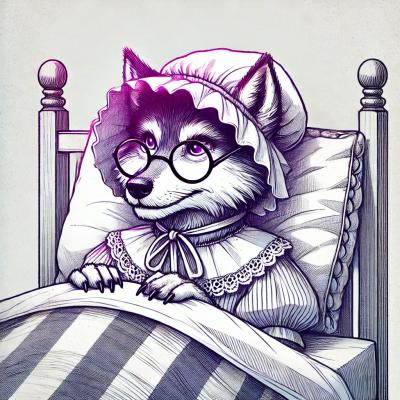
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The Flat API allows you to easily extend the abilities of the Flat Platform, with a wide range of use cases including the following:
You can find the API reference including code samples and our OpenAPI Specification at the following url: https://flat.io/developers/api/reference.
To request some API credentials, please visit https://flat.io/developers.
This JavaScript package is automatically generated by the Swagger Codegen project.
$ npm install flat-api --save
$ npm install git://github.com/FlatIO/api-client-js --save
$ bower install flat-api
The library also works in the browser environment via npm and browserify.
A build is available in the build
directory, you can also rebuild the library:
$ npm install -d && npm run build
$ ls -1 build
flat-api.js
flat-api.js.map
flat-api.min.js
Then include bundle.js in the HTML pages.
Please follow the installation instruction and execute the following JS code:
var FlatApi = require('flat-api');
// Configure OAuth2 access token for authorization
FlatApi.ApiClient.instance.authentications.OAuth2.accessToken = 'YOUR_ACCES_TOKEN';
var flatAccountApi = new FlatApi.AccountApi();
flatAccountApi.getAuthenticatedUser(function(error, data, response) {
if (error) {
console.error(error);
}
else {
console.log('Successfully retrieved user profile: ', data);
}
});
All URIs are relative to https://api.flat.io/v2
Class | Method | HTTP request | Description |
---|---|---|---|
FlatApi.AccountApi | getAuthenticatedUser | GET /me | Get current user profile |
FlatApi.ClassApi | activateClass | POST /classes/{class}/activate | Activate the class |
FlatApi.ClassApi | addClassUser | PUT /classes/{class}/users/{user} | Add a user to the class |
FlatApi.ClassApi | archiveClass | POST /classes/{class}/archive | Archive the class |
FlatApi.ClassApi | copyAssignment | POST /classes/{class}/assignments/{assignment}/copy | Copy an assignment |
FlatApi.ClassApi | createAssignment | POST /classes/{class}/assignments | Assignment creation |
FlatApi.ClassApi | createClass | POST /classes | Create a new class |
FlatApi.ClassApi | createSubmission | PUT /classes/{class}/assignments/{assignment}/submissions | Create or edit a submission |
FlatApi.ClassApi | deleteClassUser | DELETE /classes/{class}/users/{user} | Remove a user from the class |
FlatApi.ClassApi | editSubmission | PUT /classes/{class}/assignments/{assignment}/submissions/{submission} | Edit a submission |
FlatApi.ClassApi | enrollClass | POST /classes/enroll/{enrollmentCode} | Join a class |
FlatApi.ClassApi | getClass | GET /classes/{class} | Get the details of a single class |
FlatApi.ClassApi | getScoreSubmissions | GET /scores/{score}/submissions | List submissions related to the score |
FlatApi.ClassApi | getSubmission | GET /classes/{class}/assignments/{assignment}/submissions/{submission} | Get a student submission |
FlatApi.ClassApi | getSubmissions | GET /classes/{class}/assignments/{assignment}/submissions | List the students' submissions |
FlatApi.ClassApi | listAssignments | GET /classes/{class}/assignments | Assignments listing |
FlatApi.ClassApi | listClassStudentSubmissions | GET /classes/{class}/students/{user}/submissions | List the submissions for a student |
FlatApi.ClassApi | listClasses | GET /classes | List the classes available for the current user |
FlatApi.ClassApi | unarchiveClass | DELETE /classes/{class}/archive | Unarchive the class |
FlatApi.ClassApi | updateClass | PUT /classes/{class} | Update the class |
FlatApi.CollectionApi | addScoreToCollection | PUT /collections/{collection}/scores/{score} | Add a score to the collection |
