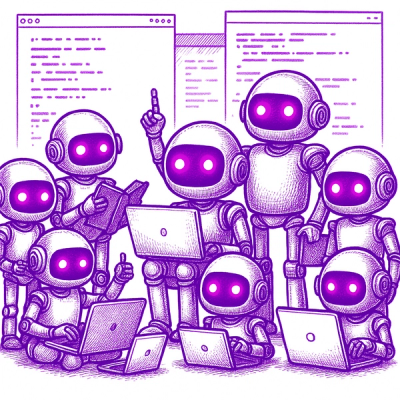
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
color-string
Advanced tools
The color-string npm package is a library for parsing and generating CSS color strings. It allows users to work with various color formats, convert between them, and manipulate color values.
Parsing Color Strings
This feature allows you to parse CSS color strings into an array of RGBA values.
const colorString = require('color-string');
const color = colorString.get.rgb('rgba(255, 204, 0, 0.7)');
console.log(color); // [255, 204, 0, 0.7]
Generating Color Strings
This feature enables you to generate CSS color strings from RGB(A) or HSL(A) values.
const colorString = require('color-string');
const rgbString = colorString.to.rgb([255, 204, 0]);
console.log(rgbString); // 'rgb(255, 204, 0)'
Converting Color Models
This feature allows you to convert color values between different color models, such as RGB to HSL.
const colorString = require('color-string');
const hslString = colorString.to.hsl([255, 204, 0]);
console.log(hslString); // 'hsl(45, 100%, 50%)'
The 'color' package is a powerful library for color conversion and manipulation. It supports chaining and has more manipulation functions compared to color-string.
Chroma.js is a comprehensive library for all kinds of color conversions and color scales. It offers more advanced features like color scales, interpolation, and blending compared to color-string.
TinyColor is a fast, small color manipulation and conversion library. It provides additional functionalities like color readability and random color generation, which are not present in color-string.
library for parsing and generating CSS color strings.
npm install color-string
colorString.get('#FFF') // {model: 'rgb', value: [255, 255, 255, 1]}
colorString.get('#FFFA') // {model: 'rgb', value: [255, 255, 255, 0.67]}
colorString.get('#FFFFFFAA') // {model: 'rgb', value: [255, 255, 255, 0.67]}
colorString.get('hsl(360, 100%, 50%)') // {model: 'hsl', value: [0, 100, 50, 1]}
colorString.get('hsl(360 100% 50%)') // {model: 'hsl', value: [0, 100, 50, 1]}
colorString.get('hwb(60, 3%, 60%)') // {model: 'hwb', value: [60, 3, 60, 1]}
colorString.get.rgb('#FFF') // [255, 255, 255, 1]
colorString.get.rgb('blue') // [0, 0, 255, 1]
colorString.get.rgb('rgba(200, 60, 60, 0.3)') // [200, 60, 60, 0.3]
colorString.get.rgb('rgba(200 60 60 / 0.3)') // [200, 60, 60, 0.3]
colorString.get.rgb('rgba(200 60 60 / 30%)') // [200, 60, 60, 0.3]
colorString.get.rgb('rgb(200, 200, 200)') // [200, 200, 200, 1]
colorString.get.rgb('rgb(200 200 200)') // [200, 200, 200, 1]
colorString.get.hsl('hsl(360, 100%, 50%)') // [0, 100, 50, 1]
colorString.get.hsl('hsl(360 100% 50%)') // [0, 100, 50, 1]
colorString.get.hsl('hsla(360, 60%, 50%, 0.4)') // [0, 60, 50, 0.4]
colorString.get.hsl('hsl(360 60% 50% / 0.4)') // [0, 60, 50, 0.4]
colorString.get.hwb('hwb(60, 3%, 60%)') // [60, 3, 60, 1]
colorString.get.hwb('hwb(60, 3%, 60%, 0.6)') // [60, 3, 60, 0.6]
colorString.get.rgb('invalid color string') // null
colorString.to.hex(255, 255, 255) // "#FFFFFF"
colorString.to.hex(0, 0, 255, 0.4) // "#0000FF66"
colorString.to.hex(0, 0, 255, 0.4) // "#0000FF66"
colorString.to.rgb(255, 255, 255) // "rgb(255, 255, 255)"
colorString.to.rgb(0, 0, 255, 0.4) // "rgba(0, 0, 255, 0.4)"
colorString.to.rgb(0, 0, 255, 0.4) // "rgba(0, 0, 255, 0.4)"
colorString.to.rgb.percent(0, 0, 255) // "rgb(0%, 0%, 100%)"
colorString.to.keyword(255, 255, 0) // "yellow"
colorString.to.hsl(360, 100, 100) // "hsl(360, 100%, 100%)"
colorString.to.hwb(50, 3, 15) // "hwb(50, 3%, 15%)"
MIT
FAQs
Parser and generator for CSS color strings
The npm package color-string receives a total of 21,577,357 weekly downloads. As such, color-string popularity was classified as popular.
We found that color-string demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.