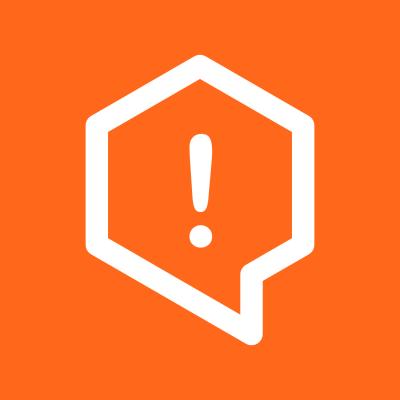
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
cypher-stream
Advanced tools

Neo4j cypher queries as node object streams.
1.0.0-alpha is Powered by Bolt™. Updated documentation is forthcoming. For the brave, check the source, tests, and go wild.
The API is largely unchanged, with the exception of authentication and some configuration options which no longer make sense in the Bolt world (i.e. http headers).
Performance should be significantly improved, given that the library no longer has to stream-parse HTTP JSON responses.
TODO:
npm install cypher-stream
Or, for Bolt
npm install cypher-stream@1.0.0-alpha
var cypher = require('cypher-stream')('bolt://localhost', 'username', 'password');
cypher('match (user:User) return user')
.on('data', function (result){
console.log(result.user.first_name);
})
.on('end', function() {
console.log('all done');
})
;
var cypher = require('cypher-stream')('bolt://localhost', 'username', 'password');
var should = require('should');
it('handles errors', function (done) {
var errored = false;
cypher('invalid query')
.on('error', function (error) {
errored = true;
String(error).should.equal('Error: Query failure: Invalid input \'i\': expected SingleStatement (line 1, column 1)\n"invalid query"\n ^');
error.neo4j.exception.should.equal('SyntaxException');
error.neo4j.stacktrace.should.be.an.array;
error.neo4j.statusCode.should.equal(400);
})
.on('end', function() {
errored.should.be.true;
done();
})
.resume() // need to manually start it since we have no on('data')
;
});
Transactions are duplex streams that allow you to write query statements then commit or roll back the written queries.
Transactions have three methods: write
, commit
, and rollback
, which add queries and commit or rollback the queue respectively.
var transaction = cypher.transaction(options)
transaction.write(query_statement);
A query_statement
can either be a string or a query statement object. A query statement object consists of a statement
property and an optional parameters
property. Additionally, you can pass an array of either.
The following are all valid options:
var transaction = cypher.transaction();
transaction.write('match (n:User) return n');
transaction.write({ statement: 'match (n:User) return n' });
transaction.write({
statement : 'match (n:User) where n.first_name = {first_name} return n',
parameters : { first_name: "Bob" }
});
transaction.write([
{
statement : 'match (n:User) where n.first_name = {first_name} return n',
parameters : { first_name: "Bob" }
},
'match (n:User) where n.first_name = {first_name} return n'
]);
transaction.commit();
transaction.rollback();
Alternatively, a query statement may also contain a commit
or rollback
property instead of calling commit()
or rollback()
directly.
transaction.write({ statement: 'match (n:User) return n', commit: true });
transaction.write({
statement : 'match (n:User) where n.first_name = {first_name} return n',
parameters : { first_name: "Bob" },
commit : true
});
To get a stream per statement, just pass a callback
function with the statement object. This works for regular cypher calls and transactions.
var results = 0;
var calls = 0;
var ended = 0;
var query = 'match (n:Test) return n limit 2';
function callback(stream) {
stream
.on('data', function (result) {
result.should.eql({ n: { test: true } });
results++;
})
.on('end', function () {
ended++;
})
;
calls++;
}
var statement = { statement: query, callback: callback };
cypher([ statement, statement ]).on('end', function () {
calls.should.equal(2);
ended.should.equal(2);
results.should.equal(4);
done();
}).resume();
var results = 0;
var calls = 0;
var ended = 0;
var query = 'match (n:Test) return n limit 2';
function callback(stream) {
stream
.on('data', function (result) {
result.should.eql({ n: { test: true } });
results++;
})
.on('end', function () {
ended++;
})
;
calls++;
}
var statement = { statement: query, callback: callback };
var transaction = cypher.transaction();
transaction.write(statement);
transaction.write(statement);
transaction.commit();
transaction.resume();
transaction.on('end', function() {
calls.should.equal(2);
ended.should.equal(2);
results.should.equal(4);
done();
});
FAQs
Streams cypher query results in a clean format
The npm package cypher-stream receives a total of 25 weekly downloads. As such, cypher-stream popularity was classified as not popular.
We found that cypher-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.