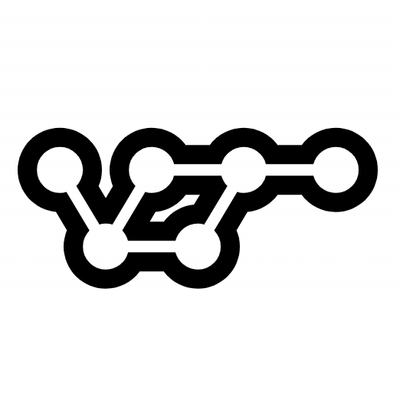
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
node-r3trans
Advanced tools
NodeJs wrapper for SAP R3trans.
npm install node-r3trans
Start by testing if the R3trans program is installed correctly and print out its version.
import { R3trans } from "node-r3trans";
const r3trans = new R3trans({
r3transDirPath: "", //Optional, can be used instead of the R3TRANS_HOME enviroment variable
tempDirPath: "", //Optional, the R3trans program will generate temporary files, and this folder indicates where they shall be generated. If left blank, defaults to the R3trans program dir path
});
r3trans.getVersion().then(version => {
console.log(version);
}).catch(err => {
console.error(err);
});
A transport data file can always be passed to the instance object methods as a buffer or a string file path.
r3trans.isTransportValid(buffer).then(valid => {
console.log("valid", valid);
}).catch(err => {
console.error(err);
});
r3trans.getLogBuffer(buffer, 2).then(log => {
console.log(log.toString());
}).catch(err => {
console.error(err);
});
r3trans.getTransportTrkorr(buffer).then(trkorr => {
console.log("trkorr", trkorr);
}).catch(err => {
console.error(err);
});
r3trans.getTableEntries(buffer, "TADIR").then(tadir => {
console.log("tadir", tadir);
}).catch(err => {
console.error(err);
});
FAQs
NodeJs wrapper for SAP R3trans program
The npm package node-r3trans receives a total of 72 weekly downloads. As such, node-r3trans popularity was classified as not popular.
We found that node-r3trans demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.