FlatApi.CollectionApi | createCollection | POST /collections | Create a new collection |
FlatApi.CollectionApi | deleteCollection | DELETE /collections/{collection} | Delete the collection |
FlatApi.CollectionApi | deleteScoreFromCollection | DELETE /collections/{collection}/scores/{score} | Delete a score from the collection |
FlatApi.CollectionApi | editCollection | PUT /collections/{collection} | Update a collection's metadata |
FlatApi.CollectionApi | getCollection | GET /collections/{collection} | Get collection details |
FlatApi.CollectionApi | listCollectionScores | GET /collections/{collection}/scores | List the scores contained in a collection |
FlatApi.CollectionApi | listCollections | GET /collections | List the collections |
FlatApi.CollectionApi | untrashCollection | POST /collections/{collection}/untrash | Untrash a collection |
FlatApi.GroupApi | getGroupDetails | GET /groups/{group} | Get group information |
FlatApi.GroupApi | getGroupScores | GET /groups/{group}/scores | List group's scores |
FlatApi.GroupApi | listGroupUsers | GET /groups/{group}/users | List group's users |
FlatApi.OrganizationApi | createLtiCredentials | POST /organizations/lti/credentials | Create a new couple of LTI 1.x credentials |
FlatApi.OrganizationApi | createOrganizationInvitation | POST /organizations/invitations | Create a new invitation to join the organization |
FlatApi.OrganizationApi | createOrganizationUser | POST /organizations/users | Create a new user account |
FlatApi.OrganizationApi | listLtiCredentials | GET /organizations/lti/credentials | List LTI 1.x credentials |
FlatApi.OrganizationApi | listOrganizationInvitations | GET /organizations/invitations | List the organization invitations |
FlatApi.OrganizationApi | listOrganizationUsers | GET /organizations/users | List the organization users |
FlatApi.OrganizationApi | removeOrganizationInvitation | DELETE /organizations/invitations/{invitation} | Remove an organization invitation |
FlatApi.OrganizationApi | removeOrganizationUser | DELETE /organizations/users/{user} | Remove an account from Flat |
FlatApi.OrganizationApi | revokeLtiCredentials | DELETE /organizations/lti/credentials/{credentials} | Revoke LTI 1.x credentials |
FlatApi.OrganizationApi | updateOrganizationUser | PUT /organizations/users/{user} | Update account information |
FlatApi.ScoreApi | addScoreCollaborator | POST /scores/{score}/collaborators | Add a new collaborator |
FlatApi.ScoreApi | addScoreTrack | POST /scores/{score}/tracks | Add a new video or audio track to the score |
FlatApi.ScoreApi | createScore | POST /scores | Create a new score |
FlatApi.ScoreApi | createScoreRevision | POST /scores/{score}/revisions | Create a new revision |
FlatApi.ScoreApi | deleteScore | DELETE /scores/{score} | Delete a score |
FlatApi.ScoreApi | deleteScoreComment | DELETE /scores/{score}/comments/{comment} | Delete a comment |
FlatApi.ScoreApi | deleteScoreTrack | DELETE /scores/{score}/tracks/{track} | Remove an audio or video track linked to the score |
FlatApi.ScoreApi | editScore | PUT /scores/{score} | Edit a score's metadata |
FlatApi.ScoreApi | forkScore | POST /scores/{score}/fork | Fork a score |
FlatApi.ScoreApi | gerUserLikes | GET /users/{user}/likes | List liked scores |
FlatApi.ScoreApi | getGroupScores | GET /groups/{group}/scores | List group's scores |
FlatApi.ScoreApi | getScore | GET /scores/{score} | Get a score's metadata |
FlatApi.ScoreApi | getScoreCollaborator | GET /scores/{score}/collaborators/{collaborator} | Get a collaborator |
FlatApi.ScoreApi | getScoreCollaborators | GET /scores/{score}/collaborators | List the collaborators |
