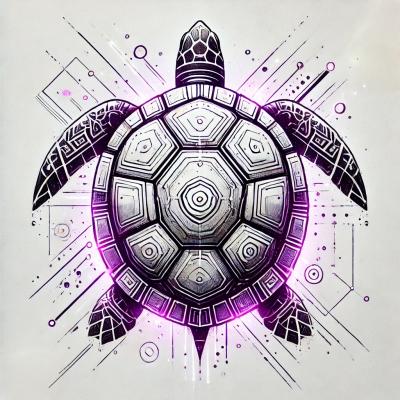
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
The gl-mat4 npm package is a utility library for performing operations on 4x4 matrices, which are commonly used in WebGL and other graphics programming contexts. It provides a variety of functions for creating, manipulating, and transforming 4x4 matrices.
Matrix Creation
This feature allows you to create a new 4x4 identity matrix. An identity matrix is a special kind of matrix that doesn't change any vector when we multiply that vector by this matrix.
const mat4 = require('gl-mat4');
const identityMatrix = mat4.create();
Matrix Multiplication
This feature allows you to multiply two 4x4 matrices. Matrix multiplication is a fundamental operation in graphics programming for combining multiple transformations.
const mat4 = require('gl-mat4');
const a = mat4.create();
const b = mat4.create();
const result = mat4.multiply(mat4.create(), a, b);
Matrix Translation
This feature allows you to apply a translation transformation to a 4x4 matrix. Translation is used to move objects in 3D space.
const mat4 = require('gl-mat4');
const out = mat4.create();
const translation = [1, 2, 3];
mat4.translate(out, out, translation);
Matrix Rotation
This feature allows you to apply a rotation transformation to a 4x4 matrix. Rotation is used to rotate objects around a specified axis.
const mat4 = require('gl-mat4');
const out = mat4.create();
const rad = Math.PI / 4;
const axis = [0, 0, 1];
mat4.rotate(out, out, rad, axis);
Matrix Scaling
This feature allows you to apply a scaling transformation to a 4x4 matrix. Scaling is used to resize objects in 3D space.
const mat4 = require('gl-mat4');
const out = mat4.create();
const scale = [2, 2, 2];
mat4.scale(out, out, scale);
gl-matrix is a high-performance matrix and vector library for WebGL. It provides similar functionalities to gl-mat4 but also includes support for other types of matrices and vectors, such as 2x2, 3x3, and 4x4 matrices, as well as 2D, 3D, and 4D vectors. It is more comprehensive and widely used in the WebGL community.
three.js is a popular 3D library that abstracts WebGL and provides a higher-level API for creating and manipulating 3D graphics. While it includes matrix and vector operations similar to gl-mat4, it also offers a full suite of tools for rendering, animation, and interaction, making it a more complete solution for 3D graphics development.
mathjs is a comprehensive math library for JavaScript that includes support for matrices and vectors, among many other mathematical operations. While it is not specifically designed for WebGL, it provides a wide range of mathematical tools that can be useful in graphics programming.
Part of a fork of @toji's
gl-matrix split into smaller pieces: this
package contains glMatrix.mat4
.
mat4 = require('gl-mat4')
Will load all of the module's functionality and expose it on a single object. Note that any of the methods may also be required directly from their files.
For example, the following are equivalent:
var scale = require('gl-mat4').scale
var scale = require('gl-mat4/scale')
Calculates the adjugate of a mat4
Creates a new mat4 initialized with values from an existing matrix
Copy the values from one mat4 to another
Creates a new identity mat4
Calculates the determinant of a mat4
Creates a matrix from a quaternion rotation.
Creates a matrix from a given angle around a given axis This is equivalent to (but much faster than):
mat4.identity(dest);
mat4.rotate(dest, dest, rad, axis);
Creates a matrix from a quaternion rotation and vector translation. This is equivalent to (but much faster than):
mat4.identity(dest);
mat4.translate(dest, vec);
var quatMat = mat4.create();
quat4.toMat4(quat, quatMat);
mat4.multiply(dest, quatMat);
Creates a matrix from a vector scaling. This is equivalent to (but much faster than):
mat4.identity(dest);
mat4.translate(dest, dest, vec);
Creates a matrix from a vector translation. This is equivalent to (but much faster than):
mat4.identity(dest);
mat4.translate(dest, dest, vec);
Creates a matrix from a vector translation This is equivalent to (but much faster than):
mat4.identity(dest);
mat4.translate(dest, dest, vec);
Creates a matrix from the given angle around the X axis This is equivalent to (but much faster than):
mat4.identity(dest)
mat4.rotateX(dest, dest, rad)
Creates a matrix from the given angle around the Y axis This is equivalent to (but much faster than):
mat4.identity(dest)
mat4.rotateY(dest, dest, rad)
Creates a matrix from the given angle around the Z axis This is equivalent to (but much faster than):
mat4.identity(dest)
mat4.rotateZ(dest, dest, rad)
Generates a frustum matrix with the given bounds
Set a mat4 to the identity matrix
Inverts a mat4
Generates a look-at matrix with the given eye position, focal point, and up axis
Multiplies two mat4's
Generates a orthogonal projection matrix with the given bounds
Generates a perspective projection matrix with the given bounds
Generates a perspective projection matrix with the given field of view.
Rotates a mat4 by the given angle
Rotates a matrix by the given angle around the X axis
Rotates a matrix by the given angle around the Y axis
Rotates a matrix by the given angle around the Z axis
Scales the mat4 by the dimensions in the given vec3
Returns a string representation of a mat4
Translate a mat4 by the given vector
Transpose the values of a mat4
zlib. See LICENSE.md for details.
FAQs
gl-matrix's mat4, split into smaller pieces
The npm package gl-mat4 receives a total of 218,755 weekly downloads. As such, gl-mat4 popularity was classified as popular.
We found that gl-mat4 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.