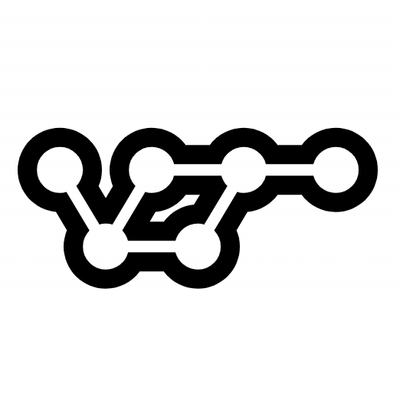
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
create-huck
Advanced tools
The following examples show how to set up a typical Layout with Vite providing hot-reloading.
See a complete layout example in the huck-examples repo.
or
See a complete podlet example in the huck-examples repo.
pnpm add -D svelte @sveltejs/vite-plugin-svelte
Ensure the following content in vite.config.js
import { svelte } from '@sveltejs/vite-plugin-svelte'
export default defineConfig({
//...
plugins: [svelte()],
//...
})
Ensure the following content in client/main.js
import App from './App.svelte'
const rootEl = document.querySelector('#app')
new App({ target: rootEl })
and create the client/App.svelte
file
<script>
let count = 0
const handleClick = () => count += 1
</script>
<button on:click={handleClick}>
Count is {count}
</button>
See a complete example in the huck-examples repo.
pnpm add -D vue @vitejs/plugin-vue
Ensure the following content in vite.config.js
import vue from '@vitejs/plugin-react'
export default defineConfig({
//...
plugins: [vue()],
//...
})
Ensure the following content in client/main.js
import { createApp } from 'vue'
import App from './App.vue'
const rootEl = document.querySelector('#app')
createApp(App).mount(rootEl)
and create the client/App.vue
file
<script setup>
import { ref } from 'vue'
const count = ref(0)
const handleClick = () => count.value += 1
</script>
<template>
<button @click="handleClick">
Count is {{ count }}
</button>
</template>
See a complete example in the huck-examples repo.
pnpm add -D react react-dom @vitejs/plugin-react
Ensure the following content in vite.config.js
import react from '@vitejs/plugin-react'
export default defineConfig({
//...
plugins: [react()],
//...
})
Ensure the following content in client/main.jsx
, and rename any references to main.js
into main.jsx
.
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
const rootEl = document.querySelector('#app')
ReactDOM.createRoot(rootEl).render(
<React.StrictMode>
<App />
</React.StrictMode>,
)
and create the client/App.jsx
file
import { useState } from 'react'
function App() {
const [count, setCount] = useState(0)
return (
<>
<button onClick={() => setCount((count) => count + 1)}>
Count is {count}
</button>
</>
)
}
export default App
The server-emitted HTML must be wrapped by a helper in Vite. The Vite server can be referenced on server.vite
, and server
is available as an argument to the render
hook.
const rootEl = await server.vite.transformIndexHtml(req.url, `<div class="page-container" id="app" ${config}></div>`)
reply.podiumSend(`
${$header}
${rootEl}
${$footer}
`)
FAQs
Unknown package
The npm package create-huck receives a total of 2 weekly downloads. As such, create-huck popularity was classified as not popular.
We found that create-huck demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.