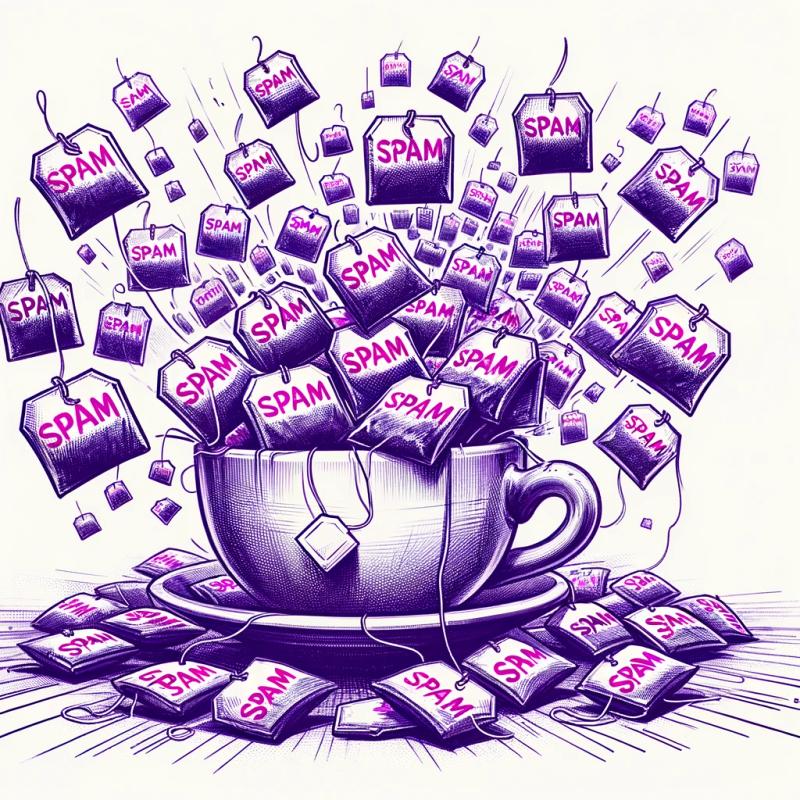
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
p-debounce
Advanced tools
Readme
Debounce promise-returning & async functions
$ npm install p-debounce
import pDebounce from 'p-debounce';
const expensiveCall = async input => input;
const debouncedFn = pDebounce(expensiveCall, 200);
for (const number of [1, 2, 3]) {
console.log(await debouncedFn(number));
}
//=> 3
//=> 3
//=> 3
Returns a function that delays calling fn
until after wait
milliseconds have elapsed since the last time it was called.
Type: Function
Promise-returning/async function to debounce.
Type: number
Milliseconds to wait before calling fn
.
Type: object
Type: boolean
Default: false
Call the fn
on the leading edge of the timeout. Meaning immediately, instead of waiting for wait
milliseconds.
Execute function_
unless a previous call is still pending, in which case, return the pending promise. Useful, for example, to avoid processing extra button clicks if the previous one is not complete.
Type: Function
Promise-returning/async function to debounce.
import {setTimeout as delay} from 'timers/promises';
import pDebounce from 'p-debounce';
const expensiveCall = async value => {
await delay(200);
return value;
}
const debouncedFn = pDebounce.promise(expensiveCall);
for (const number of [1, 2, 3]) {
console.log(await debouncedFn(number));
}
//=> 1
//=> 2
//=> 3
FAQs
Debounce promise-returning & async functions
The npm package p-debounce receives a total of 71,668 weekly downloads. As such, p-debounce popularity was classified as popular.
We found that p-debounce demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.