cli-qa
Command-line Questions & Answers. Supports color formatting, lists, yes/no confirmations.
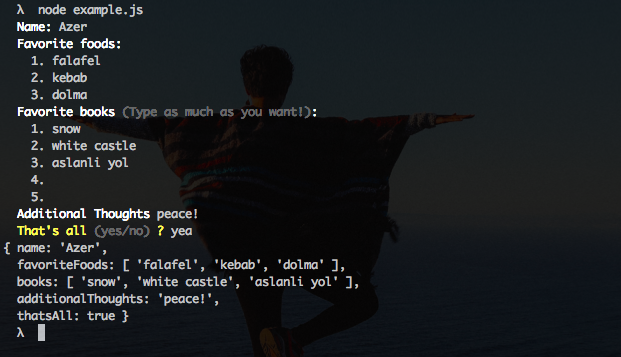
Install
$ npm install cli-qa
Usage
var QA = require('cli-qa')
var questions = [
"{bold}Name:{reset}",
{ title: "Username:", default: process.env.USER },
{ key: "email", title: "E-Mail:", validate: { email: true } },
{ title: "{bold}Favorite foods:{reset}", list: 3 },
{ key: "books", title: "{bold}Favorite books {grey}(Type as much as you want!){reset}{bold}:{reset}", list: true },
{ title: "{yellow}Favorite {green}Fruits{reset}:", commaList: true },
{ key: 'thatsAll', title: "{yellow}{bold}That's all {grey}(yes/no) {yellow}?{reset}", bool: true },
];
QA(questions, function (answers) {
console.log(answers)
})
Styling
Title and description fields can be styled with style-format interface. Here is an example;
QA([{ title: "{green}Yes{reset}/{red}No{reset}?", bool: true }], function (answers) {
answers[yesNo]
})
Reference
The list of available options for each question:
- key: The key that will be used in the answers object. If not specified, it'll be auto-generated.
- title: Title of the question. styling accepted.
- list: Specify "true" if a numerical list is expected as an answer.
- commaList: Specify "true" if a comma-separated list is expected as an answer.
- bool: Yes/no question? true/false
- default: Default value if answer is empty.
- validate: Specify requirements for validating the user input.