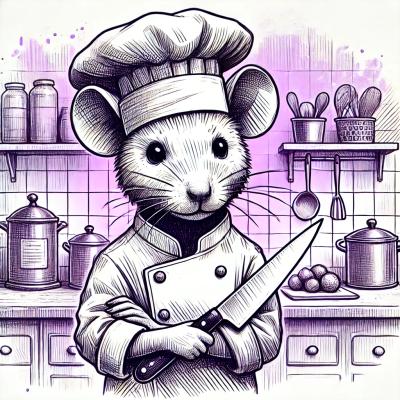
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
fixturify-project
Advanced tools
[](https://badge.fury.io/js/fixturify-project) [](https://github.com/stefanpenner/node-fixturify-project/acti
The fixturify-project npm package is a utility for creating and managing file system fixtures in a programmatic way. It is particularly useful for testing and development purposes, allowing you to easily set up and tear down file system states.
Creating a new project
This feature allows you to create a new project with a specified structure. In this example, a new project named 'my-app' is created with a single file 'index.js' containing a simple console log statement.
const { Project } = require('fixturify-project');
const project = new Project('my-app', {
files: {
'index.js': 'console.log("Hello, world!");'
}
});
project.writeSync();
Adding files to an existing project
This feature allows you to add new files to an existing project. In this example, a new file 'new-file.js' is added to the 'my-app' project with a console log statement.
const { Project } = require('fixturify-project');
const project = new Project('my-app');
project.files['new-file.js'] = 'console.log("New file");';
project.writeSync();
Reading project files
This feature allows you to read the files of an existing project. In this example, the files of the 'my-app' project are read and logged to the console.
const { Project } = require('fixturify-project');
const project = new Project('my-app');
project.readSync();
console.log(project.files);
Deleting files from a project
This feature allows you to delete files from an existing project. In this example, the 'index.js' file is deleted from the 'my-app' project.
const { Project } = require('fixturify-project');
const project = new Project('my-app');
delete project.files['index.js'];
project.writeSync();
The mock-fs package allows you to mock the file system in Node.js. It is useful for testing purposes, as it lets you create, read, and manipulate a virtual file system without affecting the real file system. Compared to fixturify-project, mock-fs focuses more on mocking the file system for testing rather than creating and managing project structures.
The memfs package provides an in-memory file system that can be used for testing and development. It allows you to create, read, and manipulate files and directories in memory. Compared to fixturify-project, memfs is more focused on providing an in-memory file system rather than managing project structures.
The fs-extra package extends the native Node.js file system module with additional methods for easier file and directory manipulation. It includes methods for copying, moving, and removing files and directories. Compared to fixturify-project, fs-extra provides more general file system utilities rather than focusing on project structure management.
When implementing JS build tooling it is common to have complete projects as fixture data. Unfortunately fixtures committed to disk can be somewhat hard to maintain and augment.
npm install --save-dev fixturify-project
const { Project } = require('fixturify-project');
const project = new Project('rsvp', '3.1.4', {
files: {
'index.js': 'module.exports = "Hello, World!"',
},
});
project.addDependency('mocha', '5.2.0');
project.addDependency('chai', '5.2.0');
project.pkg; // => the contents of package.json for the given project
project.files; // => read or write the set of files further
// if you don't set this, a new temp dir will be made for you when you write()
project.baseDir = 'some/base/dir/';
await project.write();
// after write(), you can read project.baseDir even if you didn't set it
expect(fs.existsSync(join(project.baseDir, 'index.js'))).to.eql(true);
The above example produces the following files (and most importantly the appropriate file contents:
some/base/dir/package.json
some/base/dir/index.js
some/base/dir/node_modules/mocha/package.json
some/base/dir/node_modules/chai/package.json
addDependency
returns another Project
instance, so you can nest arbitrarily deep:
const { Project } = require('fixturify-project');
let project = new Project('rsvp');
let a = project.addDependency('a');
let b = a.addDependency('b');
let c = b.addDependency('c');
await project.write();
Which produces:
$TMPDIR/xxx/package.json
$TMPDIR/xxx/index.js
$TMPDIR/xxx/node_modules/a/package.json
$TMPDIR/xxx/node_modules/a/node_modules/b/package.json
$TMPDIR/xxx/node_modules/b/node_modules/b/node_modules/c/package.json
Instead of creating all packages from scratch, you can link to real preexisting packages. This lets you take a real working package and modify it and its dependencies and watch how it behaves.
