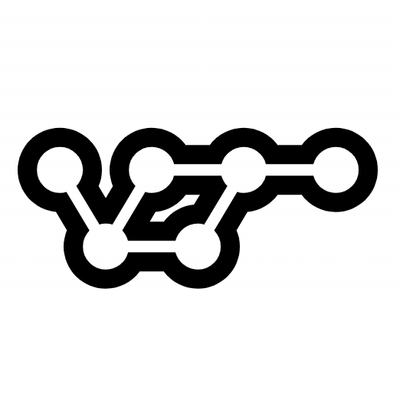
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
jalali-calendar-package
Advanced tools
A customizable and interactive JavaScript calendar built with support for the Persian (Jalali) calendar, powered by moment-jalaali. This library offers rich functionality for date selection, custom event handling, and easy UI interaction with Persian localization.
Note: still under development. use versions 1.1.1 and later.
This guide provides step-by-step instructions on how to integrate and use the Jalali Calendar in your web projects, supporting frameworks like Angular, React, or plain JavaScript.
Install the package via npm:
npm install jalali-calendar-package
You also need to install moment-jalaali if it’s not included:
npm install moment-jalaali
In a vanilla JavaScript project:
HTML
<div id="calendar-container"></div>
JavaScript
import Calendar from 'jalali-calendar-package';
const calendar = new Calendar('#calendar-container');
calendar.initializeCalendar();
Install the package
npm install jalali-calendar-package
Create a New Component
ng generate component jalali-calendar
Import and Use the Calendar in the Component
In your jalali-calendar.component.ts file:
import { Component, AfterViewInit } from '@angular/core';
import Calendar from 'jalali-calendar-package';
@Component({
selector: 'app-jalali-calendar',
templateUrl: './jalali-calendar.component.html',
styleUrls: ['./jalali-calendar.component.scss']
})
export class JalaliCalendarComponent implements AfterViewInit {
ngAfterViewInit() {
const calendar = new Calendar('#calendar-container');
calendar.initializeCalendar();
}
}
In your jalali-calendar.component.html file:
<div id="calendar-container"></div>
Install the package
npm install jalali-calendar-package
Use Calendar in a React Component
In your CalendarComponent.js file:
import React, { useEffect } from 'react';
import Calendar from 'jalali-calendar-package';
const CalendarComponent = () => {
useEffect(() => {
const calendar = new Calendar('#calendar-container');
calendar.initializeCalendar();
}, []);
return <div id="calendar-container"></div>;
}
export default CalendarComponent;
You can customize the calendar’s appearance using the guide.css file, which contains modifiable classes.
Customizing CSS
Refer to dist/guide.css for styling guidelines.
Override styles by adding your custom CSS:
/* Custom CSS for Calendar */
.calendar-container {
background-color: #fafafa; /* Change calendar background */
}
.jc-calendar-header {
background-color: #ffeb3b; /* Customize header background */
color: #000; /* Customize header text color */
}
.jc-calendar-day:hover {
background-color: #d32f2f; /* Custom hover background */
}
.jc-calendar-header button {
background-color: #388e3c; /* Change button color */
color: white; /* Button text color */
}
Include guide.css after the default calendar styles:
<link rel="stylesheet" href="path/to/calendar.css">
<link rel="stylesheet" href="path/to/guide.css"> <!-- Your custom styles -->
Calendar Initialization
initializeCalendar()
Initializes the calendar, sets up the UI, and adds default event listeners.
calendar.initializeCalendar();
Navigation and Date Management
goToToday()
Navigates the calendar to the current date.
calendar.goToToday();
navigateTo(date)
Navigates the calendar to a specified date. The date should be in the ISO string format.
calendar.navigateTo('2024-11-06');
Event Handling
The calendar allows you to define custom actions for various mouse events. Events can be assigned using addMouseActions or individually with specific methods for each event type.
addMouseActions({ onClick, onRightClick, onHover })
Assigns custom functions for click, right-click, and hover actions on day elements.
calendar.addMouseActions({
onClick: (dayElement) => {
console.log(`Clicked: ${dayElement.dataset.date}`);
},
onRightClick: (dayElement) => {
console.log(`Right-clicked: ${dayElement.dataset.date}`);
},
onHover: (dayElement) => {
dayElement.style.backgroundColor = '#e0e0e0';
}
});
handleDayClick(dayElement)
Handles a click event on a specific day element.
calendar.handleDayClick(dayElement);
handleRightClick(dayElement)
Handles a right-click event on a specific day element.
calendar.handleRightClick(dayElement);
handleHover(dayElement)
Handles a hover event on a specific day element.
calendar.handleHover(dayElement);
Selection Management
clearSelection()
Clears all selected days from the calendar.
calendar.clearSelection();
Rendering and UI Updates
renderDays()
Renders the day elements for the current month, highlighting the current day, selected days, and days from adjacent months.
calendar.renderDays();
markSelectedDays(selectedDays)
Marks specified days as selected within the calendar.
selectedDays (Array): An array of dates in Jalali format to be highlighted as selected.
calendar.markSelectedDays(['1402-08-15', '1402-08-16']);
Month and Year Selection
createMonthSelector()
Generates a modal for selecting months within the current year.
calendar.createMonthSelector();
createYearSelector()
Generates a modal for selecting years within a customizable range.
calendar.createYearSelector();
UI Container Management
getContainer(selector)
Retrieves a container element within the calendar UI by a specific selector.
selector (String): CSS selector to identify the container element.
const header = calendar.getContainer('.jc-calendar-header');
clearContainer(selector)
Clears the content of a specified container element within the calendar.
calendar.clearContainer('.jc-calendar-footer');
appendToContainer(selector, element)
Appends an HTML element to a specified container within the calendar.
selector (String): CSS selector to identify the container element.
element (HTMLElement): The element to append.
calendar.appendToContainer('.jc-calendar-days', newDayElement);
Utility Methods
formatDate(date)
Formats a date according to the Jalali calendar standard used within the package.
date (Date | String): The date to format, either as a Date object or ISO string.
const formattedDate = calendar.formatDate('2024-11-06');
console.log(formattedDate); // Example output: 1402-08-16
parseJalali(date)
Parses a Jalali date string and returns a moment object for further manipulation.
date (String): A Jalali date string.
const jalaliMoment = calendar.parseJalali('1402-08-15');
Configuration Options
The calendar supports a range of customizable options to adapt to your specific needs. These options can be passed when initializing the calendar instance.
Example:
const calendar = new CalendarClass('#my-calendar', {
initialDate: '2024-11-06', // Set the starting date
selectedDays: ['1402-08-15'], // Preselect days
weekdays: ['ش', 'ی', 'د', 'س', 'چ', 'پ', 'ج'], // Custom weekdays
months: ['فروردین', 'اردیبهشت', 'خرداد', 'تیر', 'مرداد', 'شهریور', 'مهر', 'آبان', 'آذر', 'دی', 'بهمن', 'اسفند'], // Custom month names
});
Q: Can I use this calendar with a different localization?
Yes, the package uses the moment-jalaali library, which supports Persian (Farsi) and other localizations.
Q: How do I add additional event handlers?
You can easily customize the calendar by adding your own mouse or keyboard event listeners using the addMouseActions method.
FAQs
contact me: parisamahmudigh@gmail.com
The npm package jalali-calendar-package receives a total of 0 weekly downloads. As such, jalali-calendar-package popularity was classified as not popular.
We found that jalali-calendar-package demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.