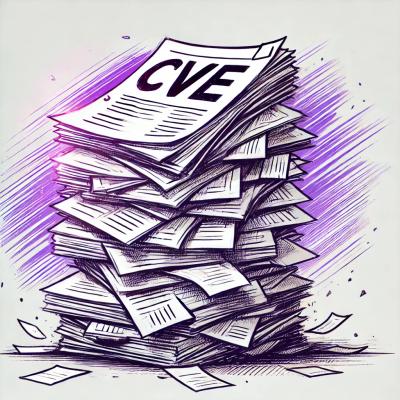
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Simple config loading with support for injecting environment variables.
npm i confugu
First, set up a yaml
file to hold your config values.
logLevel: debug
port: ${ HTTP_PORT } # use 'HTTP_PORT' environment variable
Next, just pass the path to your config file to the asynchronous load
function.
const confugu = require('confugu')
;(async () => {
const config = await confugu.load('path/to/your/config')
console.log(config.logLevel) // prints 'debug'
console.log(config.port) // prints whatever is the value of process.env.HTTP_PORT
})()
Or you can load your config synchronously using loadSync
:
const confugu = require('confugu')
const config = confugu.loadSync('path/to/your/config')
console.log(config.logLevel) // prints 'debug'
console.log(config.port) // prints whatever is the value of process.env.HTTP_PORT
Fetching a nested property safely without having to check existence all the way
down the property chain is also supported using the get
function:
const confugu = require('confugu')
const config = confugu.loadSync('path/to/your/config')
console.log(config.get('logLevel')) // prints the value of the 'logLevel' property
console.log(config.get('db.password')) // prints the value of the 'db.password' property
console.log(config.get('invalid.nested.property')) // Invalid properties are "undefined"
npm test
FAQs
Awesome configs
The npm package confugu receives a total of 4 weekly downloads. As such, confugu popularity was classified as not popular.
We found that confugu demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.