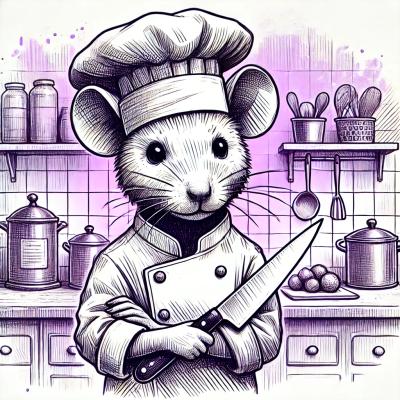
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
ordered-read-streams
Advanced tools
Combines array of streams into one Readable stream in strict order.
The ordered-read-streams npm package allows you to combine multiple readable streams into a single readable stream, ensuring that the data from each stream is read in the order the streams were provided. This can be particularly useful when you need to process multiple streams sequentially.
Combining Multiple Streams
This feature allows you to combine multiple readable streams into a single stream. The data from each stream is read in the order the streams were provided. In this example, the contents of 'file1.txt', 'file2.txt', and 'file3.txt' are read sequentially and output to the console.
const OrderedReadStreams = require('ordered-read-streams');
const fs = require('fs');
const stream1 = fs.createReadStream('file1.txt');
const stream2 = fs.createReadStream('file2.txt');
const stream3 = fs.createReadStream('file3.txt');
const combinedStream = new OrderedReadStreams([stream1, stream2, stream3]);
combinedStream.on('data', (chunk) => {
console.log(chunk.toString());
});
combinedStream.on('end', () => {
console.log('All streams have been read in order.');
});
The multistream package also allows you to combine multiple streams into a single stream. However, unlike ordered-read-streams, multistream provides more flexibility by allowing you to dynamically add streams during the reading process. This can be useful if the streams to be combined are not known upfront.
The merge-stream package allows you to merge multiple streams into one. Unlike ordered-read-streams, merge-stream does not guarantee the order of the streams. It is useful when you need to process multiple streams concurrently and do not require the data to be in a specific order.
The stream-combiner2 package allows you to combine multiple streams into a pipeline. While it does not specifically focus on maintaining the order of streams, it is useful for creating complex stream processing pipelines where the output of one stream is the input to another.
Combines array of streams into one Readable stream in strict order.
var { Readable } = require('streamx');
var ordered = require('ordered-read-streams');
var s1 = new Readable({
read: function (cb) {
var self = this;
if (self.called) {
self.push(null);
return cb(null);
}
setTimeout(function () {
self.called = true;
self.push('stream 1');
cb(null);
}, 200);
},
});
var s2 = new Readable({
read: function (cb) {
var self = this;
if (self.called) {
self.push(null);
return cb(null);
}
setTimeout(function () {
self.called = true;
self.push('stream 2');
cb(null);
}, 30);
},
});
var s3 = new Readable({
read: function (cb) {
var self = this;
if (self.called) {
self.push(null);
return cb(null);
}
setTimeout(function () {
self.called = true;
self.push('stream 3');
cb(null);
}, 100);
},
});
var readable = ordered([s1, s2, s3]);
readable.on('data', function (data) {
console.log(data);
// Logs:
// stream 1
// stream 2
// stream 3
});
ordered(streams, [options])
Takes an array of Readable
streams and produces a single OrderedReadable
stream that will consume the provided streams in strict order. The produced Readable
stream respects backpressure on itself and any provided streams.
orderedReadable.addSource(stream)
The returned Readable
stream has an addSource
instance function that takes appends a Readable
stream to the list of source streams that the OrderedReadable
is reading from.
MIT
FAQs
Combines array of streams into one Readable stream in strict order.
We found that ordered-read-streams demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.