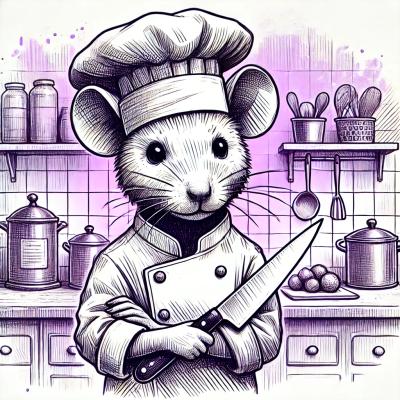
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Compile and run Constraint Handling Rules (CHR) in JavaScript
npm install chr
If you install CHR.js via npm it will create a new chrjs
executable:
$ chrjs /path/to/your.chr > /created/constrainthandler.js
If no path is specified, chrjs
reads from stdin.
The CHR.js module can be used programmatically:
var chrjs = require('chr')
var code = chrjs.transform('a ==> b')
chrjs.transformFile('path.chr', function(err, code) {
console.log(code)
})
Consider the following CHR source code, which generates all fibonacci numbers upto a given index N
as constraints of the form fib(Number,Value)
:
upto(N), fib(A,AV), fib(B,BV) ==> B === A+1, B < N | fib(B+1,AV+BV);
The CHR source code can be compiled to JavaScript by the use of the chrjs
command:
$ echo 'upto(N), fib(A,AV), fib(B,BV) ==> B === A+1, B < N | fib(B+1,AV+BV);' | chrjs > fib.js
The generated JavaScript code has references to chr/runtime
components, so make sure it is available. Open a REPL to play with the generated fib.js
:
$ node
> var konsole = require('chr/console') // for graphical representation
> var CHR = require('./fib.js') // load the generated source
> CHR.fib(1,1) // the first fibonacci is 0
> CHR.fib(2,1) // the second is 1
> konsole(CHR.Store) // print the content of the
┌────────────┐ // constraint store
│ Constraint │
├────────────┤
│ fib(1,1) │
├────────────┤
│ fib(2,1) │
└────────────┘
> CHR.upto(5) // generate the first 5 fibs
> konsole(CHR.Store) // print the content of the
┌────────────┐ // constraint store again
│ Constraint │
├────────────┤
│ fib(1,1) │
├────────────┤
│ fib(2,1) │
├────────────┤
│ upto(5) │
├────────────┤
│ fib(3,2) │
├────────────┤
│ fib(4,3) │
├────────────┤
│ fib(5,5) │
└────────────┘
> CHR.fib(6,8).fib(7,13) // constraints allow chaining
> CHR.Store.reset() // clear the constraint store
More example CHR scripts are provided in the project's /examples
directory.
The implementation is based on the compilation schema presented in the paper CHR for imperative host languages (2008; Peter Van Weert, Pieter Wuille, Tom Schrijvers, Bart Demoen). As of yet basically none of the mentioned optimizations have been implemented.
A list of open points for improving can be found in the wiki.
FAQs
Interpreter for Constraint Handling Rules (CHR) in JavaScript
The npm package chr receives a total of 73 weekly downloads. As such, chr popularity was classified as not popular.
We found that chr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.