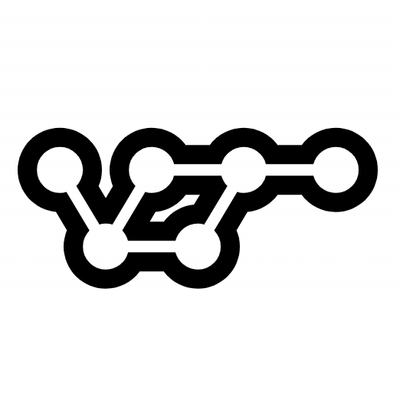
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
disable-developer-tool
Advanced tools
A simple Angular library to disable right-click context menus and prevent the F12 key press, which can be used to disable developer tools on your application for security or usability reasons.
A simple Angular library to disable right-click context menus and prevent the F12 key press, which can be used to disable developer tools on your application for security or usability reasons.
To install the disable-developer-tool
library into your Angular project, run the following command:
npm install disable-developer-tool
You can see a live demo of this library in action on StackBlitz:
To use the directives in your Angular project, follow the steps below:
typescript
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { DisableDeveloperToolModule } from 'disable-developer-tool'; // Import the module
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
DisableDeveloperToolModule // Add the module here
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Disable Right Click To disable right-click on a specific element, add the DisableRightClick directive to the element:
html
<div DisableRightClick>
This element has the right-click context menu disabled.
</div>
Disable F12 Key To disable the F12 key (usually used to open developer tools in browsers), add the DisableF12 directive to the element:
html
<div DisableF12>
This element disables the F12 key press.
</div>
You can also combine both directives on the same element:
html
<div DisableF12 DisableRightClick>
Both right-click and F12 key are disabled on this element.
</div>
This library requires the following peer dependencies to work correctly:
Version | @angular/common | @angular/core |
---|---|---|
v1.0.0 | ^12.0.5 | ^12.0.5 |
v1.1.1 | ^13.0.0 | ^13.0.0 |
v1.2.0 | ^14.0.7 | ^14.0.7 |
v1.3.0 | ^15.2.11 | ^15.2.11 |
v1.4.0 | ^16.2.16 | ^16.2.16 |
v1.5.0 | ^17.3.11 | ^17.3.11 |
v1.6.0 | ^18.2.11 | ^18.2.11 |
Ensure that these versions (or compatible versions) of Angular are installed in your project.
FAQs
A simple Angular library to disable right-click context menus and prevent the F12 key press, which can be used to disable developer tools on your application for security or usability reasons.
The npm package disable-developer-tool receives a total of 1 weekly downloads. As such, disable-developer-tool popularity was classified as not popular.
We found that disable-developer-tool demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.