FlatApi.ScoreApi | getScoreComments | GET /scores/{score}/comments | List comments |
FlatApi.ScoreApi | getScoreRevision | GET /scores/{score}/revisions/{revision} | Get a score revision |
FlatApi.ScoreApi | getScoreRevisionData | GET /scores/{score}/revisions/{revision}/{format} | Get a score revision data |
FlatApi.ScoreApi | getScoreRevisions | GET /scores/{score}/revisions | List the revisions |
FlatApi.ScoreApi | getScoreSubmissions | GET /scores/{score}/submissions | List submissions related to the score |
FlatApi.ScoreApi | getScoreTrack | GET /scores/{score}/tracks/{track} | Retrieve the details of an audio or video track linked to a score |
FlatApi.ScoreApi | getUserScores | GET /users/{user}/scores | List user's scores |
FlatApi.ScoreApi | listScoreTracks | GET /scores/{score}/tracks | List the audio or video tracks linked to a score |
FlatApi.ScoreApi | markScoreCommentResolved | PUT /scores/{score}/comments/{comment}/resolved | Mark the comment as resolved |
FlatApi.ScoreApi | markScoreCommentUnresolved | DELETE /scores/{score}/comments/{comment}/resolved | Mark the comment as unresolved |
FlatApi.ScoreApi | postScoreComment | POST /scores/{score}/comments | Post a new comment |
FlatApi.ScoreApi | removeScoreCollaborator | DELETE /scores/{score}/collaborators/{collaborator} | Delete a collaborator |
FlatApi.ScoreApi | untrashScore | POST /scores/{score}/untrash | Untrash a score |
FlatApi.ScoreApi | updateScoreComment | PUT /scores/{score}/comments/{comment} | Update an existing comment |
FlatApi.ScoreApi | updateScoreTrack | PUT /scores/{score}/tracks/{track} | Update an audio or video track linked to a score |
FlatApi.UserApi | gerUserLikes | GET /users/{user}/likes | List liked scores |
FlatApi.UserApi | getUser | GET /users/{user} | Get a public user profile |
FlatApi.UserApi | getUserScores | GET /users/{user}/scores | List user's scores |
0.6.0 (API v2.6.0)
POST /collections
: Create new collectionGET /collections
: List collectionsGET /collections/{collection}
: Get collection detailsPUT /collections/{collection}
: Update collection detailsDELETE /collections/{collection}
: Delete collectionPOST /collections/{collection}/untrash
: Untrash collectionGET /collections/{collection}/scores
: List scores contained in a collectionPUT /collections/{collection}/scores/{score}
: Add a score to a collectionDELETE /collections/{collection}/scores/{score}
: Remove a score from a collectioncollections.readonly
: Allow read-only access to a user's collections.collections.add_scores
: Allow to add scores to a user's collections.collections
: Full, permissive scope to access all of a user's collections.POST /v2/scores/{score}/untrash
)DELETE /v2/scores/{score}
can now be used without admin rights. This new behavior will unshare the score from the current account.POST /scores/{score}/fork
now accepts a collection identifier to copy a score to a specific collection.type
(document
or inline
).ScoreRights
-> ResourceRights
ScoreCollaborator
-> ResourceCollaborator
ScoreCollaboratorCreation
-> ResourceCollaboratorCreation
ResourceSharingKey
GET /scores/{score}/revisions/{revision}/{format}
no longer support part indexes for single/set of parts exports, but our own part UUIDs.GET /users/{user}/scores
will no longer list private and shared scores, but only public scores of a Flat account.FAQs
JavaScript Client for Flat REST API (https://flat.io)
The npm package flat-api receives a total of 0 weekly downloads. As such, flat-api popularity was classified as not popular.
We found that flat-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.