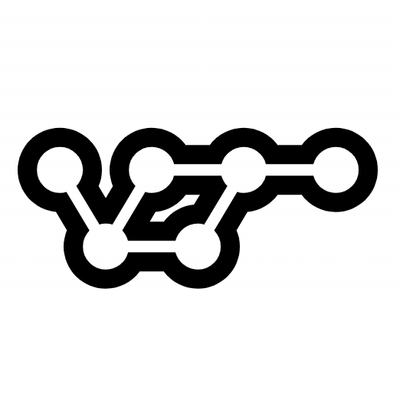
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
jquery-disable-scroll-plugin
Advanced tools
Disable scroll bar scrolling effect, compatible with other custom scroll bar plugins
This plugin allows you to disable page scrolling without hiding the scrollbars.
You need to have jQuery installed. Version 1.7.0 or higher is required.
npm install jquery
npm install jquery-disable-scroll-plugin
<script src="path/to/node_modules/jquery/dist/jquery.min.js"></script>
<script src="path/to/node_modules/jquery-disable-scroll-plugin/jquery.disable-scroll.min.js"></script>
<script src="https://unpkg.com/jquery@latest/dist/jquery.min.js"></script>
<script src="https://unpkg.com/jquery-disable-scroll-plugin@latest/jquery.disable-scroll.min.js"></script>
<script src="https://unpkg.zhimg.com/jquery@latest/dist/jquery.min.js"></script>
<script src="https://unpkg.zhimg.com/jquery-disable-scroll-plugin@latest/jquery.disable-scroll.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/jquery@latest/dist/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/jquery-disable-scroll-plugin@latest/jquery.disable-scroll.min.js"></script>
https://github.com/gxlydlyf/jquery-disable-scroll-plugin/releases/latest
<script src="path/to/jquery.min.js"></script>
<script src="path/to/jquery.disable-scroll.min.js"></script>
For parameter details, refer to here.
var $element = $('#element'); // Element to control
// Enable scrolling, choose one of the following methods
$element.EnableScroll();
$element.EnableScroll("xy");
$element.EnableScroll("all");
$element.ControlScroll(true);
$element.ControlScroll(true, "xy");
$element.ControlScroll(true, "all");
// Disable scrolling, choose one of the following methods
$element.DisableScroll();
$element.DisableScroll("xy");
$element.DisableScroll("all");
$element.ControlScroll(false);
$element.ControlScroll(false, "xy");
$element.ControlScroll(false, "all");
var direction;
direction = "x"; // When setting x
direction = "y"; // When setting y
// Enable scrolling, choose one of the following methods
$element.EnableScroll(direction);
$element.ControlScroll(true, direction);
// Disable scrolling, choose one of the following methods
$element.DisableScroll(direction);
$element.ControlScroll(false, direction);
Scrolling will be enabled when all IDs are removed.
$element.ControlScroll(false, "x", "id_name"); // Disable x-axis with ID "id_name"
// Disable x-axis with IDs 'id1' and 'id2', disable y-axis with ID 'id1'
$element.DisableScroll({
x: ['id1', 'id2'],
y: 'id1'
});
// Equivalent to
$element.ControlScroll(false, {
x: ['id1', 'id2'],
y: 'id1'
});
// Disable both axes with IDs 'id1' and 'id2'
$element.DisableScroll("xy", ['id1', 'id2']);
$element.disableScrollId(); // Returns an object
{
"x": {
"id1": true,
"id2": true
},
"y": {
"id1": true,
"id": true
}
}
$('#div').ControlScroll(
{
status: false,
direction: 'x',
id: 'id'
}
); // Disable x-axis with ID 'id'
$('#div').DisableScroll(
{
status: false,
direction: 'y',
id: 'id'
}
); // Disable y-axis with ID 'id'
$('#div').DisableScroll(
{
status: true,
direction: 'y',
id: 'id'
}
); // Enable y-axis, remove ID 'id'
$element.disableScrollStatus(); // Returns an object
False indicates disabled status, true indicates enabled status.
{
"x": false,
"y": true
}
jQuery.DisableScroll.defaults
{
"direction": "xy",
"id": "default",
"status": "disable"
}
jQuery.DisableScroll.direction = "x";
jQuery.DisableScroll.id = "id";
jQuery.DisableScroll.status = "enable"; // Applicable to ControlScroll, can also be a boolean value, true for default enabled, false for default disabled
The noConflict
function is used to handle conflicts with plugin names, ensuring that the plugin can function properly in different environments.
type
(String): Optional parameter specifying the handling type, with possible values 'fn', 'fn-ext', 'fn-default', 'method'. If not provided or out of range, defaults to 'method'.plugin
object.disable
, control
, enable
methods.false
.jQuery.NewName = jQuery.DisableScroll.noConflict('method');
// jQuery.NewName will now be equivalent to the original jQuery.DisableScroll
Function names include
{
"fn": [
"controlScroll",
"ctrlScroll",
"disableScroll",
"offScroll",
"enableScroll",
"onScroll",
"ControlScroll",
"Control_scroll",
"control_scroll",
"controlscroll",
"CtrlScroll",
"ctrl_scroll",
"ctrlscroll",
"DisableScroll",
"Disable_scroll",
"disable_scroll",
"disablescroll",
"OffScroll",
"off_scroll",
"offscroll",
"enableScroll",
"enable_scroll",
"enable_scroll",
"enablescroll",
"OnScroll",
"on_scroll",
"onscroll"
]
}
var names = jQuery.DisableScroll.noConflict('fn');
// Assign
jQuery.fn.newControl = names.control;
jQuery.fn.newDisable = names.disable;
jQuery.fn.newEnable = names.enable;
// Usage
$element.newControl(); // Equivalent to $element.ControlScroll();
$element.newDisable(); // Equivalent to $element.DisableScroll();
$element.newEnable(); // Equivalent to $element.EnableScroll();
Similar to handling type 'fn', Function names include
{
"fn-ext": [
"ControlScroll",
"Control_scroll",
"control_scroll",
"controlscroll",
"CtrlScroll",
"ctrl_scroll",
"ctrlscroll",
"DisableScroll",
"Disable_scroll",
"disable_scroll",
"disablescroll",
"OffScroll",
"off_scroll",
"offscroll",
"enableScroll",
"enable_scroll",
"enable_scroll",
"enablescroll",
"OnScroll",
"on_scroll",
"onscroll"
]
}
Similar to handling type 'fn', Function names include
{
"fn-default": [
"controlScroll",
"ctrlScroll",
"disableScroll",
"offScroll",
"enableScroll",
"onScroll"
]
}
Original Name | Aliases |
---|---|
ControlScroll | ctrlScrool CtrlScroll ctrlscroll ctrl_scroll Ctrl_scroll controlScroll control_scroll Control_scroll ControlScroll controlscroll |
DisableScroll | offScrool OffScroll offscroll off_scroll Off_scroll disableScroll disable_scroll Disable_scroll DisableScroll disablescroll |
EnableScroll | onScroll OnScrolll onscrolll on_scrolll On_scrolll enableScrolll enable_scrolll Enable_scrolll EnableScrolll enablescroll |
After downloading the project, open the index.html file to see the effect or View it online here
The plugin is theoretically compatible with all browsers.
Feel free to raise any suggestions or issues on GitHub Issues
FAQs
Disable scroll bar scrolling effect, compatible with other custom scroll bar plugins
The npm package jquery-disable-scroll-plugin receives a total of 2 weekly downloads. As such, jquery-disable-scroll-plugin popularity was classified as not popular.
We found that jquery-disable-scroll-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.