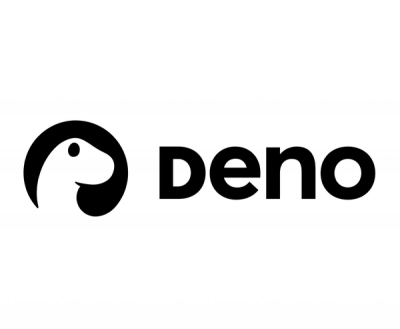
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
eslint-plugin-lodash-smells
Advanced tools
ESLint rules for lodash uses I keep an eye out for when I am doing code reviews.
Install ESLint either locally or globally.
$ npm install eslint
If you installed ESLint
globally, you have to install the lodash smells plugin globally as well. Otherwise, install it locally.
$ npm install eslint-plugin-lodash-smells
Add a plugins
section to your .eslintrc
(if it is not there already), and add lodash-smells
to the list:
{
"plugins": [
"lodash-smells"
]
}
And then enable the rules you would like to use:
{
"rules": {
"lodash-smells/no-big-ifs": 1,
"lodash-smells/no-each-push": 1,
"lodash-smells/no-get-length": 1
}
}
no-big-ifs
Intended to avoid code in this form:
_.each(myList, function(item) {
if (someCondition(item) {
doSomething(item);
}
})
Prefered:
_(myList)
.filter(someCondition)
.each(doSomething);
_.each(_.filter(myList, someCondition), doSomething);
no-each-push
Intended to avoid code in this form:
var myNewList = []
_.each(myList, function(item) {
var newItem = doSomething(item);
myNewList.push(newItem);
});
Preferred:
var myNewList = _.map(myList, doSomething);
no-get-length
Intended to avoid code in this form:
var l = _.get(myList, 'length', 0);
Preferred:
var l = _.size(myList);
FAQs
eslint rules for lodash code smells
We found that eslint-plugin-lodash-smells demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.