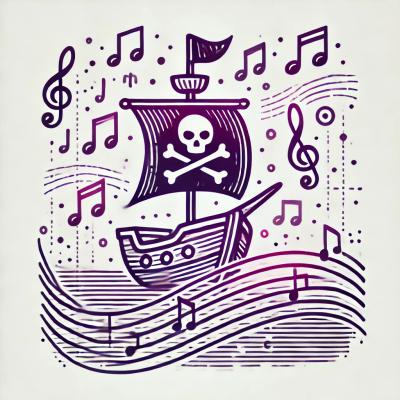
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
ember-data-updating-json-api-relationships
Advanced tools
Updating JSON:API relationships independently in Ember Data
This addon adds support to Ember Data to update relationships independently. See Updating Relationships in the JSON:API spec for more details. This library works for updating to-one and to-many relationships, as described in the JSON:API spec.
ember install ember-data-updating-json-api-relationships
First, extend the adapter:
// app/adapters/application.js
import DS from 'ember-data';
import JSONAPIAdapter from 'ember-data-updating-json-api-relationships/adapters/adapter';
export default JSONAPIAdapter.extend({
});
Second, extend the serializer:
// app/serializers/application.js
import DS from 'ember-data';
import JSONAPISerializer from 'ember-data-updating-json-api-relationships/serializers/serializer';
export default JSONAPISerializer.extend({
});
Third, add the model mixin to the model with the relationship that you want to update independently:
// app/models/article.js
import DS from 'ember-data';
import ModelMixin from 'ember-data-updating-json-api-relationships/mixins/model';
let { Model, attr, hasMany, belongsTo } = DS;
export default Model.extend(ModelMixin, {
title: attr('string'),
tags: hasMany(),
author: belongsTo()
});
Lastly, call updateRelationship(relationship)
on your model:
article.get('tags').pushObject(tag1);
article.get('tags').pushObject(tag2);
// PATCH /articles/:id/relationships/tags
article.updateRelationship('tags');
article.set('author', author);
// PATCH /articles/:id/relationships/author
article.updateRelationship('author');
This addon will set relationship URLs to what is recommended in the spec, so something like /articles/:id/relationships/tags
for a to-many relationship and /articles/:id/relationships/author
for a to-one relationship. If you need to override this, use the urlForUpdateRelationship()
hook:
// app/adapters/article.js
export default ApplicationAdapter.extend({
urlForUpdateRelationship(id, modelName, snapshot, relationshipToUpdate) {
let { host, namespace } = this;
if (relationshipToUpdate === 'tags') {
return `${host}/${namespace}/articles/${id}/categories`;
}
}
})
FAQs
Updating JSON:API relationships independently in Ember Data
The npm package ember-data-updating-json-api-relationships receives a total of 0 weekly downloads. As such, ember-data-updating-json-api-relationships popularity was classified as not popular.
We found that ember-data-updating-json-api-relationships demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.