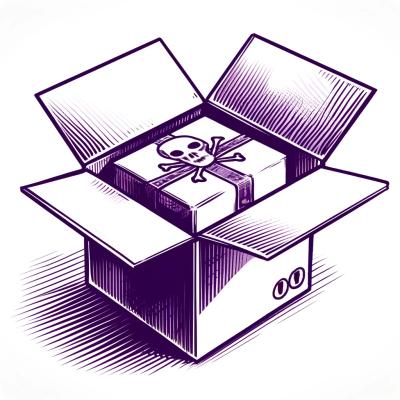
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
The hash-it npm package is a utility for creating unique hash values from JavaScript objects. It is particularly useful for creating hash keys for complex objects, ensuring that the same object will always produce the same hash value.
Hashing Objects
This feature allows you to generate a unique hash value for a given JavaScript object. The same object will always produce the same hash value, which is useful for caching or quick comparisons.
const { hash } = require('hash-it');
const obj = { a: 1, b: 2 };
const hashValue = hash(obj);
console.log(hashValue);
Hashing Arrays
This feature allows you to generate a unique hash value for an array. It ensures that the same array will always produce the same hash value, which can be useful for array comparisons or caching.
const { hash } = require('hash-it');
const arr = [1, 2, 3];
const hashValue = hash(arr);
console.log(hashValue);
Hashing Nested Structures
This feature allows you to generate a unique hash value for nested structures, including objects within objects and arrays within objects. It ensures consistency in hash values for complex data structures.
const { hash } = require('hash-it');
const nestedObj = { a: { b: 2 }, c: [1, 2, 3] };
const hashValue = hash(nestedObj);
console.log(hashValue);
The object-hash package provides similar functionality to hash-it by generating unique hash values for JavaScript objects. It supports a variety of hashing algorithms and can handle complex nested structures. Compared to hash-it, object-hash offers more customization options for the hashing process.
The hasha package is a versatile hashing utility that supports multiple hashing algorithms like MD5, SHA-1, and SHA-256. While it is not specifically designed for hashing objects, it can be used to hash strings, buffers, and other data types. It is more general-purpose compared to hash-it.
The built-in Node.js crypto module provides a wide range of cryptographic functionalities, including hashing. While it is more complex and requires more setup to hash objects compared to hash-it, it offers extensive options for cryptographic operations and is highly customizable.
Fast and consistent hashCode for any object type
// ES2015
import hash from "hash-it";
// CommonJS
const hash = require("hash-it").default;
// script
const hash = window.hashIt;
// hash any standard object
console.log(hash({ foo: "bar" })); // 8999940026732
// or a circular object
console.log(hash(window)); // 6514964902729
hash-it
has a simple goal: provide a fast, consistent, unique hashCode for any object type that is uniquely based on its values. This has a number of uses such as duplication prevention, equality comparisons, blockchain construction, etc.
Any object type?
Yes, any object type. Primitives, ES2015 classes like Symbol
, DOM elements (yes, you can even hash the window
object if you want). Any object type.
With no exceptions?
Well ... sadly, no, there are a few exceptions.
Promise
Generator
(the result of calling a GeneratorFunction
)
Promise
, there is no way to obtain the values contained within due to its dynamic iterable natureWeakMap
/ WeakSet
In each of these cases, no matter what the values of the object, they will always yield the same hash result, which is unique to each object type. If you have any ideas about how these can be uniquely hashed, I welcome them!
Here is the list of object classes that have been tested and shown to produce unique hashCodes:
Arguments
Array
ArrayBuffer
AsyncFunction
(based on toString
)Boolean
DataView
(based on its buffer
)Date
(based on getTime
)DocumentFragment
(based on outerHTML
of all children
)Error
(based on stack
)
TypeError
, ReferenceError
, etc.)Event
(based on all properties other than Event.timeStamp
)
MouseEvent
, KeyboardEvent
, etc.)Float32Array
Float64Array
Function
(based on toString
)GeneratorFunction
(based on toString
)Int8Array
Int16Array
Int32Array
HTMLElement
(based on outerHTML
)
HTMLAnchorElement
, HTMLDivElement
, etc.)Map
(order-agnostic)Null
Number
Object
(handles circular objects, order-agnostic)Proxy
RegExp
Set
(order-agnostic)String
SVGElement
(based on outerHTML
)
SVGRectElement
, SVGPolygonElement
, etc.)Symbol
(based on toString
)Uint8Array
Uint8ClampedArray
Uint16Array
Uint32Array
Undefined
Window
This is basically all I could think of, but if I have missed an object class let me know and I will add it!
