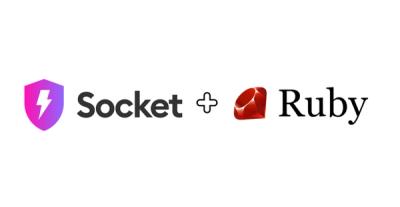
Product
Introducing Ruby Support in Socket
Socket is launching Ruby support for all users. Enhance your Rails projects with AI-powered security scans for vulnerabilities and supply chain threats. Now in Beta!
@zag-js/anatomy
Advanced tools
@zag-js/anatomy is a utility library for creating and managing component anatomy in a consistent and reusable way. It helps in defining the structure and parts of a component, making it easier to manage and style complex UI components.
Define Component Anatomy
This feature allows you to define the different parts of a component. In this example, a button component is defined with root, icon, and label parts.
const { defineParts } = require('@zag-js/anatomy');
const buttonAnatomy = defineParts({
root: 'button',
icon: 'button__icon',
label: 'button__label'
});
console.log(buttonAnatomy.parts); // { root: 'button', icon: 'button__icon', label: 'button__label' }
Get Part Selectors
This feature allows you to get the CSS selectors for each part of the component. This is useful for styling the component parts consistently.
const { defineParts } = require('@zag-js/anatomy');
const buttonAnatomy = defineParts({
root: 'button',
icon: 'button__icon',
label: 'button__label'
});
const selectors = buttonAnatomy.selectors;
console.log(selectors.root); // .button
console.log(selectors.icon); // .button__icon
console.log(selectors.label); // .button__label
Extend Component Anatomy
This feature allows you to extend an existing component anatomy with additional parts. In this example, the button anatomy is extended to include an iconWrapper part.
const { defineParts } = require('@zag-js/anatomy');
const buttonAnatomy = defineParts({
root: 'button',
icon: 'button__icon',
label: 'button__label'
});
const iconButtonAnatomy = buttonAnatomy.extend({
iconWrapper: 'button__icon-wrapper'
});
console.log(iconButtonAnatomy.parts); // { root: 'button', icon: 'button__icon', label: 'button__label', iconWrapper: 'button__icon-wrapper' }
styled-components is a library for React and React Native that allows you to use component-level styles in your application. It provides a way to style components using tagged template literals. While @zag-js/anatomy focuses on defining and managing component parts, styled-components focuses on styling components directly.
Emotion is a library designed for writing CSS styles with JavaScript. It provides powerful and flexible tools for styling applications. Similar to styled-components, Emotion focuses on styling, whereas @zag-js/anatomy focuses on defining the structure and parts of components.
FAQs
Unknown package
The npm package @zag-js/anatomy receives a total of 94,737 weekly downloads. As such, @zag-js/anatomy popularity was classified as popular.
We found that @zag-js/anatomy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket is launching Ruby support for all users. Enhance your Rails projects with AI-powered security scans for vulnerabilities and supply chain threats. Now in Beta!
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.