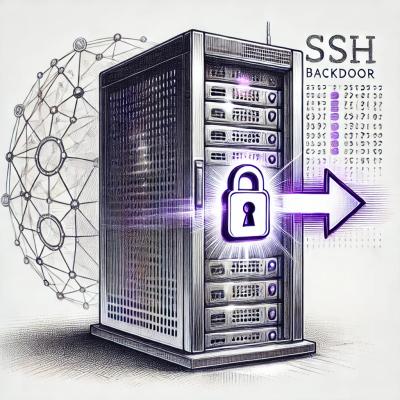
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
A public-key signature system based on Ed25519 for the NATS ecosystem in javascript
The nkeys.js package is a JavaScript library for handling NATS keys. It provides functionalities for creating, managing, and verifying NATS keys, which are used for secure communication in NATS (a high-performance messaging system).
Generate a new NATS key pair
This feature allows you to generate a new NATS key pair, which includes a public key and a private key. The public key can be shared, while the private key should be kept secure.
const nkeys = require('nkeys.js');
const kp = nkeys.createAccount();
console.log('Public Key:', kp.getPublicKey());
console.log('Private Key:', kp.getPrivateKey());
Sign a message
This feature allows you to sign a message using the private key of a NATS key pair. The signature can be used to verify the authenticity of the message.
const nkeys = require('nkeys.js');
const kp = nkeys.createAccount();
const message = Buffer.from('Hello, NATS!');
const signature = kp.sign(message);
console.log('Signature:', signature.toString('hex'));
Verify a message signature
This feature allows you to verify the signature of a message using the public key of a NATS key pair. It ensures that the message was signed by the corresponding private key.
const nkeys = require('nkeys.js');
const kp = nkeys.createAccount();
const message = Buffer.from('Hello, NATS!');
const signature = kp.sign(message);
const publicKey = kp.getPublicKey();
const isValid = nkeys.fromPublic(publicKey).verify(message, signature);
console.log('Is the signature valid?', isValid);
TweetNaCl is a cryptographic library that provides similar functionalities for key generation, signing, and verification. It is a port of the Networking and Cryptography library (NaCl) to JavaScript. Compared to nkeys.js, TweetNaCl is more general-purpose and not specifically tailored for NATS.
Node-forge is a comprehensive cryptographic library for Node.js that includes support for key generation, signing, and verification, among other cryptographic operations. It is more feature-rich and versatile compared to nkeys.js, which is specifically designed for NATS key management.
The built-in 'crypto' module in Node.js provides cryptographic functionalities, including key generation, signing, and verification. While it is not as specialized as nkeys.js for NATS, it is a powerful and widely-used library for general cryptographic operations in Node.js applications.
A public-key signature system based on Ed25519 for the NATS ecosystem system for JavaScript.
The nkeys.js library works in Deno, Node.js, and the browser!
The nkeys library is available on both npm and jsr.io
In Deno:
deno add @nats-io/nkeys
import { createUser, fromPublic, fromSeed } from "@nats-io/nkeys";
In Node:
npm install nkeys.js
const { createUser, fromSeed, fromPublic } = require("nkeys.js");
// or
import { createUser, fromPublic, fromSeed } from "nkeys.js";
On your browser projects copy node_modules/nkeys.js/nkeys.mjs
to your document
root, and then
import { createUser, fromPublic, fromSeed } from "https://host/path/nkeys.mjs";
// create an user nkey KeyPair (can also create accounts, operators, etc).
const user = createUser();
// A seed is the public and private keys together.
const seed: Uint8Array = user.getSeed();
// Seeds are encoded into Uint8Array, and start with
// the letter 'S'. Seeds need to be kept safe and never shared
console.log(`seeds start with s: ${seed[0] === "S".charCodeAt(0)}`);
// A seed's second letter encodes it's type:
// `U` for user,
// `A` for account,
// `O` for operators
console.log(`nkey is for a user? ${seed[1] === "U".charCodeAt(0)}`);
// To view a seed, simply decode it:
console.log(new TextDecoder().decode(seed));
// you can recreate the keypair with its seed:
const priv = fromSeed(seed);
// Using the KeyPair, you can cryptographically sign content:
const data = new TextEncoder().encode("Hello World!");
const sig = priv.sign(data);
// and verify a signature:
const valid = user.verify(data, sig);
if (!valid) {
console.error("couldn't validate the data/signature against my key");
} else {
console.error("data was verified by my key");
}
// others can validate using your public key:
const publicKey = user.getPublicKey();
const pub = fromPublic(publicKey);
if (!pub.verify(data, sig)) {
console.error(`couldn't validate the data/signature with ${publicKey}`);
} else {
console.info(`data was verified by ${publicKey}`);
}
// when extracting with seeds or private keys
// you should clear them when done:
seed.fill(0);
// you should also clear the keypairs:
user.clear();
priv.clear();
Our support policy for Nodejs versions follows Nodejs release support. We will support and build nkeys.js on even-numbered Nodejs versions that are current or in LTS.
Note that this library no longer shims atob
, btoa
, TextEncoder
, nor
TextDecoder
. These should be available in fairly old node builds going as far
back as Node 16. If you need to run on an older environment, use one of the
older versions on npm.
Unless otherwise noted, the NATS source files are distributed under the Apache Version 2.0 license found in the LICENSE file.
FAQs
A public-key signature system based on Ed25519 for the NATS ecosystem in javascript
We found that nkeys.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.