is(object: any, otherObject: any): boolean
Compares the two objects to determine equality.
console.log(hash.is(null, 123)); // false
console.log(hash.is(null, null)); // true
NOTE: This can also be used with partial-application to create prepared equality comparators.
const isNull = hash.is(null);
console.log(isNull(123)); // false
console.log(isNull(null)); // true
is.all(object1: any, object2: any[, object3: any[, ...objectN]]): boolean
Compares the first object to all other objects passed to determine if all are equal based on hashCode
const foo = {
foo: "bar"
};
const alsoFoo = {
foo: "bar"
};
const stillFoo = {
foo: "bar"
};
console.log(hash.is.all(foo, alsoFoo)); // true
console.log(hash.is.all(foo, alsoFoo, stillFoo)); // true
NOTE: This can also be used with partial-application to create prepared equality comparators.
const foo = {
foo: "bar"
};
const alsoFoo = {
foo: "bar"
};
const stillFoo = {
foo: "bar"
};
const isAllFoo = hash.is.all(foo);
console.log(isAllFoo(alsoFoo, stillFoo)); // true
is.any(object1: any, object2: any[, object3: any[, ...objectN]]): boolean
Compares the first object to all other objects passed to determine if any are equal based on hashCode
const foo = {
foo: "bar"
};
const alsoFoo = {
foo: "bar"
};
const nopeBar = {
bar: "baz"
};
console.log(hash.is.any(foo, alsoFoo)); // true
console.log(hash.is.any(foo, nopeBar)); // false
console.log(hash.is.any(foo, alsoFoo, nopeBar)); // true
NOTE: This can also be used with partial-application to create prepared equality comparators.
const foo = {
foo: "bar"
};
const alsoFoo = {
foo: "bar"
};
const nopeBar = {
bar: "baz"
};
const isAnyFoo = hash.is.any(foo);
console.log(isAnyFoo(alsoFoo, nopeBar)); // true
is.not(object: any, otherObject: any): boolean
Compares the two objects to determine non-equality.
console.log(hash.is.not(null, 123)); // true
console.log(hash.is.not(null, null)); // false
NOTE: This can also be used with partial-application to create prepared equality comparators.
const isNotNull = hash.is.not(null);
console.log(isNotNull(123)); // true
console.log(isNotNull(null)); // flse
While the hashes will be consistent when calculated within the same browser environment, there is no guarantee that the hashCode will be the same across different browsers due to browser-specific implementations of features. A vast majority of the time things line up, but there are some edge cases that can cause differences, so just something to be mindful of.
Standard stuff, clone the repo and npm install
dependencies. The npm scripts available:
build
=> run webpack to build development dist
file with NODE_ENV=developmentbuild:minified
=> run webpack to build production dist
file with NODE_ENV=productiondev
=> run webpack dev server to run example app / playgrounddist
=> runs build
and build:minified
lint
=> run ESLint against all files in the src
folderprepublish
=> runs prepublish:compile
when publishingprepublish:compile
=> run lint
, test:coverage
, transpile:es
, transpile:lib
, dist
test
=> run AVA test functions with NODE_ENV=test
test:coverage
=> run test
but with nyc
for coverage checkertest:watch
=> run test
, but with persistent watchertranspile:lib
=> run babel against all files in src
to create files in lib
transpile:es
=> run babel against all files in src
to create files in es
, preserving ES2015 modules (for
pkg.module
)4.1.0
FAQs
Hash any object based on its value
The npm package hash-it receives a total of 248,181 weekly downloads. As such, hash-it popularity was classified as popular.
We found that hash-it demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.