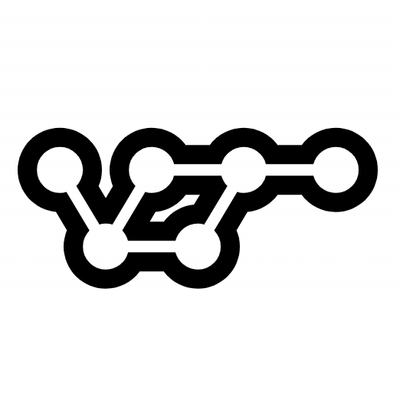
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
farm-invest-cli
Advanced tools
Are there new investment opportunities on FarmCrowdy, ThriveAgric or EFarms?
A CLI tool, built to detect updates to the products on:
with npm
npm i -g farm-invest-cli
with yarn
yarn add global farm-invest-cli
for developers
git clone https://github.com/mykeels/farm-invest-cli
cd farm-invest-cli
npm install
npm link
farm-invest-cli
farm-invest-cli agro # only agro-partnerships
farm-invest-cli efarms # only efarms
farm-invest-cli farm-crowdy # only farm-crowdy
farm-invest-cli thrive-agric # only thrive-agric
You'll get an output like:
Where the green text shows new products, and text is only shown when there is a difference between the products currently existing and the last time it checked.
const { syncAll, syncAgro, syncEFarms, syncFarmCrowdy, syncThriveAgric } = require('farm-invest-cli')
syncAll().then(diff => {
console.log(diff) // an array of (array | fast-array-diff) objects for all sources
})
syncAgro().then(diff => {
console.log(diff) // an (array | fast-array-diff) object for Agro-Partnerships
})
syncEFarms().then(diff => {
console.log(diff) // an (array | fast-array-diff) object for eFarms
})
syncFarmCrowdy().then(diff => {
console.log(diff) // an (array | fast-array-diff) object for Farm-Crowdy
})
syncThriveAgric().then(diff => {
console.log(diff) // an (array | fast-array-diff) object for Thrive-Agric
})
NB: A fast-array-diff object looks like:
{
removed:[
{ title: 'Foo', link: 'Bar' },
{ title: 'Bar', link: 'Foo' }
],
added: [ { title: 'Baz', link: 'Foo' } ]
}
const { getAgro, getEFarms, getFarmCrowdy, getThriveAgric } = require('farm-invest-cli')
getAgro().then(productList => {
console.log(productList) // an array of active products on Agro-Partnerships
})
getEFarms().then(productList => {
console.log(productList) // an array of active products on eFarms
})
getFarmCrowdy().then(productList => {
console.log(productList) // an array of active products on Farm-Crowdy
})
getThriveAgric().then(productList => {
console.log(productList) // an array of active products on Thrive-Agric
})
FAQs
Are there new investment opportunities on FarmCrowdy, ThriveAgric or EFarms?
The npm package farm-invest-cli receives a total of 2 weekly downloads. As such, farm-invest-cli popularity was classified as not popular.
We found that farm-invest-cli demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.