error.message} \n\n ${JSON.stringify(error.cause, undefined, 4)}`
);
});
MIT
FAQs
The Woosmap react native SDK is a library that use to embed interactive maps directly into your application. You can also add stores overlay to the map to call out points of interest and get relative information.
The npm package @woosmap/react-native-woosmap receives a total of 5 weekly downloads. As such, @woosmap/react-native-woosmap popularity was classified as not popular.
We found that @woosmap/react-native-woosmap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.