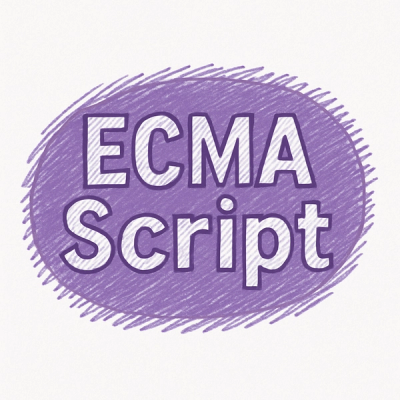
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
deep-object-diff
Advanced tools
Deep diffs two objects, including nested structures of arrays and objects, and return the difference.
The deep-object-diff npm package is designed to help developers identify differences between two JavaScript objects. It provides detailed insights into what has changed, including additions, deletions, and updates, making it particularly useful for tasks such as tracking state changes or debugging.
Deep Diff
Calculates the deep difference between two objects, returning an object that represents the changes. In this example, it would output `{ b: 3 }`, indicating that the value of `b` has changed from `2` to `3`.
const { diff } = require('deep-object-diff');
const original = { a: 1, b: 2 };
const updated = { a: 1, b: 3 };
console.log(diff(original, updated));
Detailed Diff
Provides a detailed difference between two objects, categorizing the changes into added, deleted, and updated properties. The output for this example would be an object with `added`, `deleted`, and `updated` keys, showing that `b` was updated, `c` was added, and no keys were deleted.
const { detailedDiff } = require('deep-object-diff');
const original = { a: 1, b: 2 };
const updated = { a: 1, b: 3, c: 4 };
console.log(detailedDiff(original, updated));
Deleted Diff
Identifies properties that have been deleted from the original object. In this case, the output would be `{ c: 3 }`, indicating that `c` was present in the original object but is missing in the updated object.
const { deletedDiff } = require('deep-object-diff');
const original = { a: 1, b: 2, c: 3 };
const updated = { a: 1, b: 2 };
console.log(deletedDiff(original, updated));
Lodash's isEqual method provides a way to perform deep equality comparisons between two values. While it doesn't offer a detailed breakdown of differences like deep-object-diff, it's useful for simply checking if two objects are equal.
This package offers a fast deep equality check between two objects. Similar to lodash.isequal, it doesn't provide detailed differences but is optimized for performance when comparing complex objects.
Deep-diff is another package that, like deep-object-diff, is designed to identify differences between two JavaScript objects. It offers a range of features for detecting changes, including detailed diffs, but its API and performance characteristics may differ.
❄️
Deep diff two JavaScript Objects
A small library that can deep diff two JavaScript Objects, including nested structures of arrays and objects.
yarn add deep-object-diff
npm i --save deep-object-diff
diff(originalObj, updatedObj)
returns the difference of the original and updated objects
addedDiff(original, updatedObj)
returns only the values added to the updated object
deletedDiff(original, updatedObj)
returns only the values deleted in the updated object
updatedDiff(original, updatedObj)
returns only the values that have been changed in the updated object
detailedDiff(original, updatedObj)
returns an object with the added, deleted and updated differences
import { diff, addedDiff, deletedDiff, updatedDiff, detailedDiff } from 'deep-object-diff';
diff
:const lhs = {
foo: {
bar: {
a: ['a', 'b'],
b: 2,
c: ['x', 'y'],
e: 100 // deleted
}
},
buzz: 'world'
};
const rhs = {
foo: {
bar: {
a: ['a'], // index 1 ('b') deleted
b: 2, // unchanged
c: ['x', 'y', 'z'], // 'z' added
d: 'Hello, world!' // added
}
},
buzz: 'fizz' // updated
};
console.log(diff(lhs, rhs)); // =>
/*
{
foo: {
bar: {
a: {
'1': undefined
},
c: {
'2': 'z'
},
d: 'Hello, world!',
e: undefined
}
},
buzz: 'fizz'
}
*/
addedDiff
:const lhs = {
foo: {
bar: {
a: ['a', 'b'],
b: 2,
c: ['x', 'y'],
e: 100 // deleted
}
},
buzz: 'world'
};
const rhs = {
foo: {
bar: {
a: ['a'], // index 1 ('b') deleted
b: 2, // unchanged
c: ['x', 'y', 'z'], // 'z' added
d: 'Hello, world!' // added
}
},
buzz: 'fizz' // updated
};
console.log(addedDiff(lhs, rhs));
/*
{
foo: {
bar: {
c: {
'2': 'z'
},
d: 'Hello, world!'
}
}
}
*/
deletedDiff
:const lhs = {
foo: {
bar: {
a: ['a', 'b'],
b: 2,
c: ['x', 'y'],
e: 100 // deleted
}
},
buzz: 'world'
};
const rhs = {
foo: {
bar: {
a: ['a'], // index 1 ('b') deleted
b: 2, // unchanged
c: ['x', 'y', 'z'], // 'z' added
d: 'Hello, world!' // added
}
},
buzz: 'fizz' // updated
};
console.log(deletedDiff(lhs, rhs));
/*
{
foo: {
bar: {
a: {
'1': undefined
},
e: undefined
}
}
}
*/
updatedDiff
:const lhs = {
foo: {
bar: {
a: ['a', 'b'],
b: 2,
c: ['x', 'y'],
e: 100 // deleted
}
},
buzz: 'world'
};
const rhs = {
foo: {
bar: {
a: ['a'], // index 1 ('b') deleted
b: 2, // unchanged
c: ['x', 'y', 'z'], // 'z' added
d: 'Hello, world!' // added
}
},
buzz: 'fizz' // updated
};
console.log(updatedDiff(lhs, rhs));
/*
{
buzz: 'fizz'
}
*/
detailedDiff
:const lhs = {
foo: {
bar: {
a: ['a', 'b'],
b: 2,
c: ['x', 'y'],
e: 100 // deleted
}
},
buzz: 'world'
};
const rhs = {
foo: {
bar: {
a: ['a'], // index 1 ('b') deleted
b: 2, // unchanged
c: ['x', 'y', 'z'], // 'z' added
d: 'Hello, world!' // added
}
},
buzz: 'fizz' // updated
};
console.log(detailedDiff(lhs, rhs));
/*
{
added: {
foo: {
bar: {
c: {
'2': 'z'
},
d: 'Hello, world!'
}
}
},
deleted: {
foo: {
bar: {
a: {
'1': undefined
},
e: undefined
}
}
},
updated: {
buzz: 'fizz'
}
}
*/
MIT
FAQs
Deep diffs two objects, including nested structures of arrays and objects, and return the difference.
The npm package deep-object-diff receives a total of 3,433,594 weekly downloads. As such, deep-object-diff popularity was classified as popular.
We found that deep-object-diff demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.