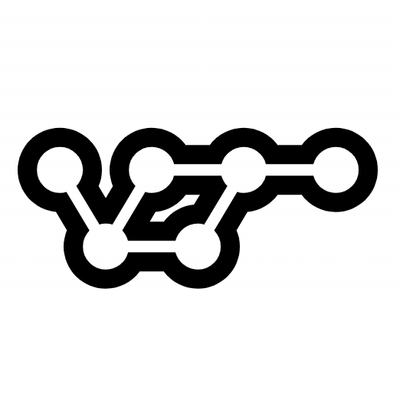
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
adonisjs-jobs
Advanced tools
Job processing for AdonisJS v6 using BullMQ
This package is available in the npm registry.
pnpm install adonisjs-jobs
Next, configure the package by running the following command.
node ace configure adonisjs-jobs
You can create a new job by running the following command.
node ace jobs:make SendEmail
First, you need to start the jobs listener, you can spawn multiple listeners to process jobs concurrently.
node ace jobs:listen # default queue from env `REDIS_QUEUE`
node ace jobs:listen --queue=high
node ace jobs:listen --queue=high --queue=medium
node ace jobs:listen --queue=high,medium,low
node ace jobs:listen --queue=high --concurrency=3
Dispatching jobs is as simple as importing the job class and calling
import SendEmail from 'path/to/jobs/send_email.js'
await SendEmail.dispatch({ ... })
await SendEmail.dispatch({ ... }, { // for more job options check https://docs.bullmq.io/
attempts: 3,
delay: 1000,
})
update your package.json
and tsconfig.json
to use import aliases
package.json
{
"imports": {
"#jobs/*": "./app/jobs/*.js"
}
}
tsconfig.json
{
"compilerOptions": {
"paths": {
"#jobs/*": ["./app/jobs/*.js"]
}
}
}
import SendEmail from '#jobs/send_email.js'
await SendEmail.dispatch({ ... })
You can view the jobs dashboard by adding the following route to your start/routes.ts
file.
import router from '@adonisjs/core/services/router'
router.jobs() // default is /jobs
// or
router.jobs('/my-jobs-dashboard')
router.jobs()
returns a route group, you can add middleware to the group
router.jobs().use(
middleware.auth({
guards: ['basicAuth'],
})
)
import { dispatch } from 'adonisjs-jobs/services/main'
await dispatch(async () => {
const { default: User } = await import('#models/user')
console.log(await User.query().count('*'))
})
FAQs
Job processing for AdonisJS
The npm package adonisjs-jobs receives a total of 128 weekly downloads. As such, adonisjs-jobs popularity was classified as not popular.
We found that adonisjs-jobs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.