const { Project } = require('fixturify-project');
let project = new Project();
let a = project.addDependency('a');
// explicit target
project.linkDependency('b', { target: '/example/b' });
// this will follow node resolution rules to lookup "c" from "../elsewhere"
project.linkDependency('c', { baseDir: '/example' });
// this will follow node resolution rules to lookup "my-aliased-name" from "../elsewhere"
project.linkDependency('d', { baseDir: '/example', resolveName: 'my-aliased-name' });
await project.write();
Produces:
$TMPDIR/xxx/package.json
$TMPDIR/xxx/index.js
$TMPDIR/xxx/node_modules/a/package.json
$TMPDIR/xxx/node_modules/a/node_modules/b -> /example/b
$TMPDIR/xxx/node_modules/b/node_modules/c -> /example/node_modules/c
$TMPDIR/xxx/node_modules/b/node_modules/d -> /example/node_modules/my-aliased-name
When constructing a whole Project from a directory, you can choose to link all dependencies instead of copying them in as Projects:
let project = Project.fromDir('./sample-project', { linkDeps: true });
project.files['extra.js'] = '// stuff';
await project.write();
This will generate a new copy of sample-project, with symlinks to all its original dependencies, but with "extra.js" added.
By default, linkDeps
will only link up dependencies
(which is appropriate
for libraries). If you want to also include devDependencies
(which is
appropriate for apps) you can use linkDevDeps
instead.
Kind: global class
Sets the base directory of the project.
Kind: instance property of Project
Param | Description |
---|---|
dir | The directory path. |
Gets the base directory path, usually a tmp directory unless a baseDir has been explicitly set.
Kind: instance property of Project
Read only: true
string
Gets the package name from the package.json.
Kind: instance property of Project
Sets the package name in the package.json.
Kind: instance property of Project
string
Gets the version number from the package.json.
Kind: instance property of Project
Sets the version number in the package.json.
Kind: instance property of Project
Merges an object containing a directory represention with the existing files.
Kind: instance method of Project
Param | Description |
---|---|
dirJSON | An object containing a directory representation to merge. |
Writes the existing files property containing a directory representation to the tmp directory.
Kind: instance method of Project
Param | Description |
---|---|
dirJSON? | An optional object containing a directory representation to write. |
Adds a dependency to the Project's package.json.
Kind: instance method of Project
Returns:
Adds a devDependency to the Project's package.json.
Kind: instance method of Project
Returns:
Removes a dependency to the Project's package.json.
Kind: instance method of Project
Param | Description |
---|---|
name | The name of the dependency to remove. |
Removes a devDependency.
Kind: instance method of Project
Param | Description |
---|---|
name | The name of the devDependency to remove. |
Links a dependency.
Kind: instance method of Project
Param | Description |
---|---|
name | The name of the dependency to link. |
Links a devDependency.
Kind: instance method of Project
Param | Description |
---|---|
name | The name of the dependency to link. |
Kind: instance method of Project
Returns:
Kind: instance method of Project
Returns:
Kind: instance method of Project
Returns:
Disposes of the tmp directory that the Project is stored in.
Kind: instance method of Project
Reads an existing project from the specified base dir.
Kind: static method of Project
Returns:
Param | Description |
---|---|
baseDir | The base directory to read the project from. |
opts | An options object. |
opts.linkDeps | Include linking dependencies from the Project's node_modules. |
opts.linkDevDeps | Include linking devDependencies from the Project's node_modules. |
FAQs
[](https://badge.fury.io/js/fixturify-project) [](https://github.com/stefanpenner/node-fixturify-project/acti
The npm package fixturify-project receives a total of 377,601 weekly downloads. As such, fixturify-project popularity was classified as popular.
We found that fixturify-project demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.